THIS NEEDS TO BE DONE IN JAVA Modify the Account class to provide a debit method that withdraws money from an Account. Ensure that the debit amount does not exceed the Account's balance. If it does, the balance should be left unchanged and the method should print out a message indicating "Debit amount exceeded amount balance." Modify class AccountTest to test method debit. Below are links to the Account and AccountTest programs. //This is for the account class public class Account { private String name; //instance variable private double balance; //instance variable //Account constructor that receives two parameters public Account(String name, double balance){ this.name = name; //assing name to instance varialbe name //validate that the balance is greater than 0.0; if it's not, //instance varisble balance keeps its default initial value of 0.0 if (balance > 0.0){ //if the balance is valaid this.balance = balance; //assign it to instance variable balance } } //method that deposits (adds) only a valid amount to the balance public void deposit (double depositAmount){ if (depositAmount > 0.0) { balance = balance + depositAmount; //add it to the balance } } //method returns the account balance public double getBalance(){ return balance; } //method that sets the name public void setName(String name){ this.name = name; } //method that returns the name public String getName(){ return name; } } //This is for the account test public class AccountTest { /** * @param args the command line arguments */ public static void main(String[] args) { //create two Account objects Account account1 = new Account("Jane Green", 50.00); Account account2 = new Account("John Blue", -7.53); //display initial balance of each object System.out.printf("%s balance: $%.2f%n", account1.getName(), account1.getBalance()); System.out.printf("%s balance: $%.2f%n", account2.getName(), account2.getBalance()); //create a Scanner to obtain input from the command window Scanner input = new Scanner(System.in); //prompt the user to input a deposit amount System.out.print("Enter deposit amount for account1: "); double depositAmount = input.nextDouble(); //obtain user input System.out.printf("%nadding %.2f to account1 balance %n%n", depositAmount); account1.deposit(depositAmount); //add to account1's balance //display balances System.out.printf("%s balance: $%.2f%n", account1.getName(), account1.getBalance()); System.out.printf("%s balance: $%.2f%n", account2.getName(), account2.getBalance()); //prompt to input a deposit for account2 System.out.print("Enter deposit amount for account2: "); depositAmount = input.nextDouble(); //obtain user input System.out.printf("%nadding %.2f to account2 balance%n%n", depositAmount); //add to the account2 balance account2.deposit(depositAmount); //display balances System.out.printf("%s balance: $%.2f%n", account1.getName(), account1.getBalance()); System.out.printf("%s balance: $%.2f%n", account2.getName(), account2.getBalance()); }
THIS NEEDS TO BE DONE IN JAVA
Modify the Account class to provide a debit method that withdraws money from an Account. Ensure that the debit amount does not exceed the Account's balance. If it does, the balance should be left unchanged and the method should print out a message indicating "Debit amount exceeded amount balance." Modify class AccountTest to test method debit. Below are links to the Account and AccountTest programs.
//This is for the account class
private String name; //instance variable
private double balance; //instance variable
//Account constructor that receives two parameters
public Account(String name, double balance){
this.name = name; //assing name to instance varialbe name
//validate that the balance is greater than 0.0; if it's not,
//instance varisble balance keeps its default initial value of 0.0
if (balance > 0.0){ //if the balance is valaid
this.balance = balance; //assign it to instance variable balance
}
}
//method that deposits (adds) only a valid amount to the balance
public void deposit (double depositAmount){
if (depositAmount > 0.0) {
balance = balance + depositAmount; //add it to the balance
}
}
//method returns the account balance
public double getBalance(){
return balance;
}
//method that sets the name
public void setName(String name){
this.name = name;
}
//method that returns the name
public String getName(){
return name;
}
}
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
//create two Account objects
Account account1 = new Account("Jane Green", 50.00);
Account account2 = new Account("John Blue", -7.53);
//display initial balance of each object
System.out.printf("%s balance: $%.2f%n", account1.getName(),
account1.getBalance());
System.out.printf("%s balance: $%.2f%n", account2.getName(),
account2.getBalance());
//create a Scanner to obtain input from the command window
Scanner input = new Scanner(System.in);
//prompt the user to input a deposit amount
System.out.print("Enter deposit amount for account1: ");
double depositAmount = input.nextDouble(); //obtain user input
System.out.printf("%nadding %.2f to account1 balance %n%n",
depositAmount);
account1.deposit(depositAmount); //add to account1's balance
//display balances
System.out.printf("%s balance: $%.2f%n",
account1.getName(), account1.getBalance());
System.out.printf("%s balance: $%.2f%n",
account2.getName(), account2.getBalance());
//prompt to input a deposit for account2
System.out.print("Enter deposit amount for account2: ");
depositAmount = input.nextDouble(); //obtain user input
System.out.printf("%nadding %.2f to account2 balance%n%n",
depositAmount);
//add to the account2 balance
account2.deposit(depositAmount);
//display balances
System.out.printf("%s balance: $%.2f%n",
account1.getName(), account1.getBalance());
System.out.printf("%s balance: $%.2f%n",
account2.getName(), account2.getBalance());
}

The main objective of this task is to modify an existing Java class named Account to provide a method called debit which allows money to be withdrawn from the account. There are certain conditions that need to be met for a successful withdrawal: the debit amount should not exceed the account's balance. If the debit amount is higher than the balance, the account's balance should remain unchanged and a message should be displayed to the user stating "Debit amount exceeded account balance." Additionally, a class called AccountTest should be modified to test the debit method in various scenarios.
Algorithm:
- Begin by modifying the Account class to include a new method named debit.
- Within the debit method, compare the debit amount with the current account balance. a. If the debit amount is greater than the balance, print a message "Debit amount exceeded account balance." and do not change the account balance. b. If the debit amount is less than or equal to the balance, subtract the debit amount from the account balance.
- Now, move on to the AccountTest class to test the newly added debit method in the Account class.
- In the main method of the AccountTest class: a. Create two instances of the Account class with initial balance values. b. Prompt the user to enter deposit amounts and use the deposit method to add money to the accounts. c. Display the new balances. d. Now, prompt the user to enter debit amounts for both accounts. e. Call the debit method on both accounts with the user-provided debit amounts. f. Display the resulting balances after attempting to debit the specified amounts from both accounts.
- End of algorithm.
Step by step
Solved in 6 steps with 1 images

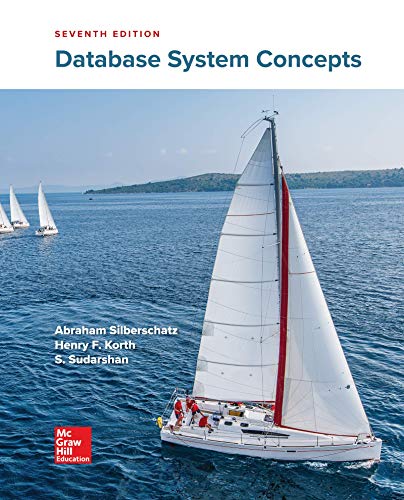
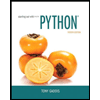
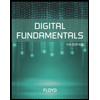
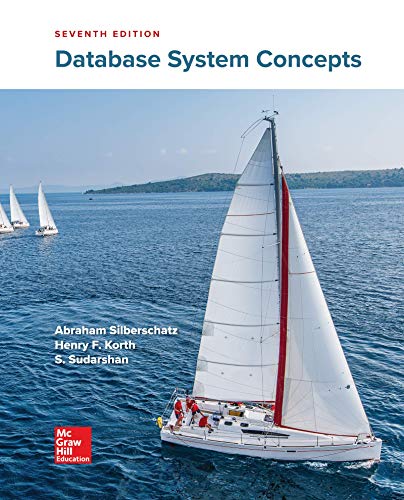
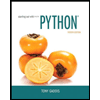
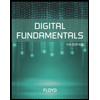
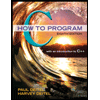
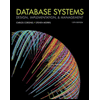
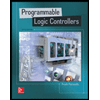