The program with the main method should be called ‘LetterCode.java’ The program with the class for performing the operations should be called LetterCodeLogic.java. The class should have two static methods: Encode and Decode (both of which receive a string parameter).
Need help with part B the decode method AND the getChoice method using try/catch
The program with the main method should be called ‘LetterCode.java’ The program with the class for performing the operations should be called LetterCodeLogic.java. The class should have two static methods: Encode and Decode (both of which receive a string parameter).
Part A: The part A program will be the Encode function
Part B: The Part B program will be the Decode function.
This is my code so far for LetterCode.java:
package lettercode;
import business.LetterCodeLogic;
import java.util.Scanner;
/**
*
* @author Sean Jeffries
*/
public class LetterCode {
static Scanner sc = new Scanner(System.in);
public static void main(String[] args) {
int choice;
String msg="", result="";
System.out.println("welcome to the Letter Code Program!");
choice = getChoice();
while (choice != 0) {
switch (choice) {
case 1: //encode
System.out.print("Enter your message to be encoded: ");
msg = sc.nextLine();
result = LetterCodeLogic.Encode(msg);
System.out.println("Your encoded message is:");
System.out.println(result);
break;
case 2: //decode
System.out.print("Enter your numbers to be decoded (seperate with commas): ");
msg = sc.nextLine();
result = LetterCodeLogic.Decode(msg);
System.out.println("Your decoded message is: ");
System.out.println(result);
break;
default:
System.out.println("Unknown operation\n");
break;
}//end of switch
choice = getChoice();
}//end of while
}//end of main
public static int getChoice() {
//must be robust with data validation and error trapping
int c;
System.out.print("Choice? 1=Encode, 2=Decode, 0=Quit: ");
c = Integer.parseInt(sc.nextLine());
return c;
}
}
And this is my code so far for the LetterCodeLogic.java
package business;
/**
*
* @author Sean Jeffries
*/
public class LetterCodeLogic {
public static String Encode(String msg) {
String m = msg.toUpperCase();
String result="";
//convert characters to numbers using the ASCII coding scheme
// A=65, B=66, C=67, etc. (space = 32)
//also use the fact that characters and integers are equivalent
//based on this scheme...
int x;
char c; //single character (not string) - use apostrophe
//pull characters froim the input string m
for(int i=0; i < m.length(); i++) {
c = m.charAt(i); //take string value at position i and turn it into a char
x = c; //creates value x from char using ASCII scheme
if (x == 32) {
x = 0; //convert value of space to zero
} else {
x -= 64; //adjust x to 1,2,3 etc.
if (x < 1 || x > 26) {
x = 99;
}
}
//character at position i of string is now a value in x
//of 0 for space, 1-26 for letter, or 99 for unkown character
result += String.valueOf(x) + " ";
}
return result;
}
public static String Decode(String msg) {
String result = "";
//input string looks like (ex): "1,2,3,4,5,6"
//need to extract numbers 1 2 3 4 5 6
//use the split method of string
String[] nums = msg.split(",");
int x=0;
char c;
for (int i = 0; i < nums.length; i++) {
//first step: convert value in current position i of array to integer...
//process here is reverse of encode: take number returned by conversion
//change it to space (ASCII 32) or capital letter (ASCII 65 and following
x = Integer.parseInt(nums[i]);
c = (char)x;
if (c == 0) {
c = (char)32;
} else {
c -= (char)64;
if (c < 65 || c > 90) {
c = (char)35;
}
}
result += String.valueOf(x) + " ";
}
return result;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

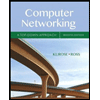
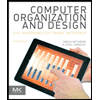
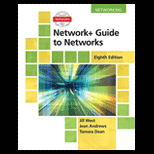
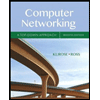
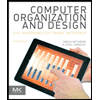
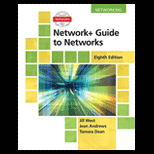
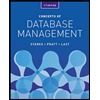
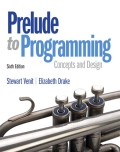
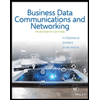