Exercise1: Write a program that takes three integer numbers from the console as inputs. For each number, the program should call a static method named isPositive() to determine if a number is positive, negative, or equal to zero.
java
Exercise1:
Write a program that takes three integer numbers from the console as inputs. For each number, the program should call a static method named isPositive() to determine if a number is positive, negative, or equal to zero.
Exercise 3:
The following code asks a user to enter her/his age then determines if a user is an adult or not. Trace the code, to find and solve the syntax errors. You need to identify the errors and rewrite the correct code.
import java.util.Scanner;
public class Ex3 {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.println("Enter your age:");
int age = input.nextInt();
double flag = isAdult(double age);
if(flag) {
System.out.println("You are an adult.");
} else {
System.out.println("You are not an adult.");
}
}
public boolean isAdult(int age) {
if(age > 18) {
return true;
} else {
return false;
}
}
}
Exercise 4:
Write a program to do the following using the Math class:
- Ask a user to enter two integer numbers
- Finds the square root of each number
- Finds the minimum
- Finds the maximum
Exercise 5:
Write a program to calculate area of circle. The main method should pass the radius of the circle to a static method named calculateArea(). The program should display the calculated area inside the main method.

Step by step
Solved in 2 steps with 1 images

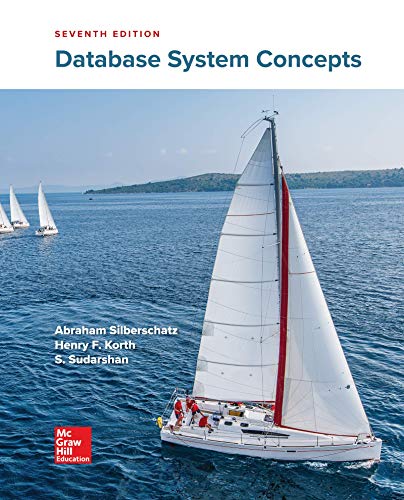
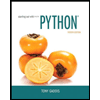
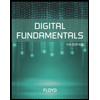
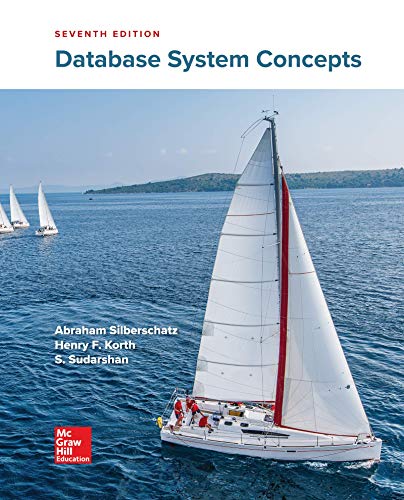
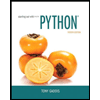
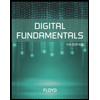
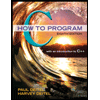
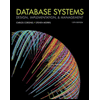
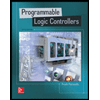