Start by understanding the problem:
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:Now, here is a description of HOW you should write the program:
Start by understanding the problem:
• Read the question carefully, and identify the things you are sure about, and the things you are unsure
about. If you have any questions, make sure to ask an instructor, a TA or post on the class discussion
forums
Next, prepare some test cases. A test case is a set of input values for the program where you will be
able to compare the result your program gives you with the result that you are expecting (because you
calculated it by hand). Prepare three examples different from those on the previous page to demonstrate
that your program works.
For this problem, you will need to hand in your testing in a separate document. This can be a simple text
document if you like, where for each of the 3 test cases, you state something like: "I used A stacks of eggs.
B stacks of sugar, C stacks of wheat and D buckets of milk. This should yield a result of X total cakes. My
program gave a result of Y total cakes. Of course, if your program is working, X and Y will be the same.
but the whole point of testing is not to assume that this will be the case in advance!
Next, start writing your code by creating a function called make_cakes (). This function should accept
four parameters, the number of stacks of eggs, the number of stacks of sugar, the number of stacks of
wheat and the number of buckets of milk. It should follow these steps:
• Calculate how many eggs, sugars, wheats and milks there are based on the number of stacks of each
• Calculate how many complete cakes can be made based on the recipe for cakes provided above
• Return the number of complete cakes you can make
Once this function is done, you can (and should!) test it before adding any further components of the
program. You can do this by just writing a few function calls with hard-coded, literal arguments and just
printing out the return value. You don't need to hand in this intermediate testing, but it's a good habit to
get into and will save you time in the long run!
Finally, add the interactive components to the program. This will include handling the keyboard input to
modify the amount of raw ingredients available as well as displaying the program's output to the Processing
canvas. Note that you should be displaying the total number of ingredients available, not the number of
stacks entered (even though it's the STACKS that increase when the user presses a key!).
Here's how the program might look like while it is running:
0
wton hand
0
Cam
0
0
backets an and
0
og on hand
32
hand
128
Camale
32
192
backets and
150

Transcribed Image Text:Minecraft is an open-world video game based on placing and interacting with 3D textured blocks. If you
are not familiar with the game, each player has a personal inventory which can hold a limited number of
blocks and items. Specific blocks and items can be combined on a crafting table to form new items. For
example, three wheats arranged in a horizontal line on a crafting table gives the player one loaf of bread.
These combinations are known as crafting recipes and the game has hundreds of them.
This question deals with the recipe for making a cake. One cake requires 1 egg. 2 sugars, 3 wheats and
3 buckets of milk. Your job is to build a program to help the player determine how many cakes they can
make based on the number of ingredients that they have.
Crafting
Figure 2: A screenshot from Minecraft showing a crafting table showing the recipe for making a
cake. Your program won't make this picture, this is just showing you the rules for making a cake!
Most items in the player's inventory - such as sugar and wheat - can be stacked in groups of 64. This
allows the player to store 64 of the same item in one inventory slot. Some items - such as eggs - can
only be stacked in groups of 16. Finally, items like buckets of milk cannot be stacked; each bucket of milk
occupies one inventory slot.
Here is a description of WHAT your program should do when it's finished:
• Pressing the "e" key/"r" key increases/decreases the number of STACKS of eggs available by one.
• Pressing the 's" key/'d' key increases/decreases the number of STACKS of sugar available by one.
• Pressing the "w" key/"q" key increases/decreases the number of STACKS of wheat available by one.
Pressing the "m" key/'n' key increases/decreases the number of individual buckets of milk available
by one.
• Display the current number of eggs, the current number of sugar, the current number of wheat and
the current number of milk available.
• Display the number of cakes the player could possibly make given the amount of raw ingredients.
Some examples:
If you have 1 stack of eggs (16 eggs total), 1 stack of sugar (64 sugars total), 1 stack of wheat (64 wheats
total) and 3 buckets of milk, you can only make 1 complete cake before you run out of milk.
• If you have 2 stacks of eggs (32 eggs total), 3 stacks of sugar (192 sugars total), 2 stacks of wheat (128
wheats total) and 150 buckets of milk, you can make 32 complete cakes before you run out of eggs.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
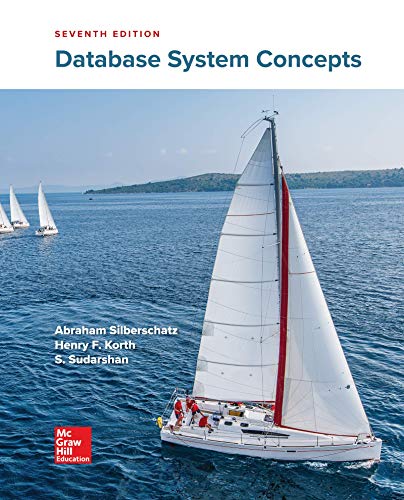
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
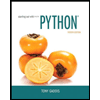
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
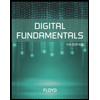
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
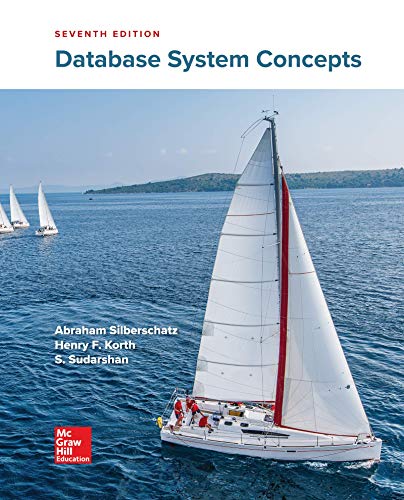
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
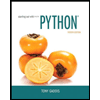
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
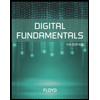
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
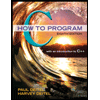
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
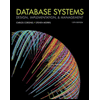
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
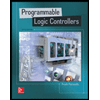
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education