self.title = title self.director = director self.producer = producer self.release_date = release_date self.duration = duration self.dvd_id = dvd_id
class dvd:
def __init__(self, title, director, producer, release_date, duration, dvd_id):
self.title = title
self.director = director
self.producer = producer
self.release_date = release_date
self.duration = duration
self.dvd_id = dvd_id
def get_title(self):
return self.title
def get_director(self):
return self.director
def get_producer(self):
return self.producer
def get_release_date(self):
return self.release_date
def get_duration(self):
return self.duration
def get_dvd_id(self):
return self.dvd_id
class customer:
def __init__(self, name, customer_id):
self.name = name
self.customer_id = customer_id
def get_name(self):
return self.name
def get_customer_id(self):
return self.customer_id
class store:
def __init__(self):
self.dvds = []
self.customers = []
def add_dvd(self, dvd):
self.dvds.append(dvd)
def add_customer(self, customer):
self.customers.append(customer)
def rent_dvd(self, dvd_id, customer_id):
for dvd in self.dvds:
if dvd.get_dvd_id() == dvd_id:
for customer in self.customers:
if customer.get_customer_id() == customer_id:
customer.dvds.append(dvd)
self.dvds.remove(dvd)
def return_dvd(self, dvd_id, customer_id):
for customer in self.customers:
if customer.get_customer_id() == customer_id:
for dvd in customer.dvds:
if dvd.get_dvd_id() == dvd_id:
self.dvds.append(dvd)
customer.dvds.remove(dvd)
def get_dvd(self, dvd_id):
for dvd in self.dvds:
if dvd.get_dvd_id() == dvd_id:
return dvd
def get_customer(self, customer_id):
for customer in self.customers:
if customer.get_customer_id() == customer_id:
return customer
def get_dvds(self):
return self.dvds
def get_customers(self):
return self.customers
def get_dvds_for_customer(self, customer_id):
for customer in self.customers:
if customer.get_customer_id() == customer_id:
return customer.dvds
# main
store = store()
dvd1 = dvd("The Godfather", "Francis Ford Coppola", "Albert S. Ruddy", 1972, 175, 1)
dvd2 = dvd("The Shawshank Redemption", "Frank Darabont", "Niki Marvin", 1994, 142, 2)
dvd3 = dvd("Pulp Fiction", "Quentin Tarantino", "Lawrence Bender", 1994, 154, 3)
store.add_dvd(dvd1)
store.add_dvd(dvd2)
store.add_dvd(dvd3)
customer1 = customer("John Smith", 1)
customer2 = customer("Mary Johnson", 2)
customer3 = customer("Mike Williams", 3)
store.add_customer(customer1)
store.add_customer(customer2)
store.add_customer(customer3)
store.rent_dvd(1, 1)
store.rent_dvd(2, 1)
store.rent_dvd(3, 2)
store.rent_dvd(3, 3)
store.return_dvd(2, 1)
print(store.get_dvd(1))
print(store.get_dvd(2))
print(store.get_dvd(3))
print(store.get_customer(1))
print(store.get_customer(2))
print(store.get_customer(3))
print(store.get_dvds())
print(store.get_customers())
print(store.get_dvds_for_customer(1))
print(store.get_dvds_for_customer(2))
print(store.get_dvds_for_customer(3))
Attribute Error: 'customer' object has no attribute 'dvds'
Eliminate This Attribute Error.
![def __init__(self, name, customer_id):
self.name = name
self.dvds = []
self.customer_id = customer_id](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F354d04cb-1bbf-4eaa-a003-30513d4daa29%2Fee9f65e7-ece1-40e7-83eb-f0a94a4175e9%2Fob7iqa_processed.png&w=3840&q=75)

Step by step
Solved in 4 steps with 6 images

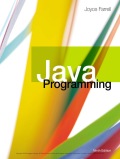
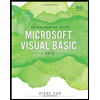
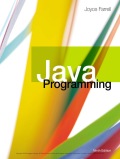
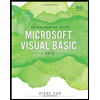