Replace all occurrences of the identifier vector with the identifier list inDisplay 19.17. Compile and run the program. Display 19.17 The Generic find Function1 //Program to demonstrate use of the generic find function.2 #include <iostream>3 #include <vector>4 #include <algorithm>5 using std::cin;6 using std::cout;7 using std::endl;8 using std::vector;9 using std::find;10 int main( )11 {12 vector<char> line; (continued) 13 cout << "Enter a line of text:\n";14 char next;15 cin.get(next);16 while (next != '\n';17 {18 line.push_back(next);19 cin.get(next);20 }21 vector<char>::const_iterator where;22 where = find(line.begin( ), line.end( ), 'e');23 //where is located at the first occurrence of 'e' in v.24 vector<char>::const_iterator p;25 cout << "You entered the following before you" << "entered your first line:\n";26 for (p = line.begin( ); p != where; p++)27 cout << *p;28 cout << endl;29 cout << "You entered the following after that:\n";30 for (p = where; p != line.end( ); p++)31 cout << *p;32 cout << endl;33 cout << "End of demonstration.\n";34 return 0;35 }
Replace all occurrences of the identifier
Display 19.17. Compile and run the program.
Display 19.17 The Generic find Function
1 //Program to demonstrate use of the generic find function.
2 #include <iostream>
3 #include <vector>
4 #include <algorithm>
5 using std::cin;
6 using std::cout;
7 using std::endl;
8 using std::vector;
9 using std::find;
10 int main( )
11 {
12 vector<char> line; (continued)
13 cout << "Enter a line of text:\n";
14 char next;
15 cin.get(next);
16 while (next != '\n';
17 {
18 line.push_back(next);
19 cin.get(next);
20 }
21 vector<char>::const_iterator where;
22 where = find(line.begin( ), line.end( ), 'e');
23 //where is located at the first occurrence of 'e' in v.
24 vector<char>::const_iterator p;
25 cout << "You entered the following before you"
<< "entered your first line:\n";
26 for (p = line.begin( ); p != where; p++)
27 cout << *p;
28 cout << endl;
29 cout << "You entered the following after that:\n";
30 for (p = where; p != line.end( ); p++)
31 cout << *p;
32 cout << endl;
33 cout << "End of demonstration.\n";
34 return 0;
35 }

Step by step
Solved in 2 steps with 1 images

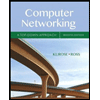
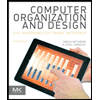
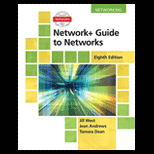
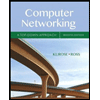
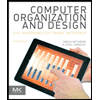
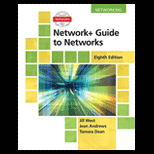
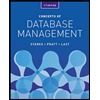
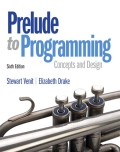
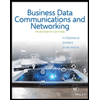