Question: Explain the double integrate (FUNC f, double a, double b); line of the integrate function. Given the C++ code: // integration.cpp #include using namespace std; // FUNC represents functions of one variable that take a double as input and returns a double typedef double (*FUNC)(double); double integrate(FUNC f, double a, double b); double line(double x); double square(double x); double cube(double x); int main(int argc, char* argv[]){ cout << "The integral of f(x)=x between 1 and 5 is: " << integrate(line, 1, 5) << endl; // 25/2 - 1/2 cout << "The integral of f(x)=x^2 between 1 and 5 is: " << integrate(square, 1, 5) << endl; // 125/3 - 1/3 cout << "The integral of f(x)=x^3 between 1 and 5 is: " << integrate(cube, 1, 5) << endl; // 625/4 - 1/4 return 0; } double integrate(FUNC f, double a, double b){ double temp=0.0; for(double x = a; x<=b; x=(x+.0000001)){ // this loop adds up the areas of small boxes for that function temp = temp+(f(x)*.0000001); } return temp; } // end of integrate double line(double x){ return x; } // end of line double square(double x){ return x*x; } // end of square double cube(double x){ return x*x*x; } // end of cube
Question: Explain the double integrate (FUNC f, double a, double b); line of the integrate function.
Given the C++ code:
// integration.cpp
#include <iostream>
using namespace std;
// FUNC represents functions of one variable that take a double as input and returns a double
typedef double (*FUNC)(double);
double integrate(FUNC f, double a, double b);
double line(double x);
double square(double x);
double cube(double x);
int main(int argc, char* argv[]){
cout << "The integral of f(x)=x between 1 and 5 is: " << integrate(line, 1, 5) << endl; // 25/2 - 1/2
cout << "The integral of f(x)=x^2 between 1 and 5 is: " << integrate(square, 1, 5) << endl; // 125/3 - 1/3
cout << "The integral of f(x)=x^3 between 1 and 5 is: " << integrate(cube, 1, 5) << endl; // 625/4 - 1/4
return 0;
}
double integrate(FUNC f, double a, double b){
double temp=0.0;
for(double x = a; x<=b; x=(x+.0000001)){ // this loop adds up the areas of small boxes for that function
temp = temp+(f(x)*.0000001);
}
return temp;
} // end of integrate
double line(double x){
return x;
} // end of line
double square(double x){
return x*x;
} // end of square
double cube(double x){
return x*x*x;
} // end of cube

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

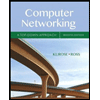
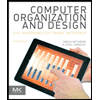
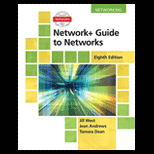
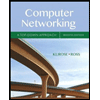
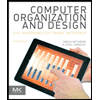
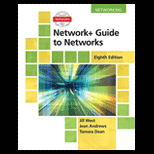
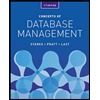
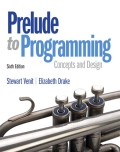
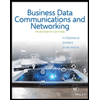