2. Write a C++ function that takes a string of even length as a parameter and returns the first half of that string.
2. Write a C++ function that takes a string of even length as a parameter and returns the first half of that string.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:### Task 2: C++ String Function
**Objective:**
Write a C++ function that takes a string of even length as a parameter and returns the first half of that string.
**Instructions:**
- The function should accept a string parameter.
- Ensure the string has an even number of characters before processing.
- Return a new string that consists of the first half of the original string.
**Example:**
For an input string "HelloWorld", the function should return "Hello".
**Code Skeleton:**
```cpp
#include <iostream>
#include <string>
std::string getFirstHalf(const std::string &input) {
if (input.length() % 2 != 0) {
throw std::invalid_argument("String length must be even.");
}
return input.substr(0, input.length() / 2);
}
int main() {
std::string str = "HelloWorld";
std::cout << "First half: " << getFirstHalf(str) << std::endl;
return 0;
}
```
**Discussion:**
This task helps in understanding string manipulation and validation in C++. The primary operations involve checking the string length and using substring methods.
Expert Solution

Step 1
Write a program in C++ language which will take a string with even number of characters and then display the first half of that string.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
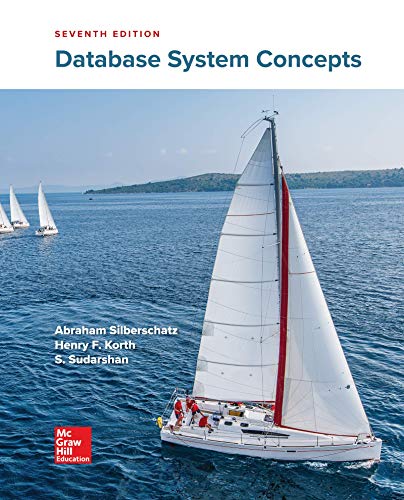
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
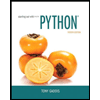
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
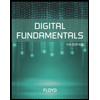
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
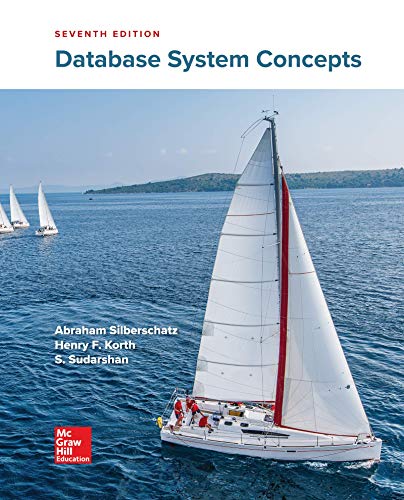
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
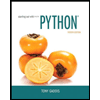
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
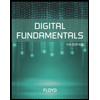
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
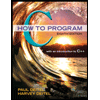
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
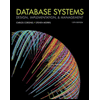
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
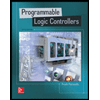
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education