In c++ In terms of testing and debugging functions, define stubs and drivers. Create a driver for the function you created in the previous volume problem. Show your source code and a sample of the program output. Does your function produce accurate results? previous code: #include #include using namespace std; const float PI = 3.14; float ConeVolume(float radius, float height) { return (PI / 3) * pow(radius, 2) * height; } int main() { float radius, height, volume; cout << "Enter the radius: "; cin >> radius; cout << "Enter the height: "; cin >> height; volume = ConeVolume(radius, height); cout << "The volume of the cone is: " << volume << endl; return 0; }
In c++
In terms of testing and debugging functions, define stubs and drivers. Create a driver for the function you created in the previous volume problem. Show your source code and a sample of the program output. Does your function produce accurate results?
previous code:
#include <iostream>
#include <cmath>
using namespace std;
const float PI = 3.14;
float ConeVolume(float radius, float height)
{
return (PI / 3) * pow(radius, 2) * height;
}
int main()
{
float radius, height, volume;
cout << "Enter the radius: ";
cin >> radius;
cout << "Enter the height: ";
cin >> height;
volume = ConeVolume(radius, height);
cout << "The volume of the cone is: " << volume << endl;
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

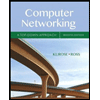
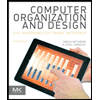
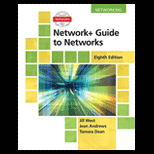
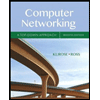
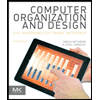
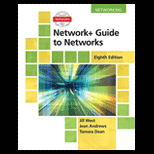
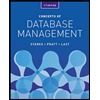
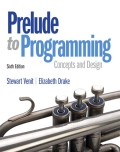
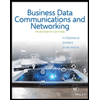