Question 8: Questions 8 - 12 are based on the following code. Now, this code results in a compile error (where it's marked "here"). Why? class Fraction { private: int numerator; int denominator; public: Fraction(int n, int d) { numerator = n; denominator = d; } void display() { cout << numerator << "/" << denominator << endl; } }; int main() { Fraction f; // <-- here return 0; } Group of answer choices There is no constructor defined Copy constructor is undefined Default constructor is undefined Constructor is defined as public Question 9: Find an incorrect definition of getter/setter for Fraction. Group of answer choices void setNumerator(int n) { numerator = n; } void getNumerator() { return numerator; } void setDenominator(int d) { denominator = d; } int getDenominator() { return denominator; } Question 10: What would be the printout of the following code segment? Fraction f(2, 3); f.display(); Group of answer choices 23 2 3 2,3 2/3 Question 11: Suppose I added the following copy constructor to Fraction. Which statement will invoke this method? Fraction(const Fraction& b) { denominator = b.denominator; numerator = b.numerator; } Group of answer choices Fraction f(2, 3); Fraction g = f; Fraction h; Fraction* j = nullptr; Question 12: Suppose I added the following overloaded operator method to Fraction. Which statement will invoke this method? Fraction operator+(const Fraction& b) { Fraction o; o.denominator = denominator * b.denominator; o.numerator = numerator * b.denominator + b.numerator * denominator; return o; } Group of answer choices Fraction f(2, 3); Fraction g = f; Fraction h; Fraction h = f + g;
Question 8:
Questions 8 - 12 are based on the following code. Now, this code results in a compile error (where it's marked "here"). Why?
class Fraction {
private:
int numerator;
int denominator;
public:
Fraction(int n, int d) {
numerator = n;
denominator = d;
}
void display() { cout << numerator << "/" << denominator << endl; }
};
int main() {
Fraction f; // <-- here
return 0;
}
What would be the printout of the following code segment?
Fraction f(2, 3);
f.display();
Suppose I added the following copy constructor to Fraction. Which statement will invoke this method?
Fraction(const Fraction& b) {
denominator = b.denominator;
numerator = b.numerator;
}
Suppose I added the following overloaded operator method to Fraction. Which statement will invoke this method?
Fraction operator+(const Fraction& b) {
Fraction o;
o.denominator = denominator * b.denominator;
o.numerator = numerator * b.denominator + b.numerator * denominator;
return o;
}

Step by step
Solved in 5 steps

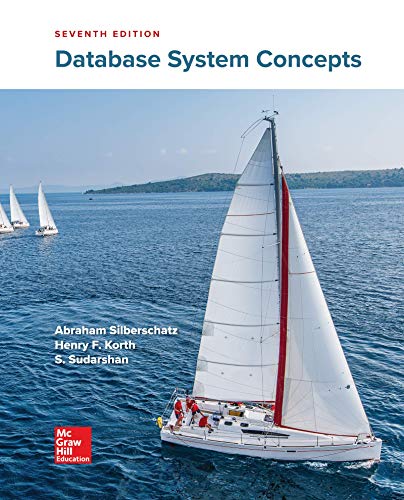
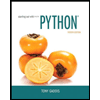
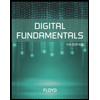
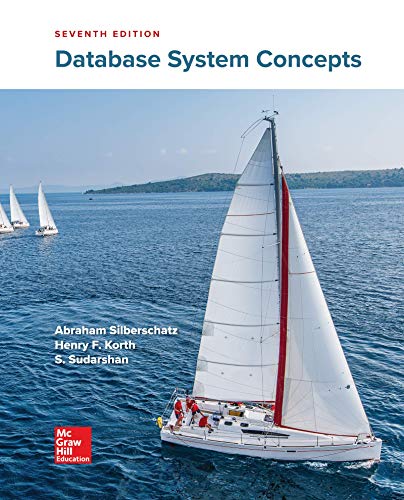
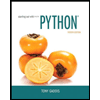
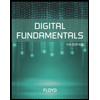
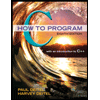
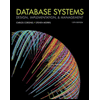
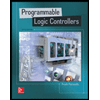