Python Define a member method print_all() for class PetData. Make use of the base class' print_all() method. Sample output for the given program with inputs: 'Fluffy' 5 4444 Name: Fluffy Age: 5 ID: 4444 class AnimalData: def __init__(self): self.full_name = '' self.age_years = 0 def set_name(self, given_name): self.full_name = given_name def set_age(self, num_years): self.age_years = num_years # Other parts omitted def print_all(self): print('Name:', self.full_name) print('Age:', self.age_years) class PetData(AnimalData): def __init__(self): AnimalData.__init__(self) self.id_num = 0 def set_id(self, pet_id): self.id_num = pet_id # FIXME: Add print_all() member method ''' Your solution goes here ''' user_pet = PetData() user_pet.set_name(input()) user_pet.set_age(int(input())) user_pet.set_id(int(input())) user_pet.print_all()
Python
Define a member method print_all() for class PetData. Make use of the base class' print_all() method.
Sample output for the given program with inputs: 'Fluffy' 5 4444
Name: Fluffy
Age: 5
ID: 4444
class AnimalData:
def __init__(self):
self.full_name = ''
self.age_years = 0
def set_name(self, given_name):
self.full_name = given_name
def set_age(self, num_years):
self.age_years = num_years
# Other parts omitted
def print_all(self):
print('Name:', self.full_name)
print('Age:', self.age_years)
class PetData(AnimalData):
def __init__(self):
AnimalData.__init__(self)
self.id_num = 0
def set_id(self, pet_id):
self.id_num = pet_id
# FIXME: Add print_all() member method
''' Your solution goes here '''
user_pet = PetData()
user_pet.set_name(input())
user_pet.set_age(int(input()))
user_pet.set_id(int(input()))
user_pet.print_all()

Code:
#Define the class AnimalData.
class AnimalData:
#constructor its member variables full name and age.
def __init__(self):
self.full_name = ''
self.age_years = 0
#Defining the method set_name() to set the name of the animal
def set_name(self, given_name):
self.full_name = given_name
#Defining the method set_age() to set the age of the animal
def set_age(self, num_years):
self.age_years = num_years
#Defining the method print_all() to display animal's name and age
def print_all(self):
print('Name:', self.full_name)
print('Age:', self.age_years)
#Defining the class PetData inheriting the base class AnimalData
class PetData(AnimalData):
#Defining the constructor of the class.
def __init__(self):
#Calling the base class constructor.
AnimalData.__init__(self)
self.id_num = 0
#Defining the method set_id() to set the id of the pet
def set_id(self, pet_id):
self.id_num = pet_id
#Overriding the method print_all() in the child class PetData() to display pet's name, age, and id
def print_all(self):
#Call the base class print_all() method to display pet name and age.
AnimalData.print_all(self)
#Display pet id.
print('ID:',self.id_num)
#Create an object of PetData class and call the
#constructor of that class.
user_pet = PetData()
#Call the set_name() method and pass the value
#'Fluffy' as name of the pet.
user_pet.set_name('Fluffy')
#Call the set_age() method and pass the value 5
#as age of the pet.
user_pet.set_age(5)
#Call the set_id() method and pass the value
#4444 as id of the pet.
user_pet.set_id(4444)
#Call the base class print_all() method to
#display required details about pet.
user_pet.print_all()
Code Screenshot:
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

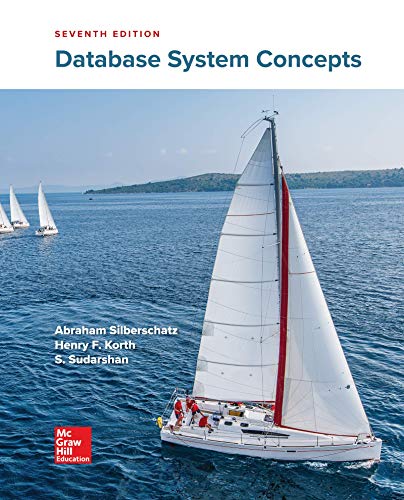
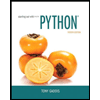
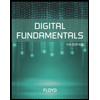
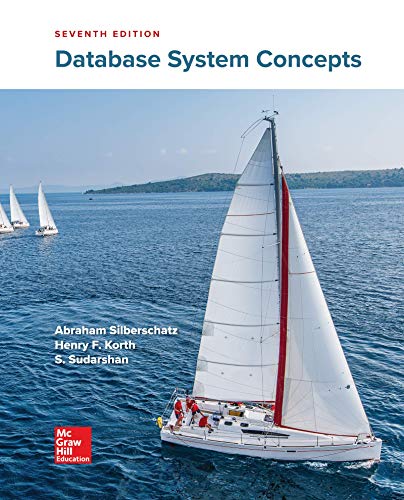
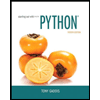
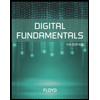
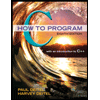
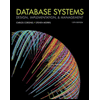
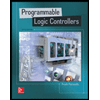