Python 3 import random from breezypythongui import EasyFrame class GuessingGame(EasyFrame): """Plays a guessing game with the user.""" def __init__(self): """Sets up the window,widgets, and data.""" EasyFrame.__init__(self, title = "Guessing Game") # self.lowerBound # self.upperBound # self.count self.myNumber = (self.lowerBound + self.upperBound) // 2 guess = "Is the number " + str(self.myNumber) + "?" self.myLabel = self.addLabel(text = guess, row = 0, column = 0, sticky = "NSEW", columnspan = 4) self.small = self.addButton(text = "Too small", row = 1, column = 0, command = self.goLarge) self.large = self.addButton(text = "Too large", row = 1, column = 1, command = self.goSmall) self.correct = self.addButton(text = "Correct", row = 1, column = 2, command = self.goCorrect) self.newButton = self.addButton(text = "New game", row = 1, column = 3, command = self.newGame) def goLarge(self): """Guess was too small, so move guess to the right of the number.""" # Write code here def goSmall(self): """Guess was too large, so move guess to the left of the number.""" # Write code here def goCorrect(self): """Guess was too correct, so announce and wait.""" # Write code here def newGame(self): """Resets the GUI to its original state.""" # Write code here def main(): """Instantiate and pop up the window.""" GuessingGame().mainloop() if __name__ == "__main__": try: while True: main() except KeyboardInterrupt: print("\nProgram closed.")
Python 3 import random from breezypythongui import EasyFrame class GuessingGame(EasyFrame): """Plays a guessing game with the user.""" def __init__(self): """Sets up the window,widgets, and data.""" EasyFrame.__init__(self, title = "Guessing Game") # self.lowerBound # self.upperBound # self.count self.myNumber = (self.lowerBound + self.upperBound) // 2 guess = "Is the number " + str(self.myNumber) + "?" self.myLabel = self.addLabel(text = guess, row = 0, column = 0, sticky = "NSEW", columnspan = 4) self.small = self.addButton(text = "Too small", row = 1, column = 0, command = self.goLarge) self.large = self.addButton(text = "Too large", row = 1, column = 1, command = self.goSmall) self.correct = self.addButton(text = "Correct", row = 1, column = 2, command = self.goCorrect) self.newButton = self.addButton(text = "New game", row = 1, column = 3, command = self.newGame) def goLarge(self): """Guess was too small, so move guess to the right of the number.""" # Write code here def goSmall(self): """Guess was too large, so move guess to the left of the number.""" # Write code here def goCorrect(self): """Guess was too correct, so announce and wait.""" # Write code here def newGame(self): """Resets the GUI to its original state.""" # Write code here def main(): """Instantiate and pop up the window.""" GuessingGame().mainloop() if __name__ == "__main__": try: while True: main() except KeyboardInterrupt: print("\nProgram closed.")
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Python 3
import random
from breezypythongui import EasyFrame
class GuessingGame(EasyFrame):
"""Plays a guessing game with the user."""
def __init__(self):
"""Sets up the window,widgets, and data."""
EasyFrame.__init__(self, title = "Guessing Game")
# self.lowerBound
# self.upperBound
# self.count
self.myNumber = (self.lowerBound + self.upperBound) // 2
guess = "Is the number " + str(self.myNumber) + "?"
self.myLabel = self.addLabel(text = guess,
row = 0, column = 0,
sticky = "NSEW",
columnspan = 4)
self.small = self.addButton(text = "Too small", row = 1,
column = 0, command = self.goLarge)
self.large = self.addButton(text = "Too large", row = 1,
column = 1,
command = self.goSmall)
self.correct = self.addButton(text = "Correct", row = 1,
column = 2,
command = self.goCorrect)
self.newButton = self.addButton(text = "New game", row = 1,
column = 3,
command = self.newGame)
def goLarge(self):
"""Guess was too small, so move guess to the right of the number."""
# Write code here
def goSmall(self):
"""Guess was too large, so move guess to the left of the number."""
# Write code here
def goCorrect(self):
"""Guess was too correct, so announce and wait."""
# Write code here
def newGame(self):
"""Resets the GUI to its original state."""
# Write code here
def main():
"""Instantiate and pop up the window."""
GuessingGame().mainloop()
if __name__ == "__main__":
try:
while True:
main()
except KeyboardInterrupt:
print("\nProgram closed.")

Transcribed Image Text:Write a GUI-based program that plays a guess-the-number game in which the roles of the
computer and the user are the reverse of what they are in the Case Study of this chapter. In this
version of the game, the computer guesses a number between 1 (lowerBound) and 100
(upperBound) and the user provides the responses.
• The window should display the computer's guesses with a label.
The user enters a hint in response, by selecting one of a set of command buttons labeled Too
small, Too large, and Correct.
• When the game is over, you should disable these buttons and wait for the user to click New
game, as before.
Be sure to use the field names provided in the comments in your starter code.
An example of the program is shown below:
Guessing Game
Is the number 50?
Too small
Too large
Correct
New game
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
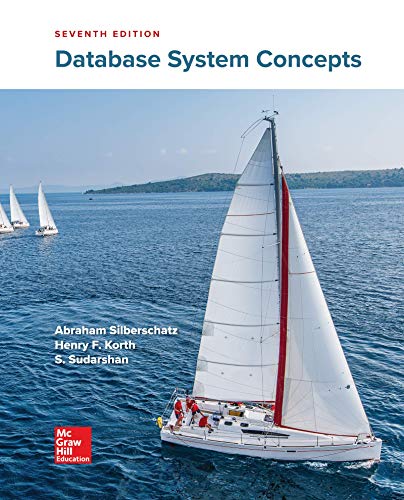
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
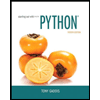
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
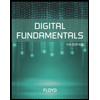
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
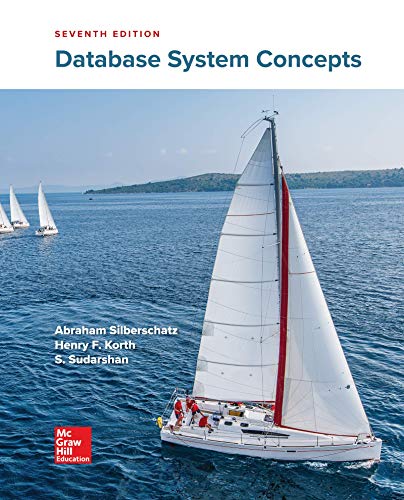
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
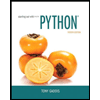
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
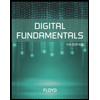
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
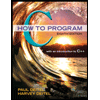
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
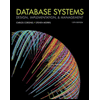
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
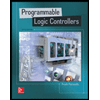
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education