transfer this code into GUI Tkinter in python print("Welcome to the ATM software") class ATM: def __init__(self): self.accounts = {} def create_account(self): first_name = input("Enter first name: ") last_name = input("Enter last name: ") dob = input("Enter date of birth (dd/mm/yyyy): ") address = input("Enter address: ") debit_card = input("Enter debit card number (16 digits): ") while len(debit_card) != 16 or not debit_card.isdigit(): debit_card = input("Invalid debit card number. Enter debit card number (16 digits): ") pin = input("Enter 4-digit pin: ") while len(pin) != 4 or not pin.isdigit(): pin = input("Invalid pin. Enter 4-digit pin: ") self.accounts[debit_card] = {"first_name": first_name, "last_name": last_name, "dob": dob, "address": address, "pin": pin, "balance": 0} print("Account created successfully!") def login(self): debit_card = input("Enter debit card number: ") pin = input("Enter pin: ") if debit_card in self.accounts and self.accounts[debit_card]["pin"] == pin: while True: print("1. Deposit\n2. Withdraw\n3. View balance\n4. Logout") choice = input("Enter your choice: ") if choice == "1": amount = float(input("Enter deposit amount: ")) self.accounts[debit_card]["balance"] += amount print("Deposit successful. Current balance : $", self.accounts[debit_card]["balance"]) elif choice == "2": amount = float(input("Enter withdraw amount: ")) if amount <= self.accounts[debit_card]["balance"]: self.accounts[debit_card]["balance"] -= amount print("Withdraw successful. Current balance : $", self.accounts[debit_card]["balance"]) else: print("Insufficient balance.") elif choice == "3": print("Current balance: $", self.accounts[debit_card]["balance"]) elif choice == "4": print("Logged out.") break else: print("Invalid choice.") else: print("Invalid debit card or pin.") atm = ATM() while True: print("1. Create account\n2. Login\n3. Exit") choice = input("Enter your choice: ") if choice == "1": atm.create_account() elif choice == "2": atm.login() elif choice == "3": print("Exiting...") break else: print("Invalid choice.")
transfer this code into GUI Tkinter in python
print("Welcome to the ATM software")
class ATM:
def __init__(self):
self.accounts = {}
def create_account(self):
first_name = input("Enter first name: ")
last_name = input("Enter last name: ")
dob = input("Enter date of birth (dd/mm/yyyy): ")
address = input("Enter address: ")
debit_card = input("Enter debit card number (16 digits): ")
while len(debit_card) != 16 or not debit_card.isdigit():
debit_card = input("Invalid debit card number. Enter debit card number (16 digits): ")
pin = input("Enter 4-digit pin: ")
while len(pin) != 4 or not pin.isdigit():
pin = input("Invalid pin. Enter 4-digit pin: ")
self.accounts[debit_card] = {"first_name": first_name, "last_name": last_name, "dob": dob, "address": address,
"pin": pin, "balance": 0}
print("Account created successfully!")
def login(self):
debit_card = input("Enter debit card number: ")
pin = input("Enter pin: ")
if debit_card in self.accounts and self.accounts[debit_card]["pin"] == pin:
while True:
print("1. Deposit\n2. Withdraw\n3. View balance\n4. Logout")
choice = input("Enter your choice: ")
if choice == "1":
amount = float(input("Enter deposit amount: "))
self.accounts[debit_card]["balance"] += amount
print("Deposit successful. Current balance : $", self.accounts[debit_card]["balance"])
elif choice == "2":
amount = float(input("Enter withdraw amount: "))
if amount <= self.accounts[debit_card]["balance"]:
self.accounts[debit_card]["balance"] -= amount
print("Withdraw successful. Current balance : $", self.accounts[debit_card]["balance"])
else:
print("Insufficient balance.")
elif choice == "3":
print("Current balance: $", self.accounts[debit_card]["balance"])
elif choice == "4":
print("Logged out.")
break
else:
print("Invalid choice.")
else:
print("Invalid debit card or pin.")
atm = ATM()
while True:
print("1. Create account\n2. Login\n3. Exit")
choice = input("Enter your choice: ")
if choice == "1":
atm.create_account()
elif choice == "2":
atm.login()
elif choice == "3":
print("Exiting...")
break
else:
print("Invalid choice.")
![PC File Edit View Navigate Code Refactor Run Tools VCS Window Help
Desktop final initial code trial.py
Structure Bookmarks
Project
> Desktop C:\Users\ihaba\Desktop
> III External Libraries
▬
T
Scratches and Consoles
Version Control ✔ Python Packages
Q Search
56°
E TODO
l
Python Console
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
final initial code trial.py - final initial code trial.py
final initial code trial.py
A
A
A
A
B
A
Ĥ
A
print("Welcome to the ATM software")
class ATM:
A
A
A
A
def __init__(self):
self.accounts = {}
def create account (self):
first_name = input("Enter first name: ")
last_name = input("Enter last name: "
dob=input("Enter date of birth (dd/mm/yyyy): ")
address=input("Enter address: ")
debit card=input("Enter debit card number (16 digits): ")
while len(debit_card) != 16 or not debit_card.isdigit():
debit card=input("Invalid debit card number. Enter debit card number (16 digits): ")
• Problems > Terminal
pin = input("Enter 4-digit pin: ")
while len(pin) != 4 or not pin.isdigit():
pin = input("Invalid pin. Enter 4-digit pin: ")
self.accounts [debit_card] = {"first_name": first_name, "last_name": last_name, "dob": dob, "address": address,
def login(self):
print("Account created successfully!")
ATM > create_account()
debit_card=input("Enter debit card number: ")
pin = input("Enter pin: ")
if debit card in self.accounts and self.accounts [debit_card]["pin"] == pin:
while True:
print("1. Deposit\n2. Withdraw\n3. View balance\n4. Logout")
choice = input ("Enter your choice: ")
if choice == "1":
"pin": pin, "balance": 0}
99-
Services
amount = float(input("Enter deposit amount: "))
self.accounts [debit_card] ["balance"] += amount
print("Deposit successful. Current balance: $", self.accounts [debit_card] ["balance"])
elif choice == "2":
amount = float(input("Enter withdraw amount: "))
if amount <= self.accounts [debit_card] ["balance"]:
self.accounts [debit_card] ["balance"] -= amount
print("Withdraw successful. Current balance: $", self.accounts [debit_card] ["balance"])
else:
print("Insufficient balance.")
elif choice == "3":"
print("Current balance: $", self.accounts [debit_card] ["balance"])
Current File ▾
$
N
ENG
Q
A 2
⠀
8:47 CRLF UTF-8 4 spaces Python 3.11
9:30 PM
2/19/2023
Notifications
Q Search
56°
i
H
36
99+
prime
video
CORSAIR
NGERNOBLED
XXXXX
20
6666
B
V
0094
| ()▬▬
ENDE
CORSAIR
PC
Sese
9:30 PM
2/19/2023](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6efda218-3274-4861-a930-554e0197f95c%2F373095fb-dbea-4c6a-9ebf-13129630b30c%2F8cnl5ua_processed.png&w=3840&q=75)
![PC File Edit View Navigate Code Refactor Run Tools VCS Window Help
Desktop final initial code trial.py
Structure Bookmarks
Project
> Desktop C:\Users\ihaba\Desktop
> III External Libraries
▬
T
Scratches and Consoles
Version Control ✔ Python Packages
Q Search
56°
E TODO
l
Python Console
29
30
31
32
33
34
35
final initial code trial.py - final initial code trial.py
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
final initial code trial.py
ATM
• Problems
A
A
A
A
A
A
else:
A
amount = float(input("Enter deposit amount: "))
self.accounts [debit_card] ["balance"]+= amount
print("Deposit successful. Current balance: $", self.accounts [debit_card] ["balance"])
elif choice == "2":
amount = float(input("Enter withdraw amount: "))
if amount <= self.accounts [debit_card] ["balance"]:
self.accounts [debit_card] ["balance"] = amount
print("Withdraw successful. Current balance : $", self.accounts [debit_card]["balance"])
else:
break
else:
elif choice == "3":"
print("Current balance: $", self.accounts [debit_card] [ "balance"])
elif choice == "4":
create account()
> Terminal
print("Logged out.")
else:
atm = ATM()
while True:
print("1. Create account\n2. Login\n3. Exit")
choice = input("Enter your choice: ")
if choice == "1":
print("Insufficient balance.")
break
print("Invalid debit card pin.")
atm.create_account()
elif choice == "2":
print("Invalid choice.")
atm.login()
elif choice == "3":
Services
print("Exiting...")
print("Invalid choice.")
8
Current File ▾
8:47
$
G
ENG
Q
A 2
x
⠀
Notifications
CRLF UTF-8 4 spaces Python 3.11
9:31 PM
2/19/2023
Q Search
56°
i
H
36
99+
prime
video
CORSAIR
NGERNOBLED
XXXXX
20
666.6
B
V
0094
| ()▬▬
ENDE
CORSAIR
PC
Sese
9:31 PM
2/19/2023](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6efda218-3274-4861-a930-554e0197f95c%2F373095fb-dbea-4c6a-9ebf-13129630b30c%2Fo38qx1x_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 2 images

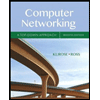
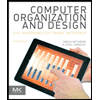
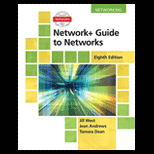
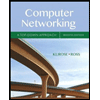
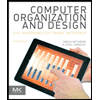
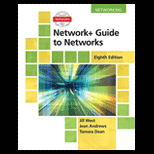
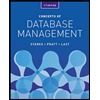
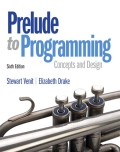
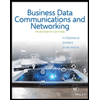