@lest public void test_0_20) { Toy t1 = new Toy(1000123, "Colouring Book", 3, 9.99); +1.setToyID(1000854); t1.setToyName("Teddy Bear"); t1.setToyQuantity(4); 11.setToyPrice(19.80); int toyID=t1.getToyID(); String toyName = t1.getToyName(); int toyQty = t1.getToyQuantity(); double toyPrice = t1.getToyPrice(); String toyInfo = t1.getToy Information(); int expToyID=1000854; int expToyQty = 4; double expToyPrice = 19.8; String expToyName = "Teddy Bear"; String expToyInfo = "Toy(1000854, Teddy Bear), quantity(4) with $( 19.80)/toy"; String errorMsg1 = String.format("\n Test set ToyID failed. Returned (%d) " + "but correct is (%d)", toyID, expToyID); assertEquals(errorMsg1, expToyID, toyID); String errorMsg2 = String.format("\n Test set ToyName failed. Returned (%s) " + "but correct is (%s)", toyName, correct is (%d)". expToyName); assertEquals(errorMsg2, expToyName, toyName); String errorMsg3 = String.format("\n Test set ToyQuantity failed. Returned (%d) " + "but toyQty, expToyQty); assertEquals(errorMsg3, expToyQty, toyQty); String errorMsg4 = String.format("\n Test set ToyPrice failed. Returned (%6.2f) " + "but correct is (%6.21)", toyPrice, expToy Price); final double THRESHOLD = .01; assertTrue(errorMsg4, Math.abs(expToyPrice-toyPrice) < THRESHOLD); String errorMsg5 = String.format("\n Test get ToyInformation failed. Returned (%s)" + "but correct is (%s)", toyInfo, expToy Info); assertEquals(errorMsg5, expToyInfo, toyInfo); } public void test_0_1() { Toy t1 = new Toy(1000123, "Colouring Book", 3, 9.99); t1.setToyID(1000127); t1.setToyName("SpongeBob DVD"); t1.setToyQuantity(8); t1.setToyPrice(29.98); int toyID = t1.getToyID(); String toyName = t1.getToyName(); int toyQty = t1.getToyQuantity(); double toyPrice = t1.getToyPrice(); String toyInfo = t1.getToy Information(); int expToyID = 1000127; int expToyQty = 8; double expToyPrice = 29.98; String expToyName = "SpongeBob DVD"; String expToyInfo = "Toy(1000127, SpongeBob DVD), quantity(8) with $( 29.98)/toy"; String errorMsg1 = String.format("\n Test set ToyID failed. Returned (%d) " + "but correct is (%d)", toyID, expToyID); assertEquals(errorMsg1, expToyID, toyID); String errorMsg2 = String.format("\n Test setToyName failed. Returned (%s) " + "but correct is (%s)", toyName, correct is (%d)", expToyName); assertEquals(errorMsg2, expToyName, toyName); String errorMsg3 = String.format("\n Test set ToyQuantity failed. Returned (%d) " + "but toyQty, expToyQty); assertEquals(errorMsg3, expToyQty, toyQty); String errorMsg4 = String.format("\n Test set Toy Price failed. Returned (%6.2f) " + "but correct is (%6.2f)", toyPrice, expToy Price); final double THRESHOLD = .01; assertTrue(errorMsg4, Math.abs(expToy Price - toyPrice) < THRESHOLD); String errorMsg5 = String.format("\n Test getToyInformation failed. Returned (%s) " + "but correct is (%s)", toyInfo, expToyInfo); assertEquals(errorMsg5, expToy Info, toyInfo); }
Please help me with this. I am having trouble. Create a java that corresponds / follows the test cases:
public void test_0_0() {
Toyt1=newToy(1000121,"Red Bike",1,89.99);
inttoyID=t1.getToyID();
StringtoyName=t1.getToyName();
inttoyQty=t1.getToyQuantity();
doubletoyPrice=t1.getToyPrice();
StringtoyInfo=t1.getToyInformation();
intexpToyID=1000121;
intexpToyQty=1;
doubleexpToyPrice=89.99;
StringexpToyName="Red Bike";
StringexpToyInfo="Toy(1000121,Red Bike), quantity(1) with $( 89.99)/toy";
StringerrorMsg1=String.format("\n Test getToyID failed. Returned (%d) " + "but correct is (%d)", toyID,
expToyID);
assertEquals(errorMsg1, expToyID, toyID);
StringerrorMsg2=String.format("\n Test getToyName failed. Returned (%s) " + "but correct is (%s)", toyName,
expToyName);
assertEquals(errorMsg2, expToyName, toyName);
StringerrorMsg3=String.format("\n Test getToyQuantity failed. Returned (%d) " + "but correct is (%d)",
toyQty,expToyQty);
assertEquals(errorMsg3, expToyQty, toyQty);
StringerrorMsg4=String.format("\n Test getToyPrice failed. Returned (%6.2f) " + "but correct is (%6.2f)",
toyPrice,expToyPrice);
finaldoubleTHRESHOLD=.01;
assertTrue(errorMsg4, Math.abs(expToyPrice - toyPrice) < THRESHOLD);
StringerrorMsg5=String.format("\n Test getToyInformation failed. Returned (%s) " + "but correct is (%s)",
toyInfo,expToyInfo);
assertEquals(errorMsg5, expToyInfo, toyInfo);
}
***The other two test cases are in the images below**
Thank you


Unlock instant AI solutions
Tap the button
to generate a solution
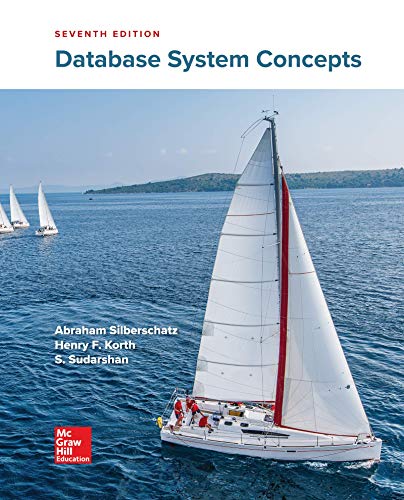
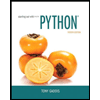
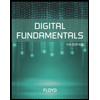
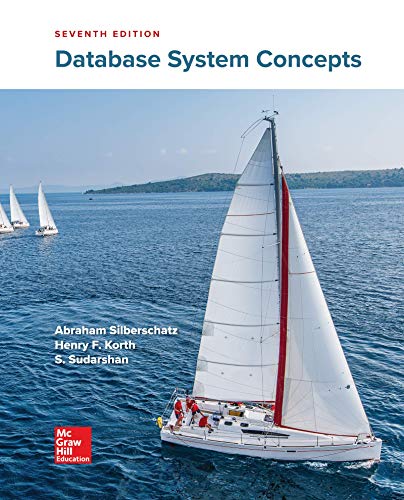
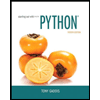
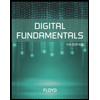
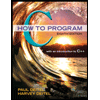
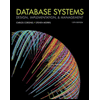
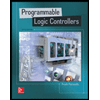