For this task, save your work in Roman.java You surely have encountered Roman numerals: I, II, III, XXII, MCMXLVI, etc. Roman numerals are represented by repeating and combing the following seven characters: I = 1, V = 5, X = 10, L = 50, C = 100, D = 500, M = 1000. To understand how they must be composed, we borrow the following excerpt from Dive Into Python: • Characters are additive. I is 1, II is 2, and III is 3. VI is 6 ("5 and 1"), VII is 7, and VIII is 8. ⚫ The tens characters (I, X, C, and M) can be repeated up to three times. At 4, you need to subtract from the next highest fives character. You can't represent 4 as IIII; instead, it is represented as IV ("1 less than 5"). The number 40 is written as XL (10 less than 50), 41 as XLI, 42 as XLII, 43 as XLIII, and then 44 as XLIV (10 less than 50, then 1 less than 5). • Similarly, at 9, you need to subtract from the next highest tens character: 8 is VIII, but 9 is IX (1 less than 10), not VIIII (since the I character cannot be repeated four times). The number 90 is XC, 900 is CM. • The fives characters cannot be repeated. The number 10 is always represented as X, never as VV. The number 100 is always C, never LL. • Roman numerals are always written highest to lowest, and read left to right, so the order the of characters matters very much. DC is 600; CD is a completely different number (400, 100 less than 500). CI is 101; IC is not a valid Roman numeral (because you can't subtract 1 directly from 100; you would need to write it as XCIX, for 10 less than 100, then 1 less than 10). Your Task: You will write a public class Roman. Inside it, implement a function public static int romanToInt(String romanNum) that takes a string that is a number represented using Roman numerals and returns the number, as an integer, equals in value to the input. For example: romanToInt("I")==1 ⚫ romanToInt("V")==5 ⚫ romanToInt("VII")==7 ⚫ romanToInt("MCMLIV")==1954 ⚫ romanToInt("MCMXC")==1990 Implementation Tips: Instead of a series of if-else constructs, you might wish to learn about the switch-case construct. This may simplify your life quite a bit. For this problem, you don't need a Map, HashMap, or anything like that.
For this task, save your work in Roman.java You surely have encountered Roman numerals: I, II, III, XXII, MCMXLVI, etc. Roman numerals are represented by repeating and combing the following seven characters: I = 1, V = 5, X = 10, L = 50, C = 100, D = 500, M = 1000. To understand how they must be composed, we borrow the following excerpt from Dive Into Python: • Characters are additive. I is 1, II is 2, and III is 3. VI is 6 ("5 and 1"), VII is 7, and VIII is 8. ⚫ The tens characters (I, X, C, and M) can be repeated up to three times. At 4, you need to subtract from the next highest fives character. You can't represent 4 as IIII; instead, it is represented as IV ("1 less than 5"). The number 40 is written as XL (10 less than 50), 41 as XLI, 42 as XLII, 43 as XLIII, and then 44 as XLIV (10 less than 50, then 1 less than 5). • Similarly, at 9, you need to subtract from the next highest tens character: 8 is VIII, but 9 is IX (1 less than 10), not VIIII (since the I character cannot be repeated four times). The number 90 is XC, 900 is CM. • The fives characters cannot be repeated. The number 10 is always represented as X, never as VV. The number 100 is always C, never LL. • Roman numerals are always written highest to lowest, and read left to right, so the order the of characters matters very much. DC is 600; CD is a completely different number (400, 100 less than 500). CI is 101; IC is not a valid Roman numeral (because you can't subtract 1 directly from 100; you would need to write it as XCIX, for 10 less than 100, then 1 less than 10). Your Task: You will write a public class Roman. Inside it, implement a function public static int romanToInt(String romanNum) that takes a string that is a number represented using Roman numerals and returns the number, as an integer, equals in value to the input. For example: romanToInt("I")==1 ⚫ romanToInt("V")==5 ⚫ romanToInt("VII")==7 ⚫ romanToInt("MCMLIV")==1954 ⚫ romanToInt("MCMXC")==1990 Implementation Tips: Instead of a series of if-else constructs, you might wish to learn about the switch-case construct. This may simplify your life quite a bit. For this problem, you don't need a Map, HashMap, or anything like that.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:For this task, save your work in Roman.java
You surely have encountered Roman numerals: I, II, III, XXII, MCMXLVI, etc. Roman numerals
are represented by repeating and combing the following seven characters: I = 1, V = 5, X = 10, L = 50,
C = 100, D = 500, M = 1000. To understand how they must be composed, we borrow the following excerpt
from Dive Into Python:
• Characters are additive. I is 1, II is 2, and III is 3. VI is 6 ("5 and 1"), VII is 7, and VIII is 8.
⚫ The tens characters (I, X, C, and M) can be repeated up to three times. At 4, you need to subtract
from the next highest fives character. You can't represent 4 as IIII; instead, it is represented as
IV ("1 less than 5"). The number 40 is written as XL (10 less than 50), 41 as XLI, 42 as XLII, 43 as
XLIII, and then 44 as XLIV (10 less than 50, then 1 less than 5).
• Similarly, at 9, you need to subtract from the next highest tens character: 8 is VIII, but 9 is IX (1
less than 10), not VIIII (since the I character cannot be repeated four times). The number 90 is
XC, 900 is CM.
• The fives characters cannot be repeated. The number 10 is always represented as X, never as VV.
The number 100 is always C, never LL.
• Roman numerals are always written highest to lowest, and read left to right, so the order the of
characters matters very much. DC is 600; CD is a completely different number (400, 100 less than
500). CI is 101; IC is not a valid Roman numeral (because you can't subtract 1 directly from 100;
you would need to write it as XCIX, for 10 less than 100, then 1 less than 10).
Your Task: You will write a public class Roman. Inside it, implement a function
public static int romanToInt(String romanNum)
that takes a string that is a number represented using Roman numerals and returns the number, as an
integer, equals in value to the input. For example:
romanToInt("I")==1
⚫ romanToInt("V")==5
⚫ romanToInt("VII")==7
⚫ romanToInt("MCMLIV")==1954
⚫ romanToInt("MCMXC")==1990
Implementation Tips: Instead of a series of if-else constructs, you might wish to learn about the
switch-case construct. This may simplify your life quite a bit.
For this problem, you don't need a Map, HashMap, or anything like that.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 2 images

Recommended textbooks for you
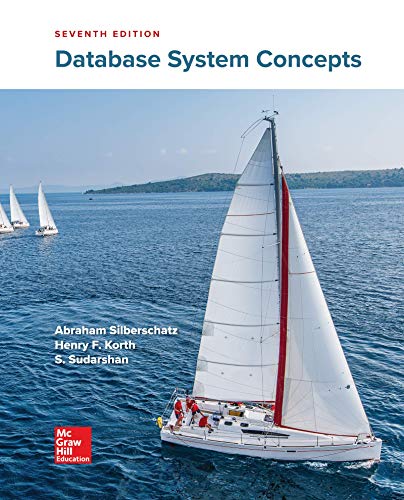
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
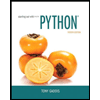
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
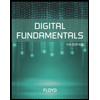
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
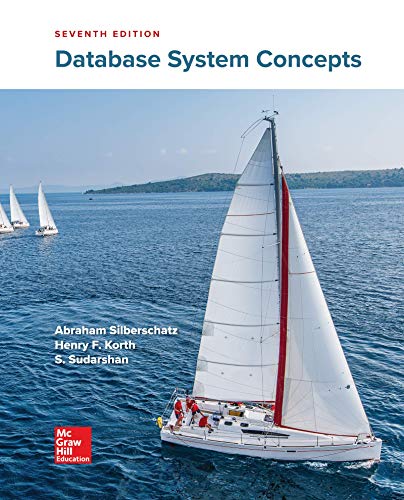
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
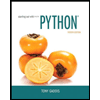
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
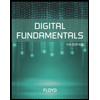
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
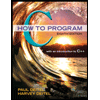
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
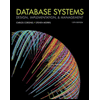
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
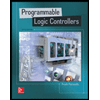
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education