public class YahtzeeP1 { final static int SCORE_NO_VALUE = -1; static int aces = SCORE_NO_VALUE; static int twos = SCORE_NO_VALUE; static int threes = SCORE_NO_VALUE; static int fours = SCORE_NO_V
Hello! I am having trouble trying to write out the code that is supposed to begin by creating the "framework" of my Yahtzee Java program and I am just not sure on how to go about it. There are instructions (//***) at the very end of the code on what I have to write out, but that is where I am having trouble. Can you also write the comments that explains the "why" and "how" for each code as well? Thanks and I appreciate it. Here is what I have so far:
public class YahtzeeP1 {
final static int SCORE_NO_VALUE = -1;
static int aces = SCORE_NO_VALUE;
static int twos = SCORE_NO_VALUE;
static int threes = SCORE_NO_VALUE;
static int fours = SCORE_NO_VALUE;
static int fives = SCORE_NO_VALUE;
static int sixes = SCORE_NO_VALUE;
static int threeKind = SCORE_NO_VALUE;
static int fourKind = SCORE_NO_VALUE;
static int fullHouse = SCORE_NO_VALUE;
static int smallStraight = SCORE_NO_VALUE;
static int largeStraight = SCORE_NO_VALUE;
static int yahtzee = SCORE_NO_VALUE;
static int chance = SCORE_NO_VALUE;
static int yahtzeeBonusCount = SCORE_NO_VALUE;
final static int YAHTZEE_SCORE = 50;
final static int YAHTZEE_BONUS_SCORE = 100;
final static String BORDER_CHAR = "*";
final static String INVALID_INPUT_MESSAGE = "*** Invalid input ***";
final static int NUMBER_OF_CATEGORIES = 13;
static int die1;
static int die2;
static int die3;
static int die4;
static int die5;
static int turnCount = 0;
static int numberOfRolls = 0;
public static void main(String[] args) {
final int MAX_NUMBER_ON_DIE = 6;
final int MAX_NUMBER_ROLLS = 3;
final String WELCOME_MESSAGE = "Welcome to YAHTZEE";
final String PRESS_ENTER_MESSAGE = "Press the Enter key to continue: ";
final String REROLL_MESSAGE_1 = "Enter: S for ScoreCard; D for Dice; X to Exit";
final String REROLL_MESSAGE_2 = "Or: A series of numbers to re-roll dice as follows:";
final String REROLL_MESSAGE_3 = "\t\tYou may re-roll any of the dice by entering " +
"the die #s without spaces.";
final String REROLL_MESSAGE_4 = "\t\tFor example, to re-roll dice #1, #3 & #4, " +
"enter 134 or enter 0 for none.";
final String REROLL_MESSAGE_5 = "You have %d roll(s) left this turn.";
final String REROLL_MESSAGE_6 = "Which of the dice would you like to roll again? ";
final String CATEGORY_MESSAGE_1 = "Enter: 1-" + NUMBER_OF_CATEGORIES +
"for category; S for ScoreCard; D for Dice; X to Exit";
final String CATEGORY_MESSAGE_2 = "Which category would you like to choose? ";
boolean turnOver = false;
boolean gameExit = false;
boolean gameComplete = false;
String dice2Reroll;
char die2Reroll;
String scoreOptionInput;
int scoreOption = 0;
Scanner input = new Scanner(System.in);
long seed = (new java.util.Date()).getTime();
Random generator = new Random(seed);
System.out.println();
System.out.println(WELCOME_MESSAGE);
do {
System.out.println();
System.out.print(PRESS_ENTER_MESSAGE);
input.nextLine();
turnCount++;
die1 = generator.nextInt(MAX_NUMBER_ON_DIE) + 1;
die2 = generator.nextInt(MAX_NUMBER_ON_DIE) + 1;
die3 = generator.nextInt(MAX_NUMBER_ON_DIE) + 1;
die4 = generator.nextInt(MAX_NUMBER_ON_DIE) + 1;
die5 = generator.nextInt(MAX_NUMBER_ON_DIE) + 1;
numberOfRolls = 1;
displayDice();
turnOver = false;
do {
System.out.println();
System.out.println(REROLL_MESSAGE_1);
System.out.println();
System.out.println(REROLL_MESSAGE_2);
System.out.println(REROLL_MESSAGE_3);
System.out.println(REROLL_MESSAGE_4);
System.out.println();
System.out.printf(REROLL_MESSAGE_5, (MAX_NUMBER_ROLLS - numberOfRolls));
System.out.println();
System.out.println();
System.out.print(REROLL_MESSAGE_6);
dice2Reroll = input.nextLine().trim();
//*** INSTRUCTIONS FOR CODE FOR YOU TO WRITE (Included within the uploaded image)


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

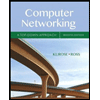
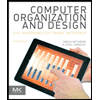
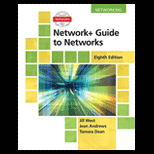
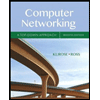
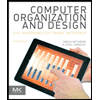
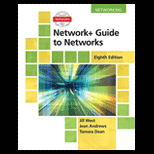
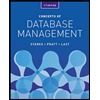
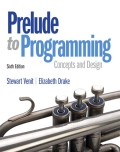
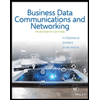