public class Main static int maxHeads (int[] birds, int m) { return 0; } } Run Debug public static void main(String[] args) { int arri] = {20, 1, 15); int arr21] (2, 1, 7, 4, 2); int arr3] (4, 10, 6, 7, 3, 1); System.out.println("Test 1, Total Heads 20 = " + maxHeads (arr1, m: 1)); //m = 1, bird 1 cut 20 heads, arrl is now (10,1,15) System.out.println("Test 2, Total Heads 35= " + maxHeads (arr1, m: 2)); //m2, bird 3 cut 15 head, arr1 is now (10,1,7) System.out.println("Test 3, Total Heads 45= " + maxHeads (arr1, m: 3)); //n = 3, bird 1 cut 10 heads, arrl is now (5,1, 7) System.out.println("Test 4, Total Heads 7 = " + maxHeads (arr2, m: 1)); // = 1 System.out.println("Test 5, Total Heads 11 = " + maxHeads (arr2, m: 2)); //m = 2 System.out.println("Test 6, Total Heads 14 = " + maxHeads (arr2, m: 3)); //m = 3 System.out.println("Test 7, Total Heads 10 = " + maxHeads (arr3, m: 1)); //n = 1 System.out.println("Test 8, Total Heads 17=" + maxHeads (arr3, m: 2)); // 2 System.out.println("Test 9, Total Heads 23= " + maxHeads (arr3, m: 3)); //n = 3 System.out.println("Test 10, Total Heads 28 = " + maxHeads (arr3, m: 4));//m4 System.out.println("Test 11, Total Heads 32= " + maxHeads (arr3, m: 5));//n = 5 System.out.println("Test 12, Total Heads 35 = " + maxHeads (arr3, m: 6));//n = 6 MS 2 OUTPUT DEBUG CONSOLE JUPYTER Question #12: Game Design You are creating a game where the hero, called Hercules, has to fight n angry and magical birds with multiple heads. The ith bird has birds[i] total number of heads. Assume it takes your hero 1 second to behead all heads of a specific bird, but after that magically this bird gets back half of its heads. If the bird had initially b heads, then after it is beheaded it grows floor(b/2) heads back. You have m seconds to cut as many heads as possible from the birds. a) Describe an efficient algorithm that finds the maximum number of heads cut by Hercules. Hint: start with the bird that has the maximum number of heads, etc. b) Write a java class that implements your algorithms (copy paste implementation). c) What is the data structure you used? Hint: Some options arrays, sorted arrays, linked lists, heaps etc. Choose the best. d) What is the running time of your algorithm? Ilgiven an array @birds of size n, and @m the available seconds return the //maximum number of head cut by the hero int maxStymphBirds(int[] birds, int m) Output A single integer, the maximum number of heads you can cut in m seconds. Example 1 n=3 m = 3 birds = [20, 1, 15] output = 45
public class Main static int maxHeads (int[] birds, int m) { return 0; } } Run Debug public static void main(String[] args) { int arri] = {20, 1, 15); int arr21] (2, 1, 7, 4, 2); int arr3] (4, 10, 6, 7, 3, 1); System.out.println("Test 1, Total Heads 20 = " + maxHeads (arr1, m: 1)); //m = 1, bird 1 cut 20 heads, arrl is now (10,1,15) System.out.println("Test 2, Total Heads 35= " + maxHeads (arr1, m: 2)); //m2, bird 3 cut 15 head, arr1 is now (10,1,7) System.out.println("Test 3, Total Heads 45= " + maxHeads (arr1, m: 3)); //n = 3, bird 1 cut 10 heads, arrl is now (5,1, 7) System.out.println("Test 4, Total Heads 7 = " + maxHeads (arr2, m: 1)); // = 1 System.out.println("Test 5, Total Heads 11 = " + maxHeads (arr2, m: 2)); //m = 2 System.out.println("Test 6, Total Heads 14 = " + maxHeads (arr2, m: 3)); //m = 3 System.out.println("Test 7, Total Heads 10 = " + maxHeads (arr3, m: 1)); //n = 1 System.out.println("Test 8, Total Heads 17=" + maxHeads (arr3, m: 2)); // 2 System.out.println("Test 9, Total Heads 23= " + maxHeads (arr3, m: 3)); //n = 3 System.out.println("Test 10, Total Heads 28 = " + maxHeads (arr3, m: 4));//m4 System.out.println("Test 11, Total Heads 32= " + maxHeads (arr3, m: 5));//n = 5 System.out.println("Test 12, Total Heads 35 = " + maxHeads (arr3, m: 6));//n = 6 MS 2 OUTPUT DEBUG CONSOLE JUPYTER Question #12: Game Design You are creating a game where the hero, called Hercules, has to fight n angry and magical birds with multiple heads. The ith bird has birds[i] total number of heads. Assume it takes your hero 1 second to behead all heads of a specific bird, but after that magically this bird gets back half of its heads. If the bird had initially b heads, then after it is beheaded it grows floor(b/2) heads back. You have m seconds to cut as many heads as possible from the birds. a) Describe an efficient algorithm that finds the maximum number of heads cut by Hercules. Hint: start with the bird that has the maximum number of heads, etc. b) Write a java class that implements your algorithms (copy paste implementation). c) What is the data structure you used? Hint: Some options arrays, sorted arrays, linked lists, heaps etc. Choose the best. d) What is the running time of your algorithm? Ilgiven an array @birds of size n, and @m the available seconds return the //maximum number of head cut by the hero int maxStymphBirds(int[] birds, int m) Output A single integer, the maximum number of heads you can cut in m seconds. Example 1 n=3 m = 3 birds = [20, 1, 15] output = 45
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
How would I implement the given function? Based on the given prompt?

Transcribed Image Text:### Game Design Problem: Cutting the Maximum Number of Heads
#### Scenario:
The scenario involves creating a game where the hero, called Hercules, fights birds with multiple heads. Each bird initially has a specified number of heads, and Hercules can cut them off. Once a head is cut, it reappears after a certain number of seconds, defined by a function `f(seconds)`.
#### Objective:
The objective is to maximize the number of heads Hercules can cut within `n` seconds.
#### Tasks:
1. **Algorithm Description:**
- Design an efficient algorithm that targets birds with the highest number of heads first.
2. **Java Class Implementation:**
- Implement a Java class that embodies the algorithm logic.
3. **Data Structures:**
- Consider using data structures like sorted arrays, linked lists, or heaps.
4. **Running Time Analysis:**
- Examine the algorithm's running time for optimization.
#### Code Explanation:
The Java code provided appears to be solving the problem using a method, `maxheads(birds, m)`, which calculates the maximum number of heads Hercules can cut off:
- **Arrays Representing Birds:**
- `arr1`, `arr2`, and `arr3` are arrays where each element represents the number of heads on a specific bird.
- **Method Invocation:**
- The method `maxheads` is called multiple times with different arrays and time limits to test and output results.
- **Output Interpretation:**
- For example, in "Test 1", Hercules cuts a total of 20 heads from `arr1` within 1 second.
#### Example Problem:
- Given `n = 3` and `m = 3`, with `birds = {20, 1, 15}`, the algorithm should output `45`, representing the maximum heads cut in the given time.
This setup demonstrates an understanding of priority decision-making and basic time-complexity considerations in algorithm design, useful in both game development and broader computational problem-solving contexts.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
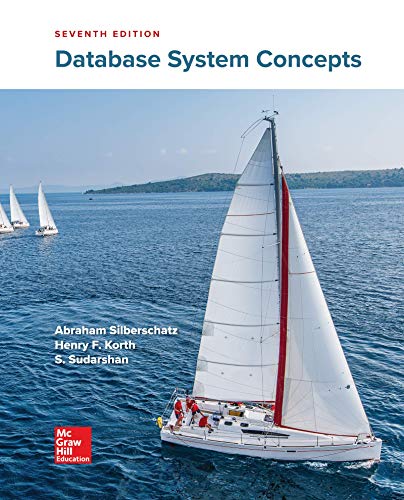
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
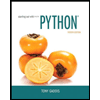
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
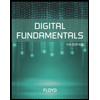
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
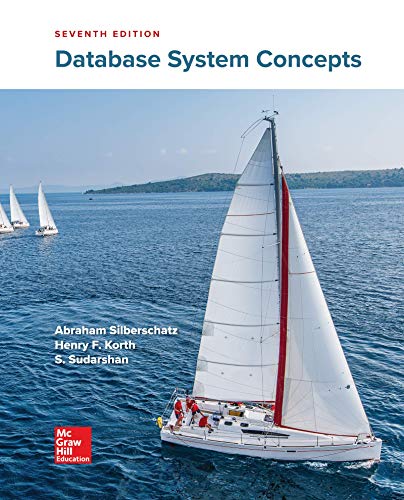
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
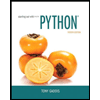
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
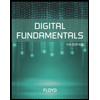
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
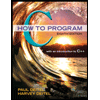
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
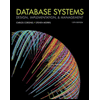
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
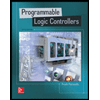
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education