backward. That is,, if the argument is 1234, it outputs the following to the screen: 4321.
Write a recursive void function that has one parameter that is a positive integer. When called, the function writes its argument to the screen backward. That is,, if the argument is 1234, it outputs the following to the screen: 4321.
I've literally tried to create this program and I have no idea how to make it work. Please help me. Is it possible to explain line by line what the code does?

#include <iostream>
using namespace std;
void reverse(int n) //function reverse
{
if (n < 10)
{
cout << n; //printing the number in reverse.
return;
}
else
{
cout << n % 10; //finding modulus of number
reverse(n/10); //dividing the number by 10 and calling recursively
}
}
int main()
{
cout<<"Enter the number : "; //printing for input
int n;
cin>>n; //taking input
reverse(n); //calling the function
return 0;
}
Step by step
Solved in 3 steps with 2 images

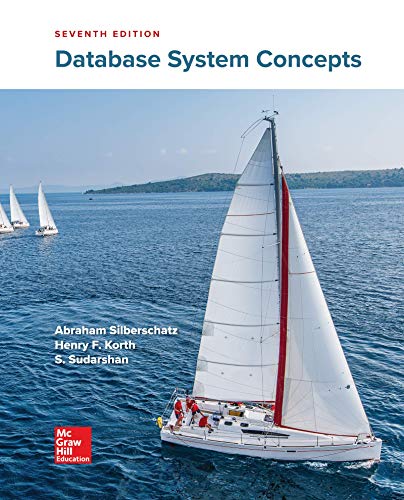
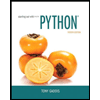
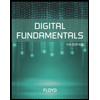
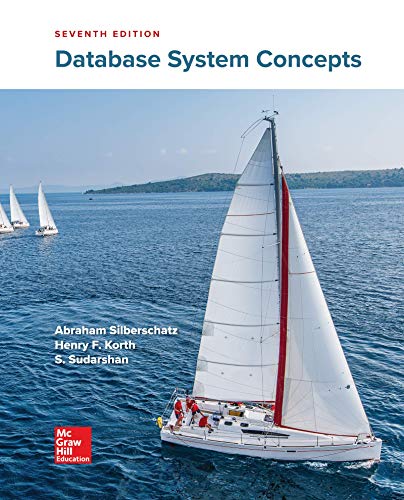
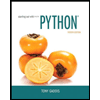
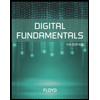
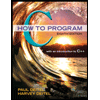
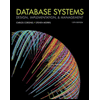
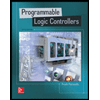