public class main { public static void main(String[] args) { Polygon[] shapes = new Polygon[3]; shapes[0] shapes[1] = new Triangle(17.0,12.0,9.0); shapes[2] = new Rectangle(6.0,2.0); = new Triangle(3.0,4.0,5.0); System.out.println("Shapes: "); for ( Polygon p : shapes ) { System.out.println(p); System.out.println("has area " + p.area() + " and perimeter + p. perimeter) ); System.out.println();
public class main { public static void main(String[] args) { Polygon[] shapes = new Polygon[3]; shapes[0] shapes[1] = new Triangle(17.0,12.0,9.0); shapes[2] = new Rectangle(6.0,2.0); = new Triangle(3.0,4.0,5.0); System.out.println("Shapes: "); for ( Polygon p : shapes ) { System.out.println(p); System.out.println("has area " + p.area() + " and perimeter + p. perimeter) ); System.out.println();
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![public class main {
public static void main(String[] args) {
Polygon[] shapes = new Polygon[3];
shapes[0]
shapes[1]
shapes[2]
= new Triangle(3.0,4.0,5.0);
new Triangle(17.0,12.0,9.0);
= new Rectangle(6.0,2.0);
System.out.println("Shapes: ");
for ( Polygon p : shapes ) {
System.out.println(p);
System.out.println("has area
10
+ p.area() +
+ p. perimeter() );
11
12
and perimeter
System.out.println();
}
}
13
14
15
16
}
Polygon Interface: Any class that inherits a Interface must implement
these methods.
DON'T MAKE ANY CHANGES IN THIS FILE
public interface Polygon {
/*
Calculates the area of the Polygon
*/
10
public double area();
11
12
/*
Calculates the perimeter of the Polygon
*/
public double perimeter();
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc7f33394-5a52-4a6c-8272-5682354fd51c%2F17a22c0c-f5df-4cb4-8dd9-2ad2568058ff%2Fje3akrc_processed.png&w=3840&q=75)
Transcribed Image Text:public class main {
public static void main(String[] args) {
Polygon[] shapes = new Polygon[3];
shapes[0]
shapes[1]
shapes[2]
= new Triangle(3.0,4.0,5.0);
new Triangle(17.0,12.0,9.0);
= new Rectangle(6.0,2.0);
System.out.println("Shapes: ");
for ( Polygon p : shapes ) {
System.out.println(p);
System.out.println("has area
10
+ p.area() +
+ p. perimeter() );
11
12
and perimeter
System.out.println();
}
}
13
14
15
16
}
Polygon Interface: Any class that inherits a Interface must implement
these methods.
DON'T MAKE ANY CHANGES IN THIS FILE
public interface Polygon {
/*
Calculates the area of the Polygon
*/
10
public double area();
11
12
/*
Calculates the perimeter of the Polygon
*/
public double perimeter();
}

Transcribed Image Text:Create a class called Rectangle that implements the Polygon interface
Must have a default constructor that sets:
double: width = 1
double: height = 1
Must have another Constructor that takes in:
double: width --> 1st argument
double: height --> 2nd argument
10
Must have setters and getters for width and height.
double getWidth()
double getHeight()
void setHeight()
11
12
13
14
15
void setWidth()
16
17
Must have a tostring (test Provided)
"String tostring()" should return the following:
"Rectangle width & height: " + width + ", ▪
18
20
height
e */
import java.lang. Math;
Create a class called Triangle that uses the Polygon interface;
Assume this is a right triangle
double a, b, c;
Must have a default constructor that sets:
a = 3
11
b = 4
12
C = 5
Must have a Constructor that takes in 3 variables:
a --> 1st argument
b --> 2nd argument
C --> 3rd argument
Must have setters and getters for all the variables
double getA()
void setA()
19
20
22
23
...
24
Must have a toString (test Provided)
"String tostring()" Should return the following:
"Triangle edge lengths: " + a + ", " +
25
26
27
28
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

Recommended textbooks for you
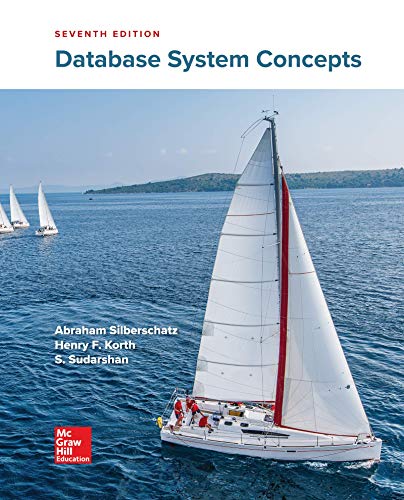
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
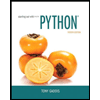
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
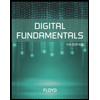
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
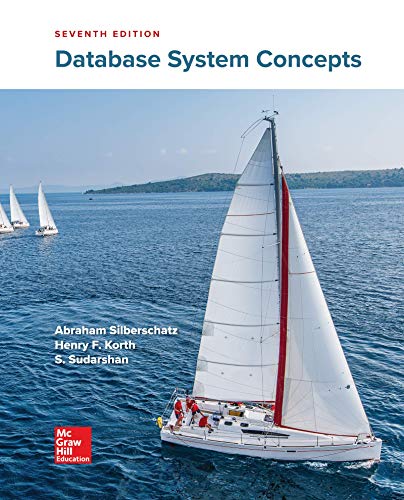
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
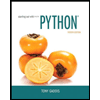
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
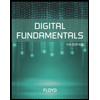
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
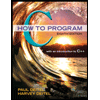
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
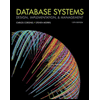
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
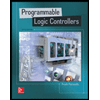
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education