Prompt Below is the definition for the Receipt class: public class Receipt { private String[] items; private double[] prices; private int itemCount; public Receipt() { items = new String[100]; prices = new double[100]; itemCount = 0; } public Receipt(int length) { items = new String[length]; prices = new double[length]; itemCount = 0; } public boolean addItem(String itemName, double itemPrice) { if (itemCount == items.length) return false; items[itemCount] = itemName; prices[itemCount] = itemPrice; itemCount++; return true; } public void printReceipt() { double total = 0; for (int i = 0; i < itemCount; i++) { System.out.printf("%.2f %s\n", prices[i], items[i]); total += prices[i]; } System.out.printf("\n%.2f Total Sale\n", total); } } what does the Receipt class do? (Look at its methods - what is it capable of doing?) what is the purpose of the itemCount variable? In Java, the length of an array cannot be changed once it is created. If you want to extend the length of an array, you have to create a new, longer array and copy the old array's contents into it. Create a new method in the Receipt class called extendReceipt(int number) which uses this process to "extend" the receipt's arrays by the argument number. Your post only needs to show the one method
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Prompt
Below is the definition for the Receipt class:
public class Receipt {
private String[] items;
private double[] prices;
private int itemCount;
public Receipt() {
items = new String[100];
prices = new double[100];
itemCount = 0;
}
public Receipt(int length) {
items = new String[length];
prices = new double[length];
itemCount = 0;
}
public boolean addItem(String itemName, double itemPrice) {
if (itemCount == items.length) return false;
items[itemCount] = itemName;
prices[itemCount] = itemPrice;
itemCount++;
return true;
}
public void printReceipt() {
double total = 0;
for (int i = 0; i < itemCount; i++) {
System.out.printf("%.2f %s\n", prices[i], items[i]);
total += prices[i];
}
System.out.printf("\n%.2f Total Sale\n", total);
}
}
- what does the Receipt class do? (Look at its methods - what is it capable of doing?)
- what is the purpose of the itemCount variable?
- In Java, the length of an array cannot be changed once it is created. If you want to extend the length of an array, you have to create a new, longer array and copy the old array's contents into it. Create a new method in the Receipt class called extendReceipt(int number) which uses this process to "extend" the receipt's arrays by the argument number. Your post only needs to show the one method

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

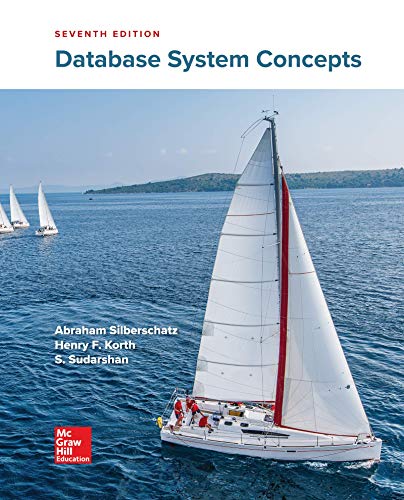
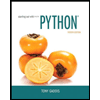
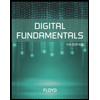
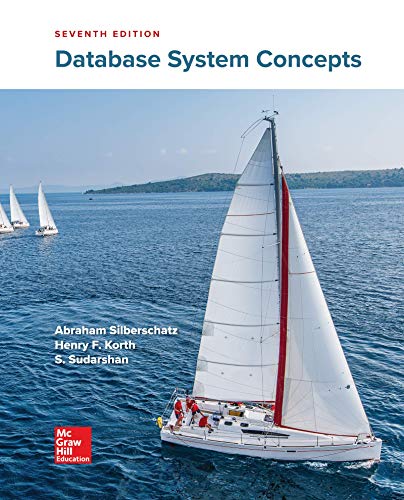
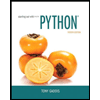
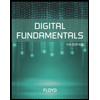
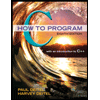
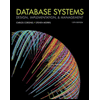
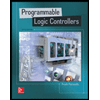