Programming Exercise: Using Java, complete the CodeWriter class (part of the overall compiler) which has a single public method (you may have several private helper methods) that takes in a String expression and outputs the corresponding pseudo VM code (to the console), according to the algorithm below: Page source code codewrite(exp): if exp is a number n: output "push n" stack-machine code push x x + g(2,y,-z) * 5 if exp is a variable var: output "push var" resulting code push 2 push y push z neg if exp is "exp, op exp": codewrite(exp₁). codewrite (exp₂), output "op" if exp is "op exp": codeWrite(exp), output "op" if exp is "f (exp, exp2, ...)": codeWrite(exp₁), codewrite(exp). output "call" call g push 5 You may use the following API design to help guide your work, or choose to implement your own design. Note, since the class is named CodeWriter, I changed the method name to writeCode (instead of codeWrite, as it is confusing otherwise). CodeWriter Class Fields a exp: string Methods CodeWriter(string exp) 0 main(string[] args): void writeCode(string exp): void Finally, here are several test expressions you can use to verify the output of your CodeWriter. String testExp1 = "x + -y - z + 5"; String testExp2 = "f(x, y) * z + 2"; > 1 of 2 --------- - ZOOM +
Programming Exercise: Using Java, complete the CodeWriter class (part of the overall compiler) which has a single public method (you may have several private helper methods) that takes in a String expression and outputs the corresponding pseudo VM code (to the console), according to the algorithm below: Page source code codewrite(exp): if exp is a number n: output "push n" stack-machine code push x x + g(2,y,-z) * 5 if exp is a variable var: output "push var" resulting code push 2 push y push z neg if exp is "exp, op exp": codewrite(exp₁). codewrite (exp₂), output "op" if exp is "op exp": codeWrite(exp), output "op" if exp is "f (exp, exp2, ...)": codeWrite(exp₁), codewrite(exp). output "call" call g push 5 You may use the following API design to help guide your work, or choose to implement your own design. Note, since the class is named CodeWriter, I changed the method name to writeCode (instead of codeWrite, as it is confusing otherwise). CodeWriter Class Fields a exp: string Methods CodeWriter(string exp) 0 main(string[] args): void writeCode(string exp): void Finally, here are several test expressions you can use to verify the output of your CodeWriter. String testExp1 = "x + -y - z + 5"; String testExp2 = "f(x, y) * z + 2"; > 1 of 2 --------- - ZOOM +
Chapter2: Using Data
Section: Chapter Questions
Problem 14RQ
Related questions
Question
Please help me do this exercise
![Programming Exercise:
Using Java, complete the CodeWriter class (part of the overall compiler) which has a
single public method (you may have several private helper methods) that takes in a
String expression and outputs the corresponding pseudo VM code (to the console),
according to the algorithm below:
Page
source code
codewrite(exp):
if exp is a number n:
output "push n"
stack-machine code
push x
x + g(2,y,-z) * 5
if exp is a variable var:
output "push var"
resulting
code
push 2
push y
push z
neg
if exp is "exp, op exp":
codewrite(exp₁).
codewrite (exp₂),
output "op"
if exp is "op exp":
codeWrite(exp),
output "op"
if exp is "f (exp, exp2, ...)":
codeWrite(exp₁),
codewrite(exp).
output "call"
call g
push 5
You may use the following API design to help guide your work, or choose to implement
your own design. Note, since the class is named CodeWriter, I changed the method
name to writeCode (instead of codeWrite, as it is confusing otherwise).
CodeWriter
Class
Fields
a exp: string
Methods
CodeWriter(string exp)
0 main(string[] args): void
writeCode(string exp): void
Finally, here are several test expressions you can use to verify the output of your
CodeWriter.
String testExp1 = "x + -y - z + 5";
String testExp2
=
"f(x, y) * z + 2";
>
1
of 2
---------
- ZOOM
+](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F46c0fb16-724b-4726-818c-9156075ac6cc%2Fa79645dc-4bee-4fc0-bf76-deb63c78e071%2Fehl84o_processed.png&w=3840&q=75)
Transcribed Image Text:Programming Exercise:
Using Java, complete the CodeWriter class (part of the overall compiler) which has a
single public method (you may have several private helper methods) that takes in a
String expression and outputs the corresponding pseudo VM code (to the console),
according to the algorithm below:
Page
source code
codewrite(exp):
if exp is a number n:
output "push n"
stack-machine code
push x
x + g(2,y,-z) * 5
if exp is a variable var:
output "push var"
resulting
code
push 2
push y
push z
neg
if exp is "exp, op exp":
codewrite(exp₁).
codewrite (exp₂),
output "op"
if exp is "op exp":
codeWrite(exp),
output "op"
if exp is "f (exp, exp2, ...)":
codeWrite(exp₁),
codewrite(exp).
output "call"
call g
push 5
You may use the following API design to help guide your work, or choose to implement
your own design. Note, since the class is named CodeWriter, I changed the method
name to writeCode (instead of codeWrite, as it is confusing otherwise).
CodeWriter
Class
Fields
a exp: string
Methods
CodeWriter(string exp)
0 main(string[] args): void
writeCode(string exp): void
Finally, here are several test expressions you can use to verify the output of your
CodeWriter.
String testExp1 = "x + -y - z + 5";
String testExp2
=
"f(x, y) * z + 2";
>
1
of 2
---------
- ZOOM
+
AI-Generated Solution
Unlock instant AI solutions
Tap the button
to generate a solution
Recommended textbooks for you
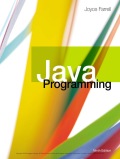
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
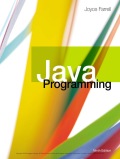
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT