Processing Grades The file Grades.java contains a program that reads in a sequence of student grades and computes the average grade, the number of students who pass (a grade of at least 60) and the number who fail. The program uses a loop (which you learn about in the next section). 1. Compile and run the program to see how it works. 2. Study the code and do the following. O Replace the statement that finds the sum of the grades with one that uses the += operator. O Replace each of three statements that increment a counting variable with statements using the increment operator. 3. Run your program to make sure it works. Now replace the "if" statement that updates the pass and fail counters with the conditional operator. 4. // // // // // // // import java.util.Scanner; Grades.java Read in a sequence of grades and compute the average grade, the number of passing grades (at least 60) and the number of failing grades. public class Grades { // // Reads in and processes grades until a negative number is entered. // public static void main (String[] args) { double grade; double sumofGrades; // a running total of the student grades int numStudents; //a count of the students int numPass; //a student's grade //a count of the number who pass // a count of the number who fail int numFail; Scanner scan = new Scanner (System.in); System.out.println ("\nGrade Processing Program\n"); // Initialize summing and counting variables sumofGrades = 0; numStudents = 0; numPass = 0; numFail = 0; // Read in the first grade System.out.print ("Enter the first student's grade: "); grade = scan.nextDouble(); while (grade >= 0) { sumofGrades = sumofGrades + grade; numStudents = numStudents + 13; if (grade < 60) numFail = numFail + 1; else numPass = numPass + 1;
Processing Grades The file Grades.java contains a program that reads in a sequence of student grades and computes the average grade, the number of students who pass (a grade of at least 60) and the number who fail. The program uses a loop (which you learn about in the next section). 1. Compile and run the program to see how it works. 2. Study the code and do the following. O Replace the statement that finds the sum of the grades with one that uses the += operator. O Replace each of three statements that increment a counting variable with statements using the increment operator. 3. Run your program to make sure it works. Now replace the "if" statement that updates the pass and fail counters with the conditional operator. 4. // // // // // // // import java.util.Scanner; Grades.java Read in a sequence of grades and compute the average grade, the number of passing grades (at least 60) and the number of failing grades. public class Grades { // // Reads in and processes grades until a negative number is entered. // public static void main (String[] args) { double grade; double sumofGrades; // a running total of the student grades int numStudents; //a count of the students int numPass; //a student's grade //a count of the number who pass // a count of the number who fail int numFail; Scanner scan = new Scanner (System.in); System.out.println ("\nGrade Processing Program\n"); // Initialize summing and counting variables sumofGrades = 0; numStudents = 0; numPass = 0; numFail = 0; // Read in the first grade System.out.print ("Enter the first student's grade: "); grade = scan.nextDouble(); while (grade >= 0) { sumofGrades = sumofGrades + grade; numStudents = numStudents + 13; if (grade < 60) numFail = numFail + 1; else numPass = numPass + 1;
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Topic Video
Question
![Processing Grades
The file Grades.java contains a program that reads in a sequence of student grades and computes the average grade, the
number of students who pass (a grade of at least 60) and the number who fail. The program uses a loop (which you learn
about in the next section).
1. Compile and run the program to see how it works.
2. Study the code and do the following.
O Replace the statement that finds the sum of the grades with one that uses the += operator.
O Replace each of three statements that increment a counting variable with statements using the increment operator.
Run your program to make sure it works.
Now replace the "if" statement that updates the pass and fail counters with the conditional operator.
3.
4.
// ************************************************************
//
Grades.java
//
//
//
//
Read in a sequence of grades and compute the average
grade, the number of passing grades (at least 60)
and the number of failing grades.
// ***************************** *******************************
import java.util.Scanner;
public class Grades
{
//
//
Reads in and processes grades until a negative number is entered.
//
public static void main (String[] args)
{
double grade;
double sumOfGrades; // a running total of the student grades
int numStudents; //a count of the students
int numPass;
//a student's grade
//a count of the number who pass
// a count of the number who fail
int numFail;
Scanner scan
= new Scanner (System.in);
System.out.println ("\nGrade Processing Program\n");
// Initialize summing and counting variables
sumofGrades
= 0;
numStudents = 0;
numPass = 0;
numFail = 0;
// Read in the first grade
System.out.print ("Enter the first student's grade: ");
grade = scan.nextDouble();
while (grade >= 0)
{
sumofGrades = sumOfGrades + grade;
numStudents = numStudents + 1;
if (grade < 60)
numFail = numFail + 1;
else
numPass = numPass
+ 1;
70
Chapter 6: More Conditionals and Loops
// Read the next grade
System.out.print ("Enter the next grade (a negative to quit): ");
grade = scan.nextDouble();
}
if (numStudents > 0)
{
System.out.println ("\nGrade Summary: ");
System.out.println ("Class Average:
System.out.println ("Number of Passing Grades:
System.out.println ("Number of Failing Grades:
}
+ sumOfGrades/numStudents);
" + numPass);
+ numFai1);
else
System.out.println ("No grades processed.");
}
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4a7e6ad5-0eb5-476a-8034-bd3421cbec45%2Fdc9515a0-de4a-4d9c-a2f2-a36a31214dc3%2Fm7cnh5_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Processing Grades
The file Grades.java contains a program that reads in a sequence of student grades and computes the average grade, the
number of students who pass (a grade of at least 60) and the number who fail. The program uses a loop (which you learn
about in the next section).
1. Compile and run the program to see how it works.
2. Study the code and do the following.
O Replace the statement that finds the sum of the grades with one that uses the += operator.
O Replace each of three statements that increment a counting variable with statements using the increment operator.
Run your program to make sure it works.
Now replace the "if" statement that updates the pass and fail counters with the conditional operator.
3.
4.
// ************************************************************
//
Grades.java
//
//
//
//
Read in a sequence of grades and compute the average
grade, the number of passing grades (at least 60)
and the number of failing grades.
// ***************************** *******************************
import java.util.Scanner;
public class Grades
{
//
//
Reads in and processes grades until a negative number is entered.
//
public static void main (String[] args)
{
double grade;
double sumOfGrades; // a running total of the student grades
int numStudents; //a count of the students
int numPass;
//a student's grade
//a count of the number who pass
// a count of the number who fail
int numFail;
Scanner scan
= new Scanner (System.in);
System.out.println ("\nGrade Processing Program\n");
// Initialize summing and counting variables
sumofGrades
= 0;
numStudents = 0;
numPass = 0;
numFail = 0;
// Read in the first grade
System.out.print ("Enter the first student's grade: ");
grade = scan.nextDouble();
while (grade >= 0)
{
sumofGrades = sumOfGrades + grade;
numStudents = numStudents + 1;
if (grade < 60)
numFail = numFail + 1;
else
numPass = numPass
+ 1;
70
Chapter 6: More Conditionals and Loops
// Read the next grade
System.out.print ("Enter the next grade (a negative to quit): ");
grade = scan.nextDouble();
}
if (numStudents > 0)
{
System.out.println ("\nGrade Summary: ");
System.out.println ("Class Average:
System.out.println ("Number of Passing Grades:
System.out.println ("Number of Failing Grades:
}
+ sumOfGrades/numStudents);
" + numPass);
+ numFai1);
else
System.out.println ("No grades processed.");
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
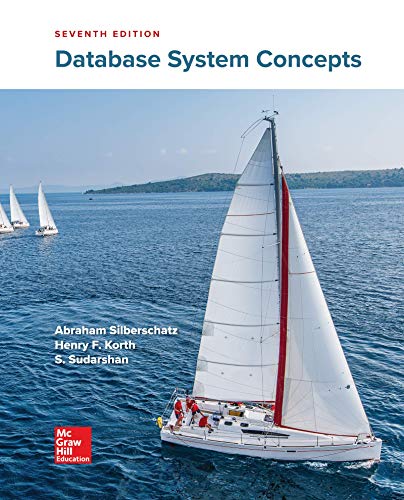
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
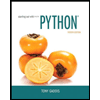
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
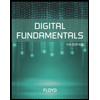
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
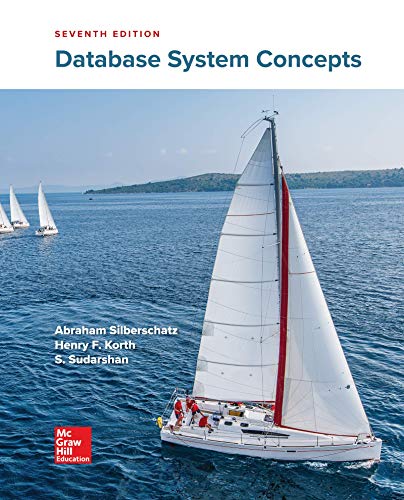
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
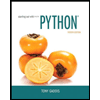
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
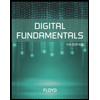
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
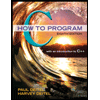
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
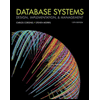
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
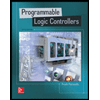
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education