Write a program named: WhileLessThan.java This program will prompt the user for an integer value greater than or equal to 10. Write a loop which will execute as long as the user inputs a value less than 10. After getting a valid input, print the value to the screen as shown. Enter an integer greater than or equal to 10: 3 Enter an integer greater than or equal to 10: 21 You entered: 21
Write a program named: WhileLessThan.java
This program will prompt the user for an integer value greater than or equal to 10.
Write a loop which will execute as long as the user inputs a value less than 10. After getting a valid input, print the value to the screen as shown.
Enter an integer greater than or equal to 10: 3
Enter an integer greater than or equal to 10: 21
You entered: 21
Another sample:
Enter an integer greater than or equal to 10: -2
Enter an integer greater than or equal to 10: 9
Enter an integer greater than or equal to 10: 10
You entered: 10

CODE:-
import java.util.Scanner;
public class WhileLessThan {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
int n;
do {
System.out.print("Enter an integer greater than or equal to 10: ");
n = in.nextInt();
} while (n < 10);
System.out.println("You entered: " + n);
}
}
Step by step
Solved in 2 steps with 1 images

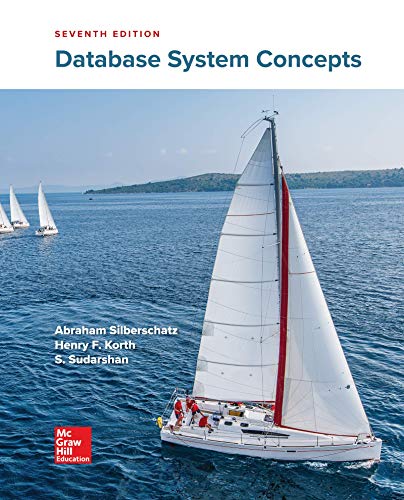
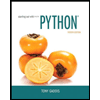
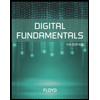
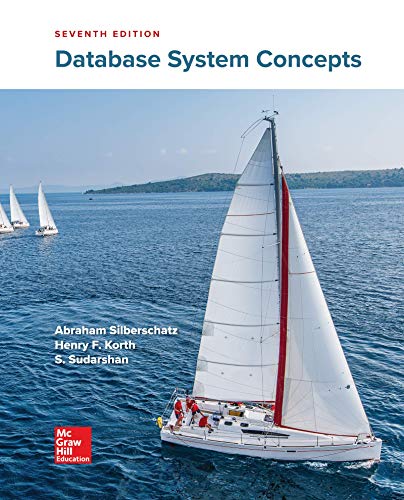
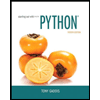
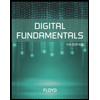
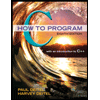
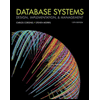
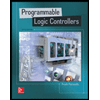