Answer in Java please
Answer in Java please


import java.util.Scanner;
// Create the class name of Twelve Days
public class TwelveDays
{
// Create a main method of the program
public static void main(String[] args)
{
final int MAX = 12;
int lastDay = 0; // last day for the song, user will update
Scanner scan = new Scanner(System.in);
//Get the last day and use input validation
//Begin 1st while
// Here starting with do while loop
// Because initially one-time user
//enter in the Christmas day
do {
//The user should ONLY be able to
//enter a day between 1 and 12.
System.out.print("How many days (1 to 12)? ");
lastDay = scan.nextInt();
}
//A while loop for input validation.
while (lastDay < 1 || lastDay > 12);
int day = 1; // loop control variable for song verses
//Begin 2nd while
// A second while loop to output the verses
while (day <= lastDay)
{
//Output: "On the" + day
//Output the suffix for the day
// (Day 1 should be 1st,
//day 2 should be 2nd,
//day 3 should be 3rd,
//and all other days should end with “th”. )
String string1="On the ";
String string2=" day of Christmas my true love gave to me";
String day1="st";
String day2="nd";
String day3="rd";
String otherdays="th";
// In this loop we use two switch statements.
//Begin 1st switch
//The first switch statement should add
//the appropriate suffix to the day.
switch (day)
{
case 1: {
System.out.println(string1+day+day1+string2);
break;
}
case 2: {
System.out.println(string1+day+day2+string2);
break;
}
case 3: {
System.out.println(string1+day+day3+string2);
break;
}
case 4: {
System.out.println(string1+day+otherdays+string2);
break;
} case 5: {
System.out.println(string1+day+otherdays+string2);
break;
} case 6: {
System.out.println(string1+day+otherdays+string2);
break;
} case 7: {
System.out.println(string1+day+otherdays+string2);
break;
} case 8: {
System.out.println(string1+day+otherdays+string2);
break;
} case 9: {
System.out.println(string1+day+otherdays+string2);
break;
} case 10: {
System.out.println(string1+day+otherdays+string2);
break;
} case 11: {
System.out.println(string1+day+otherdays+string2);
break;
} case 12: {
System.out.println(string1+day+otherdays+string2);
break;
}
}
//Output " day of Christmas my true love gave to me "
//Output the gift
//Begin 2nd switch
// The second switch statement should output
//the gift for the day.
switch (day)
{
case 12: {
System.out.println("Twelve drummers drumming,");
}
case 11: {
System.out.println("Eleven pipers piping,");
}
case 10: {
System.out.println("Ten lords a-leaping,");
}
case 9: {
System.out.println("Nine ladies dancing,");
}
case 8: {
System.out.println("Eight maids a-milking,");
}
case 7: {
System.out.println("Seven swans a-swimming,");
}
case 6: {
System.out.println("Six geese a-laying,");
}
case 5: {
System.out.println("Five golden rings,");
}
case 4: {
System.out.println("Four calling birds,");
}
case 3: {
System.out.println("Three French hens,");
}
case 2: {
System.out.println("Two turtle doves, and");
}
// don’t use the break statement in this one
// Using default
default: {
System.out.println("A partridge in a pear tree.\n\n");
}
}
// Increment the loop control variable
day++;
}
}
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

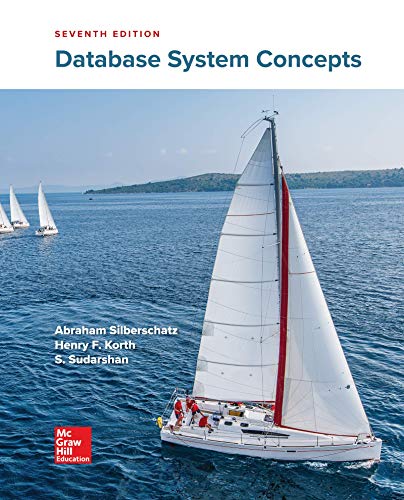
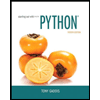
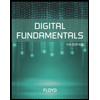
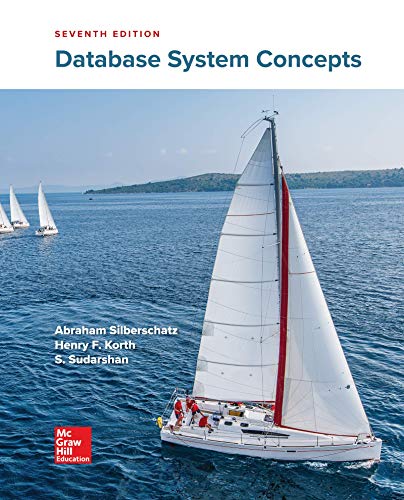
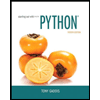
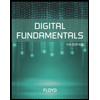
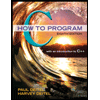
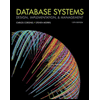
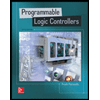