Problem I ( Assembler ) Provide the assembly implementation of the C - code below . Sub 10 is a function that subtract 10 from a given input x. Assumption : MyArray base address is store in register $S1. Feel free to use instruction li or si. li load an immediate value into a register . For instance, li $S4 5 will copy value 5 into register $S4. C code for ( i = 0,1 < 10 , i ++ ) { MyArray [ i ] = MyArray [ i - 1 ] + MyArray [ i + 1 ] ; Sub10 ( MyArray [ i ]; } Sub10 ( x ) { Return ( x - 10 ) ; } Code in Assembly Language: sub10(int): ; Implementation of the sub10() function push rbp mov rbp, rsp mov DWORD PTR [rbp-4], edi mov eax, DWORD PTR [rbp-4] sub eax, 10 pop rbp ret main: ; Main function Implementation push rbp mov rbp, rsp sub rsp, 64 mov DWORD PTR [rbp-4], 0 .L5: cmp DWORD PTR [rbp-4], 9 jg .L4 mov eax, DWORD PTR [rbp-4] lea edx, [rax+20] mov eax, DWORD PTR [rbp-4] cdqe mov DWORD PTR [rbp-64+rax*4], edx add DWORD PTR [rbp-4], 1 jmp .L5 .L4: mov DWORD PTR [rbp-8], 1 .L7: cmp DWORD PTR [rbp-8], 8 jg .L6 mov eax, DWORD PTR [rbp-8] sub eax, 1 cdqe mov edx, DWORD PTR [rbp-64+rax*4] mov eax, DWORD PTR [rbp-8] add eax, 1 cdqe mov eax, DWORD PTR [rbp-64+rax*4] add edx, eax mov eax, DWORD PTR [rbp-8] cdqe mov DWORD PTR [rbp-64+rax*4], edx mov eax, DWORD PTR [rbp-8] cdqe mov eax, DWORD PTR [rbp-64+rax*4] mov edi, eax call sub10(int) mov DWORD PTR [rbp-12], eax add DWORD PTR [rbp-8], 1 jmp .L7 .L6: mov eax, 0 leave ret __static_initialization_and_destruction_0(int, int): push rbp mov rbp, rsp sub rsp, 16 mov DWORD PTR [rbp-4], edi mov DWORD PTR [rbp-8], esi cmp DWORD PTR [rbp-4], 1 jne .L11 cmp DWORD PTR [rbp-8], 65535 jne .L11 mov edi, OFFSET FLAT:_ZStL8__ioinit call std::ios_base::Init::Init() [complete object constructor] mov edx, OFFSET FLAT:__dso_handle mov esi, OFFSET FLAT:_ZStL8__ioinit mov edi, OFFSET FLAT:_ZNSt8ios_base4InitD1Ev call __cxa_atexit .L11: nop leave ret _GLOBAL__sub_I_sub10(int): push rbp mov rbp, rsp mov esi, 65535 mov edi, 1 call __static_initialization_and_destruction_0(int, int) pop rbp ret Question is: Can you convert above assembly code to MIPS? This is
Problem I ( Assembler )
Provide the assembly implementation of the C - code below . Sub 10 is a function that subtract 10 from a given input x.
Assumption :
MyArray base address is store in register $S1.
Feel free to use instruction li or si.
li load an immediate value into a register . For instance, li $S4 5 will copy value 5 into register $S4.
C code
for ( i = 0,1 < 10 , i ++ )
{
MyArray [ i ] = MyArray [ i - 1 ] + MyArray [ i + 1 ] ;
Sub10 ( MyArray [ i ];
}
Sub10 ( x )
{
Return ( x - 10 ) ;
}
Code in Assembly Language:
sub10(int): ; Implementation of the sub10() function
push rbp
mov rbp, rsp
mov DWORD PTR [rbp-4], edi
mov eax, DWORD PTR [rbp-4]
sub eax, 10
pop rbp
ret
main: ; Main function Implementation
push rbp
mov rbp, rsp
sub rsp, 64
mov DWORD PTR [rbp-4], 0
.L5:
cmp DWORD PTR [rbp-4], 9
jg .L4
mov eax, DWORD PTR [rbp-4]
lea edx, [rax+20]
mov eax, DWORD PTR [rbp-4]
cdqe
mov DWORD PTR [rbp-64+rax*4], edx
add DWORD PTR [rbp-4], 1
jmp .L5
.L4:
mov DWORD PTR [rbp-8], 1
.L7:
cmp DWORD PTR [rbp-8], 8
jg .L6
mov eax, DWORD PTR [rbp-8]
sub eax, 1
cdqe
mov edx, DWORD PTR [rbp-64+rax*4]
mov eax, DWORD PTR [rbp-8]
add eax, 1
cdqe
mov eax, DWORD PTR [rbp-64+rax*4]
add edx, eax
mov eax, DWORD PTR [rbp-8]
cdqe
mov DWORD PTR [rbp-64+rax*4], edx
mov eax, DWORD PTR [rbp-8]
cdqe
mov eax, DWORD PTR [rbp-64+rax*4]
mov edi, eax
call sub10(int)
mov DWORD PTR [rbp-12], eax
add DWORD PTR [rbp-8], 1
jmp .L7
.L6:
mov eax, 0
leave
ret
__static_initialization_and_destruction_0(int, int):
push rbp
mov rbp, rsp
sub rsp, 16
mov DWORD PTR [rbp-4], edi
mov DWORD PTR [rbp-8], esi
cmp DWORD PTR [rbp-4], 1
jne .L11
cmp DWORD PTR [rbp-8], 65535
jne .L11
mov edi, OFFSET FLAT:_ZStL8__ioinit
call std::ios_base::Init::Init() [complete object constructor]
mov edx, OFFSET FLAT:__dso_handle
mov esi, OFFSET FLAT:_ZStL8__ioinit
mov edi, OFFSET FLAT:_ZNSt8ios_base4InitD1Ev
call __cxa_atexit
.L11:
nop
leave
ret
_GLOBAL__sub_I_sub10(int):
push rbp
mov rbp, rsp
mov esi, 65535
mov edi, 1
call __static_initialization_and_destruction_0(int, int)
pop rbp
ret
Question is:
Can you convert above assembly code to MIPS? This is the code for x86-64

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

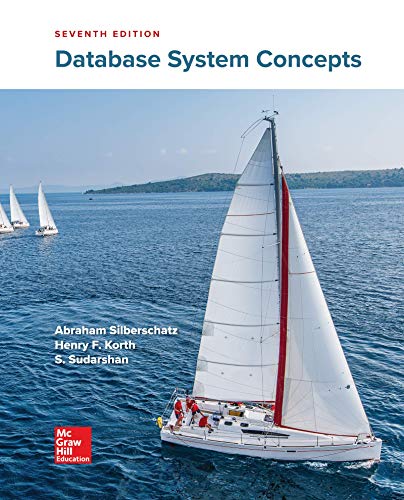
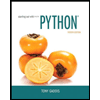
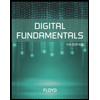
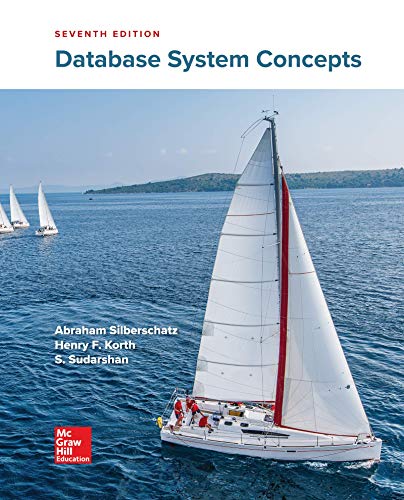
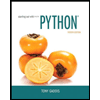
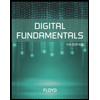
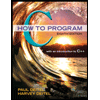
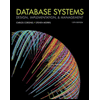
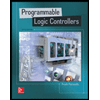