POINTERS-DYNAMIC ARRAY- EXCEPTION HANDLING POINTERS: GRADE ELIMINATION. A program that will input 10 score for quizzes (0-100) .Get the lowest quiz and eliminate the lowest quiz and compute and output the average of the 9 remaining quizzes. Finally, output only the SUM, LOWEST GRADE and the AVERAGE. DYNAMIC ARRAY & EXCEPTION HANDLING: COMPUTE FOR MILES PER GALLON Make a program that will calculate and compute for the quotient of miles and gallons (mpg: miles per gallons). Your program should must ask the user to specify the size of the array (using dynamic array) for the following variable: miles ,gallons and mpg. Prompt the user to Initialize the value of miles (value for miles should be 100-250) and gallons (values should be from 5-25). Use pointer galPtr for gallons, milPtr for miles and mpgPtr for mpg. Use function MilesPerrGallon (double,double) to compute for the values of mpg and use exception handling try-throw-catch to validate the values of miles and gallons. Finally set the width to 7 and the decimal places to 2. #include #include using namespace std; //global declaration int i; const int size=10; typedef double *pointers;//typedefinition used for miles per gallon pointer variables //prototype //for grade elimination void getInput(double *input);//prompt the user to input 10 grades double getLow(double *low);//compute and returns the value of the lowest grade double getSum(double *sum);//compute for the sum of 10 grades //choices and try again char getChoice(char *ch);//display the menu of choices and input the choice of the user //miles per gallon void MilesPerrGallon(double *ptr1,double *ptr2,int sz);//final output of the computer miles //and gallons void MperG();//prompts the user to specify the size of the array for miles and gallons //verify and validate the input for miles and gallons using exception handling int main() { //add only declaration here do{ chr=&choice; opt=getChoice(chr); switch(*chr) {
POINTERS-DYNAMIC ARRAY- EXCEPTION HANDLING
POINTERS:
GRADE ELIMINATION. A
DYNAMIC ARRAY & EXCEPTION HANDLING:
COMPUTE FOR MILES PER GALLON Make a program that will calculate and compute for the quotient of miles and gallons (mpg: miles per gallons). Your program should must ask the user to specify the size of the array (using dynamic array) for the following variable: miles ,gallons and mpg. Prompt the user to Initialize the value of miles (value for miles should be 100-250) and gallons (values should be from 5-25). Use pointer galPtr for gallons, milPtr for miles and mpgPtr for mpg. Use function MilesPerrGallon (double,double) to compute for the values of mpg and use exception handling try-throw-catch to validate the values of miles and gallons. Finally set the width to 7 and the decimal places to 2.
#include<iostream>
#include<iomanip>
using namespace std;
//global declaration
int i;
const int size=10;
typedef double *pointers;//typedefinition used for miles per gallon pointer variables
//prototype
//for grade elimination
void getInput(double *input);//prompt the user to input 10 grades
double getLow(double *low);//compute and returns the value of the lowest grade
double getSum(double *sum);//compute for the sum of 10 grades
//choices and try again
char getChoice(char *ch);//display the menu of choices and input the choice of the user
//miles per gallon
void MilesPerrGallon(double *ptr1,double *ptr2,int sz);//final output of the computer miles
//and gallons
void MperG();//prompts the user to specify the size of the array for miles and gallons
//verify and validate the input for miles and gallons using exception handling
int main()
{
//add only declaration here
do{
chr=&choice;
opt=getChoice(chr);
switch(*chr)
{
case '1':
{
double grade[size];
double *ptr;
double *plow, *psum, *pave;
double gsum,glow;
double ave;
system("cls");
//add declaration here
system("cls");
//add code here
//NOTE ALL CODES MUST USE pointer variables –input,process and //output
}
break;
case '2': MperG();
break;
case '3':
cout<<"goodbye for now....\n";
system("pause");
exit(1);
break;
}
//Add code here
cout<<endl;
system("pause");
return 0;
}
///////////////////////////
char getChoice(char *ch)
{
//add code here
}
//////////////////////////////
void getInput(double *input)
{
//add code here
}
/////////////////////////////
double getSum(double *sum)
{
double add=0;
//add code here
return add;
}
////////////////////////////////
double getLow(double *low)
{
double baba;
//add code here
return baba;
}
/////////////////////////
void MperG()
{
int ctr;
pointers milPtr,galPtr;
int msize;
system("cls");
//add code here
for(ctr=0;ctr<msize;ctr++)
{
//add code here
try{
//add code here
}//end try
catch(double e)
{
//add code here
}//end catch
//add code here
}//end while
}//loop for miles
/*********************************************************/
cout<<"GALLONS\n";
for(ctr=0;ctr<msize;ctr++)
{
bool ans=true;
while(ans){
//add code here
try{
//add code here
}//end try
catch(double e)
{
//add code here
}//end catch
//add code here
}//end while
}//loop for gallons
MilesPerrGallon(milPtr,galPtr,msize);
}
////////////////////////
void MilesPerrGallon(double *ptr1,double *ptr2,int sz)
{
double mpg[sz];
pointers mpgPtr;
int index;
//add code here
}
![SAMPLE OUTPUT
Choice[1]:Grade Elimination
enter 10 grades
grade[e]:45
grade[1]:89
grade[2]:33
grade[3]:78
grade[4]:90
grade[5]:89
grade[6]:78
grade[7]:77
grade[8]:76
grade[9]:65
<<<<<<<<<MENU>>
>>>
[1] score of 10 quizzes
[2] miles per gallon
[3] to quit
enter your choice:
<<<<<<<<<MENU>>>>>>>>>>
[1] score of 10 quizzes
[2] miles per gallon
[3] to quit
enter your choice: 6
«<<<<<<<<MENU>>>>>>>>>>
[1] score of 10 quizzes
[2] miles per gallon
[3] to quit
enter your choice:
you entered
grade[1]:45
grade[2]:89
grade[3]:33
grade[4]:78
grade[5]:90
grade[6]:89
grade[7]:78
grade[8]:77
grade[9]:76
grade[10]:65
sum = 720
lowest = 33
average =76.33
Do you want to try again?[y/n]:
Choice[1]:Grade Elimination
enter 10 grades
grade[0]:
%3D](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4aa948c0-25ab-4eb9-a89d-8ac61516c98d%2Fb4422203-edc9-44f8-a31c-9ca373f64eac%2Fm92e95_processed.jpeg&w=3840&q=75)
![you entered
grade[1]:45
grade[2]:89
grade[3]:33
grade[4]:78
grade[5]:90
grade[6]:89
grade[7]:78
grade[8]:77
grade[9]:76
grade[10]:65
sum = 720
lowest = 33
average =76.33
Do you want to try again?[y/n]:h
Do you want to try again?[y/n]:
COMPUTING FOR MPG : miles per gallon...
Specify the size of the array:
COMPUTING FOR MPG : miles per gallon...
Specify the size of the array:2
size 5-15 only
Specify the size of the array:
COMPUTING FOR MPG : miles per gallon...
Specify the size of the array:2
size 5-15 only
Specify the size of the array:6
MILES
miles[e]:
Do you want to try again?[y/n]:y
k<<<<<<<<<MENU>>>
[1] score of 10 quizzes
[2] miles per gallon
[3] to quit
enter your choice:
MILES
miles[0]:1
1.00 is invalid!.. 100-250 only
reenter a new value
miles[e]:
>>>>>
new value
gallons[2]:11
gallons[3]:11
gallons[4]:11
gallons[5]:11
reenter
miles[e]:1
miles[1]:11
miles[2]:111
miles[3]:111
miles[4]:111
miles[5]:111
GALLONS
gallons [e]:111
111.00 is invalid!.. 5-25 only
reenter a new value
gallons [@]:
MPG
10.09
niles
111.00 /
111.00 /
111.ee
111.00
111.00 /
111.00
Do you want to try again?[y/n]:
gallon
11.e0
11.00
10.09
11.e0
10.09
11.00
10.09
%3D
11.00
%3D
10.09
reenter a new value
gallons[e]:11
gallons[1]:11
gallons[2]:11
gallons[3]:11
gallons [4]:11
gallons [5]:11
11.00
10.09](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4aa948c0-25ab-4eb9-a89d-8ac61516c98d%2Fb4422203-edc9-44f8-a31c-9ca373f64eac%2Fzzywo6u_processed.jpeg&w=3840&q=75)

Step by step
Solved in 3 steps with 2 images

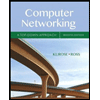
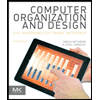
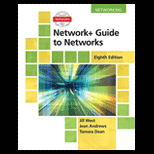
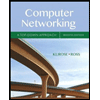
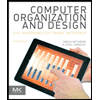
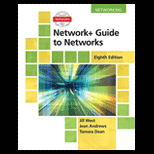
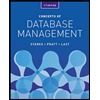
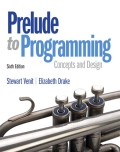
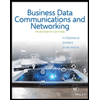