pub l class va lidParentheses{ //You will be given an array of characters which only contain '(' and ')' //You need to validate the parentheses: // If there is '(', there must be ')' but not neccessary right after // '(' and ')' need to be in correct order public static boolean validateParentheses(char[] parentheses){ //TODO: YOUR CODE HERE return false; //Return for compilation, this is wrong -> delete when done Run | Debug public static void main(String[] args){ char[] parentheses1 = {'(', ')'}; System.out.println("Test 1 passed: " + (validateParentheses(parentheses1) == true)); System.out.println(x: ."); char[] parentheses2 = {'(', '(', ')', ')'}; System.out.println("Test 2 passed: " + (validateParentheses (parentheses2) == true)); System.out.println(x: "- -"); char[] parentheses3 = {'(', '(', ')'}; // this is false because it needds an extra close parenthesis to match the first open parenthesis System.out.println("Test 3 passed: " + (validateParentheses(parentheses3) == false)); System.out.println(x: "- ");| char[] parentheses4 = {')', '(', ')', '('}; / this is false because of a close parenthesis appears before an open parenthesis (first one/index 0) System.out.println("Test 4 passed: " + (validateParentheses(parentheses4) == false)); System.out.println(x: "- "); char[] parentheses5 = {'(', ')', ')', ')', '(', '('}; // || this is false because of a close parenthesis appears before an open parenthesis (third one/index 2) System.out.println("Test 5 passed: " + (validateParentheses (parentheses5) == false)); System.out.println(x: "); char[] parentheses6 = {'(', ')', '(', ')'}; System.out.println("Test 6 passed: " + (validateParentheses (parentheses6) == true)); System.out.println(x: "- -"); char[] parentheses7 = {'(', '(', '(', ')', ')', '('}; // this is false because there is no close parenthesis to match openned ones (first one and sixth one) System.out.println("Test 7 passed: " + (validateParentheses(parentheses7) == true)); System.out.println(x: "- ");
pub l class va lidParentheses{ //You will be given an array of characters which only contain '(' and ')' //You need to validate the parentheses: // If there is '(', there must be ')' but not neccessary right after // '(' and ')' need to be in correct order public static boolean validateParentheses(char[] parentheses){ //TODO: YOUR CODE HERE return false; //Return for compilation, this is wrong -> delete when done Run | Debug public static void main(String[] args){ char[] parentheses1 = {'(', ')'}; System.out.println("Test 1 passed: " + (validateParentheses(parentheses1) == true)); System.out.println(x: ."); char[] parentheses2 = {'(', '(', ')', ')'}; System.out.println("Test 2 passed: " + (validateParentheses (parentheses2) == true)); System.out.println(x: "- -"); char[] parentheses3 = {'(', '(', ')'}; // this is false because it needds an extra close parenthesis to match the first open parenthesis System.out.println("Test 3 passed: " + (validateParentheses(parentheses3) == false)); System.out.println(x: "- ");| char[] parentheses4 = {')', '(', ')', '('}; / this is false because of a close parenthesis appears before an open parenthesis (first one/index 0) System.out.println("Test 4 passed: " + (validateParentheses(parentheses4) == false)); System.out.println(x: "- "); char[] parentheses5 = {'(', ')', ')', ')', '(', '('}; // || this is false because of a close parenthesis appears before an open parenthesis (third one/index 2) System.out.println("Test 5 passed: " + (validateParentheses (parentheses5) == false)); System.out.println(x: "); char[] parentheses6 = {'(', ')', '(', ')'}; System.out.println("Test 6 passed: " + (validateParentheses (parentheses6) == true)); System.out.println(x: "- -"); char[] parentheses7 = {'(', '(', '(', ')', ')', '('}; // this is false because there is no close parenthesis to match openned ones (first one and sixth one) System.out.println("Test 7 passed: " + (validateParentheses(parentheses7) == true)); System.out.println(x: "- ");
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![3
public class ValidParentheses{
//You will be given an array of characters which only contain '(' and ')'
//You need to validate the parentheses:
4
5
6
//
If there is '(', there must be ')' but not neccessary right after
7
'(' and ')' need to be in correct order
8
public static boolean validateParentheses (char[] parentheses){
9
//TODO: YOUR CODE HERE
10
11
return false; //Return for compilation, this is wrong -> delete when done
12
13
Run | Debug
public static void main(String[] args){
char[] parentheses1 = {'(', ')'};
System.out.println("Test 1 passed:
14
15
+ (validateParentheses (parentheses1) == true));
-");
16
17
System.out.println( x: ".
18
char[] parentheses2 = {'(', '(', ')', ')'};
System.out.println("Test 2 passed: " + (validateParentheses (parentheses2)
System.out.println(x: "
19
20
== true));
21
");
22
23
char[] parentheses3 = {'(', '(', ')'};
24
/ this is false because it needds an extra close parenthesis to match the first open parenthesis
System.out.println("Test 3 passed: "
System.out.println(x:
+ (validateParentheses (parentheses3)
");[
25
== false));
26
27
28
char[] parentheses4 = {')', '(', ')', '('};
/ this is false because of a close parenthesis appears before an open parenthesis (first one/index 0)
System.out.println("Test 4 passed: " + (validateParentheses (parentheses4)
System.out.println(x: "
29
30
== false));
31
");
32
33
char[] parentheses5 = {'(', ')', ')', ')', '(', '('};
// || this is false because of a close parenthesis appears before an open parenthesis (third one/index 2)
System.out.println("Test 5 passed: "
34
+ (validateParentheses (parentheses5) == false));
");
35
36
System.out.println(x: ".
37
char[] parentheses6 = {'(', ')', '(', ')'};
System.out.println("Test 6 passed: " + (validateParentheses (parentheses6)
System.out.println( x: ".
38
39
true));
==
40
");
41
42
char[] parentheses7 = {'(', '(', '(', ')', ')', '('};
// this is false because there is no close parenthesis to match openned ones (first one and sixth one)
System.out.println("Test 7 passed:
43
+ (validateParentheses (parentheses7) == true));
-");
44
45
System.out.println(x: ":
46
47
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5dfcde0a-cc8e-4f7e-b5e2-9ec6ad36a3c9%2F01aa82b2-8cc9-4cd1-8870-4fad8a25aad9%2F0z3g17_processed.png&w=3840&q=75)
Transcribed Image Text:3
public class ValidParentheses{
//You will be given an array of characters which only contain '(' and ')'
//You need to validate the parentheses:
4
5
6
//
If there is '(', there must be ')' but not neccessary right after
7
'(' and ')' need to be in correct order
8
public static boolean validateParentheses (char[] parentheses){
9
//TODO: YOUR CODE HERE
10
11
return false; //Return for compilation, this is wrong -> delete when done
12
13
Run | Debug
public static void main(String[] args){
char[] parentheses1 = {'(', ')'};
System.out.println("Test 1 passed:
14
15
+ (validateParentheses (parentheses1) == true));
-");
16
17
System.out.println( x: ".
18
char[] parentheses2 = {'(', '(', ')', ')'};
System.out.println("Test 2 passed: " + (validateParentheses (parentheses2)
System.out.println(x: "
19
20
== true));
21
");
22
23
char[] parentheses3 = {'(', '(', ')'};
24
/ this is false because it needds an extra close parenthesis to match the first open parenthesis
System.out.println("Test 3 passed: "
System.out.println(x:
+ (validateParentheses (parentheses3)
");[
25
== false));
26
27
28
char[] parentheses4 = {')', '(', ')', '('};
/ this is false because of a close parenthesis appears before an open parenthesis (first one/index 0)
System.out.println("Test 4 passed: " + (validateParentheses (parentheses4)
System.out.println(x: "
29
30
== false));
31
");
32
33
char[] parentheses5 = {'(', ')', ')', ')', '(', '('};
// || this is false because of a close parenthesis appears before an open parenthesis (third one/index 2)
System.out.println("Test 5 passed: "
34
+ (validateParentheses (parentheses5) == false));
");
35
36
System.out.println(x: ".
37
char[] parentheses6 = {'(', ')', '(', ')'};
System.out.println("Test 6 passed: " + (validateParentheses (parentheses6)
System.out.println( x: ".
38
39
true));
==
40
");
41
42
char[] parentheses7 = {'(', '(', '(', ')', ')', '('};
// this is false because there is no close parenthesis to match openned ones (first one and sixth one)
System.out.println("Test 7 passed:
43
+ (validateParentheses (parentheses7) == true));
-");
44
45
System.out.println(x: ":
46
47
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
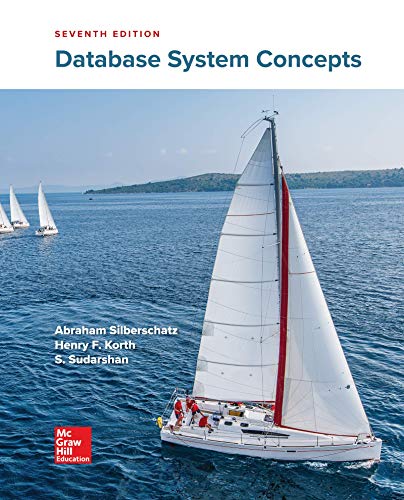
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
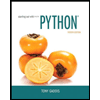
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
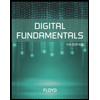
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
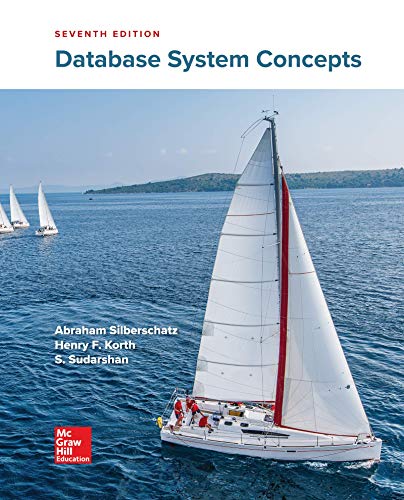
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
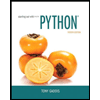
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
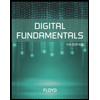
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
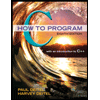
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
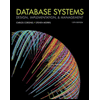
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
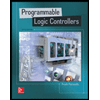
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education