PLEASE USE PYTHON PROGRAMMING Create a class called Numbers, which has a single class attribute called MULTIPLIER, and a constructor which takes the parameters x and y (these should all be numbers). Write a method called add which returns the sum of the attributes x and y. Write a class method called multiply, which takes a single number parameter aand returns the product of a and MULTIPLIER. Write a static method called subtract, which takes two number parameters, b and c, and returns b - c. Write a method called value which returns a tuple containing the values of x and y. Make this method into a property, and write a setter and a deleter for manipulating the values of x and y. class Numbers: # TODO: create a class attribute called MULTIPLIER def __init__(self, x, y): self.x = x self.y = y def add(self): # TODO: return x + y def multiply(self, a): # TODO: return the attribute MULTIPLIER * by a. @staticmethod def subtract(b, c): # TODO: # TODO: Make this a property def value(self): return self.x, self.y # TODO: Create a setter and a deleter for value. # test the class. num = Numbers(5,6) print(num.add()) print(num.multiply(2)) print(num.subtract(4, 4))
PLEASE USE PYTHON PROGRAMMING
Create a class called Numbers, which has a single class attribute called MULTIPLIER, and a constructor which takes the parameters x and y (these should all be numbers).
- Write a method called add which returns the sum of the attributes x and y.
- Write a class method called multiply, which takes a single number parameter aand returns the product of a and MULTIPLIER.
- Write a static method called subtract, which takes two number parameters, b and c, and returns b - c.
- Write a method called value which returns a tuple containing the values of x and y. Make this method into a property, and write a setter and a deleter for manipulating the values of x and y.
class Numbers:
# TODO: create a class attribute called MULTIPLIER
def __init__(self, x, y):
self.x = x
self.y = y
def add(self):
# TODO: return x + y
def multiply(self, a):
# TODO: return the attribute MULTIPLIER * by a.
@staticmethod
def subtract(b, c):
# TODO:
# TODO: Make this a property
def value(self):
return self.x, self.y
# TODO: Create a setter and a deleter for value.
# test the class.
num = Numbers(5,6)
print(num.add())
print(num.multiply(2))
print(num.subtract(4, 4))

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

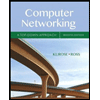
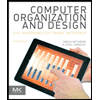
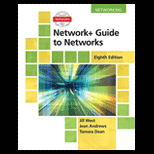
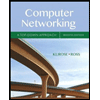
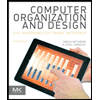
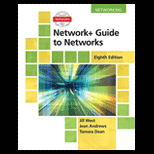
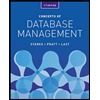
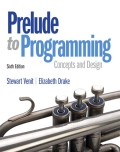
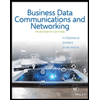