Please help me modify my code according to the error prompt #include void processData(const char* inputFilePath, const char* outputFilePath) { FILE* inputFile = fopen(inputFilePath, "r"); FILE* outputFile = fopen(outputFilePath, "w"); int n; fscanf(inputFile, "%d", &n); // Read the number of data points fprintf(outputFile, "%d\n", n); // Write the number of data points to output int value; for (int i = 0; i < n; i++) { fscanf(inputFile, "%d", &value); // Read the data point int squaredValue = value * value; // Compute the square of the data point fprintf(outputFile, "%d\n", squaredValue); // Write the squared value to output } fclose(inputFile); fclose(outputFile); } int main() { processData("input_file.dat", "output_file.dat"); return 0; }
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
Please help me modify my code according to the error prompt
#include <stdio.h>
void processData(const char* inputFilePath, const char* outputFilePath) {
FILE* inputFile = fopen(inputFilePath, "r");
FILE* outputFile = fopen(outputFilePath, "w");
int n;
fscanf(inputFile, "%d", &n); // Read the number of data points
fprintf(outputFile, "%d\n", n); // Write the number of data points to output
int value;
for (int i = 0; i < n; i++) {
fscanf(inputFile, "%d", &value); // Read the data point
int squaredValue = value * value; // Compute the square of the data point
fprintf(outputFile, "%d\n", squaredValue); // Write the squared value to output
}
fclose(inputFile);
fclose(outputFile);
}
int main() {
processData("input_file.dat", "output_file.dat");
return 0;
}
![Syntax Error(s)
_tester_.c: In function answer":
__tester_.c:58:5: error: 'main' is normally a non-static function [-Werror=main]
int main() {
ANNN
At top level:
_tester_.c:58:5: error: 'main' defined but not used [-Werror=unused-function]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5f0d30cb-f786-4882-9c09-523002c7167f%2Fa4e74ced-d096-446e-b78f-69787c8e414b%2Faw40qt8_processed.png&w=3840&q=75)

Step by step
Solved in 5 steps with 3 images

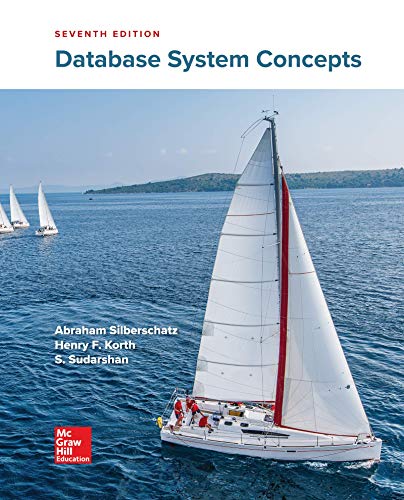
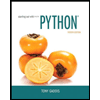
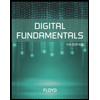
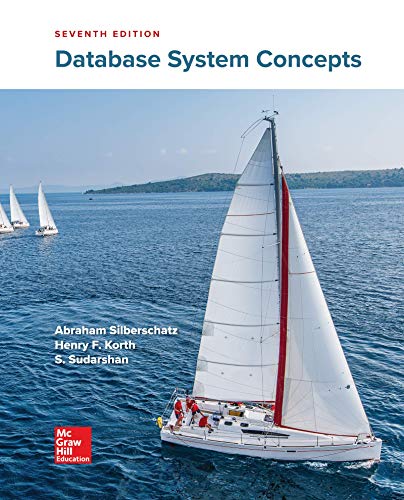
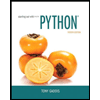
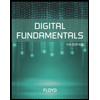
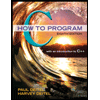
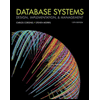
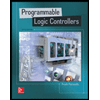