Convert Code Into Python and Eliminate Syntax errors: #include #include #include #include using namespace std; int main() { //declare input and output file sterams ifstream inFile; ofstream outFile; //declare required variables double roughness[50], min, max, sum=0, sqSum=0; double Ra, Rq, maxRoughness; int i = 0; //open input file inFile.open("Surface.txt"); //check input file exist or not if(!inFile) //display error message cout<<"Error in opening the file..."<>roughness[i]; //initialise min and max values min = max = roughness[i]; //initialise sum sum+=roughness[i]; //initialise sqSum sqSum+=roughness[i]*roughness[i]; //loop till end of file while(!inFile.eof()) { i++; //read value from file; one at a time inFile>>roughness[i]; //check value is less than min or not if(roughness[i]max) max=roughness[i]; //calculate cumulative sum sum+=roughness[i]; //calculate cumulative sum square if the value sqSum+=roughness[i]*roughness[i]; } //close infile inFile.close(); //increment i as it started from 0 i++; //calculate value of Ra Ra = abs(sum)/i; //calculate value of Rq Rq = sqrt(sqSum/i); //calculate value of Maximum roughness maxRoughness = max-min; //open out put file outFile.open("Indicators.txt"); //write message to output file outFile<<"Input data (measures of the roughness" <<" of the surface of an object):"<
Convert Code Into Python and Eliminate Syntax errors:
#include<iostream>
#include<fstream>
#include<math.h>
#include<iomanip>
using namespace std;
int main()
{
//declare input and output file sterams
ifstream inFile;
ofstream outFile;
//declare required variables
double roughness[50], min, max, sum=0, sqSum=0;
double Ra, Rq, maxRoughness;
int i = 0;
//open input file
inFile.open("Surface.txt");
//check input file exist or not
if(!inFile)
//display error message
cout<<"Error in opening the file..."<<endl;
else
{
//read the first value from file
inFile>>roughness[i];
//initialise min and max values
min = max = roughness[i];
//initialise sum
sum+=roughness[i];
//initialise sqSum
sqSum+=roughness[i]*roughness[i];
//loop till end of file
while(!inFile.eof())
{
i++;
//read value from file; one at a time
inFile>>roughness[i];
//check value is less than min or not
if(roughness[i]<min)
min=roughness[i];
//check value is greater than max or not
if(roughness[i]>max)
max=roughness[i];
//calculate cumulative sum
sum+=roughness[i];
//calculate cumulative sum square if the value
sqSum+=roughness[i]*roughness[i];
}
//close infile
inFile.close();
//increment i as it started from 0
i++;
//calculate value of Ra
Ra = abs(sum)/i;
//calculate value of Rq
Rq = sqrt(sqSum/i);
//calculate value of Maximum roughness
maxRoughness = max-min;
//open out put file
outFile.open("Indicators.txt");
//write message to output file
outFile<<"Input data (measures of the roughness"
<<" of the surface of an object):"<<endl<<endl;
//for formatting
outFile<<"\t";
//loop to write 6 values per line
for(int j=0; j<i; j++)
{
outFile<<roughness[j]<<" ";
//insert new line after 6 values
if((j+1)%6==0)
outFile<<endl<<"\t";
}
//for formatting
outFile<<endl<<endl<<endl;
//write the 3 surface roughness indicators to file
outFile<<"Arithmetic mean value (Ra) : "<<Ra<<endl;
outFile<<"Root-mean square average (Rq) : "<<Rq<<endl;
outFile<<"Maximum roughness height : "<<maxRoughness;
outFile.close();
}
//display message
cout<<"Calculations completed and the results are stored in Indicators.txt"
<<endl;
system("PAUSE");
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 10 images

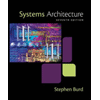
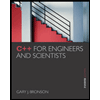
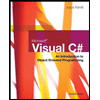
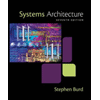
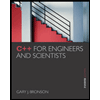
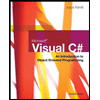
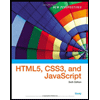
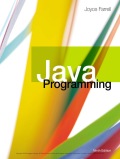