Part 1 code: #include <iostream> using namespace std; #include <cstdlib> int main() { srand(17); const int ARRAYSIZE = 20; // size for the array int RandArray[ARRAYSIZE]; // array declared int i; // to iterate the loop // this loop will store thei random number in the array for (i = 0; i < ARRAYSIZE; i++) RandArray[i] = rand() % 100; // this loop will print the array for (i = 0; i < ARRAYSIZE; i++) cout <<"randArray["<< i <<"]=" << RandArray[i] << endl; return 0; } At the bottom of the code from Part 1: Write code to find the largest value (and the index where it resides. Loop through all indices and update largestFoundSoFar (and the indexOfLargest) if the value in the array is even larger. At the end, print the largestFoundsoFar, and the index. largestFoundSoFar=95 at index 16 code format: #include <iostream>using namespace std; #include <cstdlib> // required for rand() int main(){// Put Part1 code here int largestFoundSoFar = -1; // anything is larger than this!int indexOfLargest = -1;// sign that it is not initialized// make a loop to check each element, // and if larger than largestFoundSoFar // update the largestFoundSoFar and indexOfLargest// print the largest and index. Example:// largestFoundSoFar=95 at index 16return 0;}
Part 1 code:
#include <iostream>
using namespace std;
#include <cstdlib>
int main()
{
srand(17);
const int ARRAYSIZE = 20; // size for the array
int RandArray[ARRAYSIZE]; // array declared
int i; // to iterate the loop
// this loop will store thei random number in the array
for (i = 0; i < ARRAYSIZE; i++)
RandArray[i] = rand() % 100;
// this loop will print the array
for (i = 0; i < ARRAYSIZE; i++)
cout <<"randArray["<< i <<"]=" << RandArray[i] << endl;
return 0;
}
At the bottom of the code from Part 1:
Write code to find the largest value (and the index where it resides.
- Loop through all indices and update largestFoundSoFar (and the indexOfLargest) if the value in the array is even larger.
- At the end, print the largestFoundsoFar, and the index.
largestFoundSoFar=95 at index 16
code format:
#include <iostream>
using namespace std;
#include <cstdlib> // required for rand()
int main()
{
// Put Part1 code here
int largestFoundSoFar = -1; // anything is larger than this!
int indexOfLargest = -1;// sign that it is not initialized
// make a loop to check each element,
// and if larger than largestFoundSoFar
// update the largestFoundSoFar and indexOfLargest
// print the largest and index. Example:
// largestFoundSoFar=95 at index 16
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

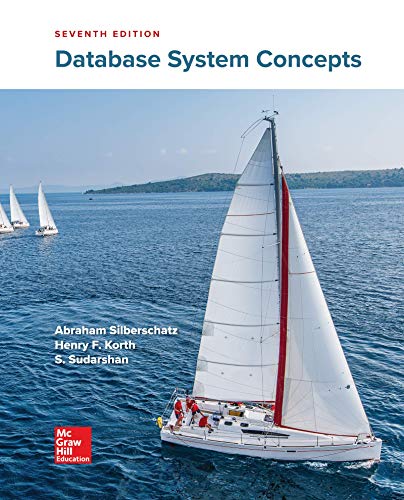
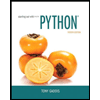
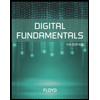
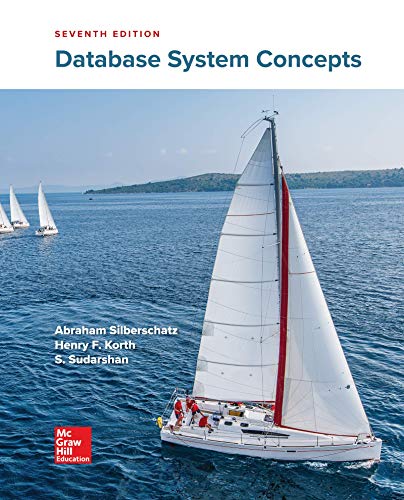
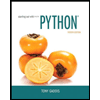
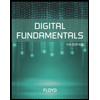
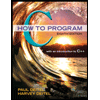
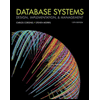
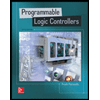