Write the C++ code of queue which is performed multiple task which are given below and performed all task by using Arrays. N.b array size=ten i)En-queue values Two,three,four,five,six,seven and eight ii)De-queue values Two and three iii)Please create is empty and queue function for telling them queue is empty or full.
Write the C++ code of queue which is performed multiple task which are given below and performed all task by using Arrays.
N.b array size=ten
i)En-queue values Two,three,four,five,six,seven and eight
ii)De-queue values Two and three
iii)Please create is empty and queue function for telling them queue is empty or full.
iv)Sort all values of queue and print it

#include <iostream>
using namespace std;
int queue[100], n = 10, front = - 1, rear = - 1;
bool isempty()
{
if(front == -1 && rear == -1)
return true;
else
return false;
}
bool isfull()
{
if (rear == n - 1)
return true;
else
return false;
}
void Enqueue(int value)
{
if (isfull())
cout<<"Queue is full"<<endl;
else
{
if (front == - 1)
front = 0;
rear++;
queue[rear] = value;
}
}
void Dequeue()
{
if( isempty() )
cout<<"Queue is empty\n";
else if( front == rear )
front = rear = -1;
else
{
cout<<"Element deleted : "<< queue[front] <<endl;
front++;
}
}
void print()
{
if (isempty())
cout<<"Queue is empty"<<endl;
else
{
cout<<"Queue : ";
for (int i = front; i <= rear; i++)
cout<<queue[i]<<" ";
cout<<endl;
}
}
void sortQueue()
{
//Sorting elements of queue using bubble sort
for (int j = 0; j < (n-1); ++j)
{
for (int i = 0; i < n - (j-1); ++i)
{
if (queue[i] > queue[i + 1])
{
int temp = queue[i];
queue[i] = queue[i + 1];
queue[i + 1] = temp;
}
}
}
}
int main()
{
//Enqueue operations
Enqueue(2);
Enqueue(3);
Enqueue(4);
Enqueue(5);
Enqueue(6);
Enqueue(7);
Enqueue(8);
print();
//Dequeue operations
Dequeue();
Dequeue();
print();
return 0;
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

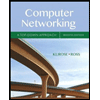
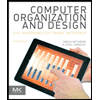
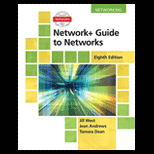
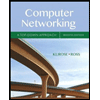
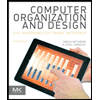
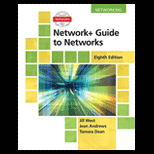
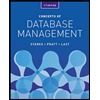
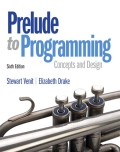
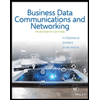