/** P3.2. Adding a method public void addInterest(double rate) to the BankAccount32 class which adds interest at the given rate. */ public class BankAccount32 { //instance variable private double balance ; /** Constructor: initializes balance to zero */ public BankAccount32() { balance = 0 ; } /** Constructor: initializes balance to given initialBalance paramter @param initialBalance */ public BankAccount32(double initialBalance) { balance = initialBalance ; } /** Adds interest to the BankAccount balance at the given rate @param rate the percent of interest to add HINT: don't forget to convert percent to fraction first! */ //-----------Start below here. To do: approximate lines of code = 2 // Write the addInterest method //-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions. /** Returns the balance @return the balance */ public double getBalance() { return balance ; } /** Deposits amount of money to the account @param amount the amount of money to put in */ public void deposit(double amount) { balance = balance + amount ; } /** Withdraws amount of money to the account @param amount the amount of money to take out */ public void withdraw(double amount) { double newBalance = balance - amount ; balance = newBalance ; } /** Returns a string representation of the account @return the string representation */ public String toString() { return "BankAccount[balance = " + balance + "]" ; } }
/** P3.2. Adding a method public void addInterest(double rate) to the BankAccount32 class which adds interest at the given rate. */ public class BankAccount32 { //instance variable private double balance ; /** Constructor: initializes balance to zero */ public BankAccount32() { balance = 0 ; } /** Constructor: initializes balance to given initialBalance paramter @param initialBalance */ public BankAccount32(double initialBalance) { balance = initialBalance ; } /** Adds interest to the BankAccount balance at the given rate @param rate the percent of interest to add HINT: don't forget to convert percent to fraction first! */ //-----------Start below here. To do: approximate lines of code = 2 // Write the addInterest method //-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions. /** Returns the balance @return the balance */ public double getBalance() { return balance ; } /** Deposits amount of money to the account @param amount the amount of money to put in */ public void deposit(double amount) { balance = balance + amount ; } /** Withdraws amount of money to the account @param amount the amount of money to take out */ public void withdraw(double amount) { double newBalance = balance - amount ; balance = newBalance ; } /** Returns a string representation of the account @return the string representation */ public String toString() { return "BankAccount[balance = " + balance + "]" ; } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
/**
P3.2.
Adding a method
public void addInterest(double rate)
to the BankAccount32 class which adds interest at the given rate.
*/
public class BankAccount32
{
//instance variable
private double balance ;
/**
Constructor: initializes balance to zero
*/
public BankAccount32()
{
balance = 0 ;
}
/**
Constructor: initializes balance to given initialBalance paramter
@param initialBalance
*/
public BankAccount32(double initialBalance)
{
balance = initialBalance ;
}
/**
Adds interest to the BankAccount balance at the given rate
@param rate the percent of interest to add
HINT: don't forget to convert percent to fraction first!
*/
//-----------Start below here. To do: approximate lines of code = 2
// Write the addInterest method
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
/**
Returns the balance
@return the balance
*/
public double getBalance()
{
return balance ;
}
/**
Deposits amount of money to the account
@param amount the amount of money to put in
*/
public void deposit(double amount)
{
balance = balance + amount ;
}
/**
Withdraws amount of money to the account
@param amount the amount of money to take out
*/
public void withdraw(double amount)
{
double newBalance = balance - amount ;
balance = newBalance ;
}
/**
Returns a string representation of the account
@return the string representation
*/
public String toString()
{
return "BankAccount[balance = " + balance + "]" ;
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
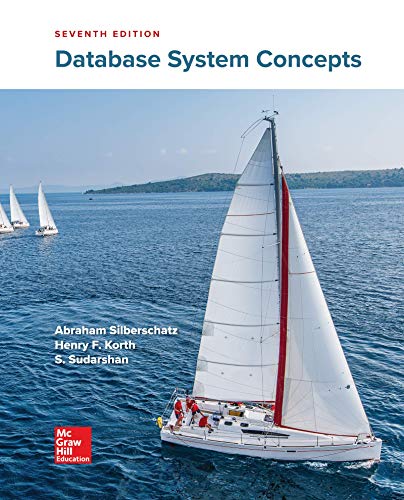
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
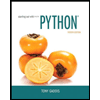
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
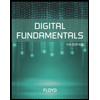
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
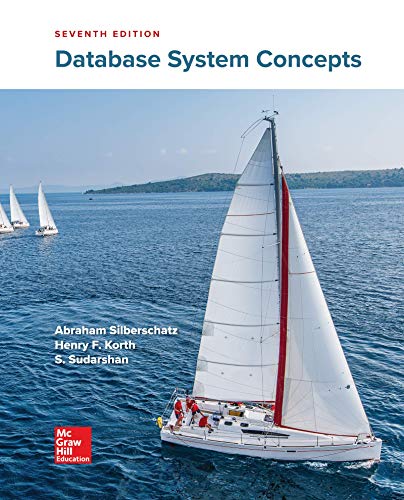
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
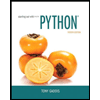
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
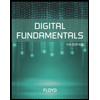
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
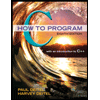
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
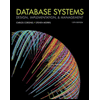
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
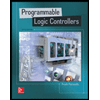
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education