our main task is to write a recursive function sierpinski() that plots a Sierpinski triangle of order n to standard drawing. Think recursively: sierpinski() should draw one filled equilateral triangle (pointed downwards) and then call itself recursively three times (with an appropriate stopping condition). It should draw 1 filled triangle for n = 1; 4 filled triangles for n = 2; and 13 filled triangles for n = 3; and so forth. Sierpinski.java When writing your program, exercise modular design by organizing it into four functions, as specified in the following API: public class Sierpinski { // Height of an equilateral triangle with the specified side length. public static double height(double length) // Draws a filled equilateral triangle with the specified side length // whose bottom vertex is (x, y). public static void filledTriangle(double x, double y, double length) // Draws a Sierpinski triangle of order n, such that the largest filled // triangle has the specified side length and bottom vertex (x, y). public static void sierpinski(int n, double x, double y, double length) // Takes an integer command-line argument n; // draws the outline of an upwards equilateral triangle of length 1 // whose bottom-left vertex is (0, 0) and bottom-right vertex is (1, 0); // and draws a Sierpinski triangle of order n that fits inside the outline. public static void main(String[] args) }
Your main task is to write a recursive function sierpinski() that plots a Sierpinski triangle of order n to standard drawing. Think recursively: sierpinski() should draw one filled equilateral triangle (pointed downwards) and then call itself recursively three times (with an appropriate stopping condition). It should draw 1 filled triangle for n = 1; 4 filled triangles for n = 2; and 13 filled triangles for n = 3; and so forth.
Sierpinski.java
When writing your program, exercise modular design by organizing it into four functions, as specified in the following API:
public class Sierpinski { // Height of an equilateral triangle with the specified side length. public static double height(double length) // Draws a filled equilateral triangle with the specified side length // whose bottom vertex is (x, y). public static void filledTriangle(double x, double y, double length) // Draws a Sierpinski triangle of order n, such that the largest filled // triangle has the specified side length and bottom vertex (x, y). public static void sierpinski(int n, double x, double y, double length) // Takes an integer command-line argument n; // draws the outline of an upwards equilateral triangle of length 1 // whose bottom-left vertex is (0, 0) and bottom-right vertex is (1, 0); // and draws a Sierpinski triangle of order n that fits inside the outline. public static void main(String[] args) } |

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

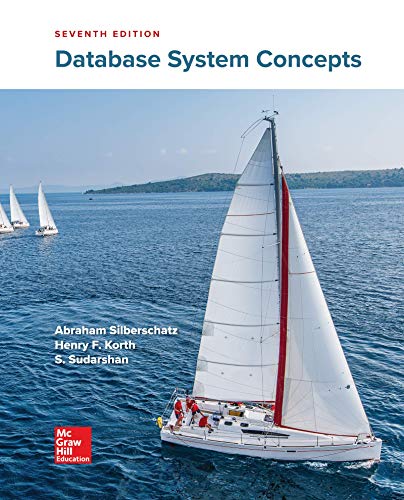
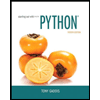
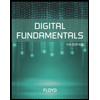
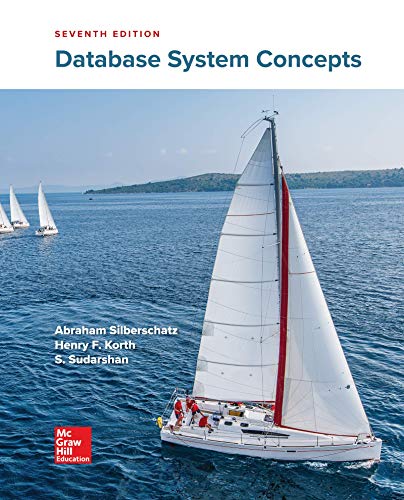
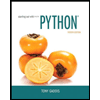
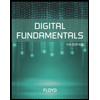
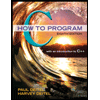
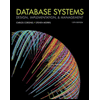
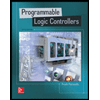