ONLY LAST TWO PARTS WHERE IT SAY TODO//////////////////////////// package com.mac286.ourvector; /* * Design a class ourVector that mimics the javaVector. The class has the following * properties: - A private variable size that keeps track of how many elements in the array - A reference to an array of integers - An increment by how much to increase wjen it's full - A default constructor that creates an array of 5 with increment 10; - A constructor that accepts the initial capacity and creates an array of that capacity and an increment of twice the capacity - A constructor that accepts two integers, the first for the capacity and the second for increment. - Capacity, the length of the array - int size(); A method that returns the size of the array - boolean isEmpty(); returns true if it's empty and false if not. - void addBack(int e); adds e to the back of the array - void addFront(int e); adds e to the front of the array. - Override String toString(); to return the content of the array in the form [-1, -4, -6] - int elementAt(int ind); returns the elements at index ind, if valid. - int removeBack(); removes the element in the back of the array and returns it (update size) - int removeFront(); removes the element in the front of the array and returns it (update size) - void add(int ind, int e); adds e to the index ind. - int remove(int ind); removes element at index ind and returns it. */ /* So far our vector works only with integer. In order to create a vector that works with any type we use generic type. class className { } * */ public class ourVector { //private variables privateintsize; //Let's use .length fro capacity no need for another variable privateintincrement; privateT[]V;//V is a reference //constructors publicourVector(){ V=(T[])newObject[5]; size=0; increment=10; } publicourVector(intcapacity){ V=(T[])newObject[capacity];; size=0; increment=2*capacity; } publicourVector(intcapacity,intincr){ V=(T[])newObject[capacity]; size=0; increment=incr; } //methods publicintsize(){ returnsize; } publicbooleanisEmpty(){ return(size==0);//if size is 0 return true else return false } //WH TODO: Finish the resize method and test it privatevoidresize(){ //create a temporary array temp with capacity V.length+increment T[]temp=(T[])newObject[V.length+increment]; //copy all elements from V to temp for(inti=0;i=0&&ind0;i--){ V[i]=V[i-1]; } //insert e at index 0 V[0]=e; //increase size. size++; } //removeFront removes the front and returns publicTremoveFront(){ if(this.isEmpty()){ System.out.println("The vector is empty"); returnnull; } //save the first Tsave=V[0]; //bring all elements down by one for(inti=1;i
ONLY LAST TWO PARTS WHERE IT SAY TODO////////////////////////////
package com.mac286.ourvector;
/*
* Design a class ourVector that mimics the javaVector. The class has the following
* properties:
- A private variable size that keeps track of how many elements in the array
- A reference to an array of integers
- An increment by how much to increase wjen it's full
- A default constructor that creates an array of 5 with increment 10;
- A constructor that accepts the initial capacity and creates an array of that capacity
and an increment of twice the capacity
- A constructor that accepts two integers, the first for the capacity and the second for
increment.
- Capacity, the length of the array
- int size(); A method that returns the size of the array
- boolean isEmpty(); returns true if it's empty and false if not.
- void addBack(int e); adds e to the back of the array
- void addFront(int e); adds e to the front of the array.
- Override String toString(); to return the content of the array in the form
[-1, -4, -6]
- int elementAt(int ind); returns the elements at index ind, if valid.
- int removeBack(); removes the element in the back of the array and returns it (update size)
- int removeFront(); removes the element in the front of the array and returns it (update size)
- void add(int ind, int e); adds e to the index ind.
- int remove(int ind); removes element at index ind and returns it.
*/
/*
So far our
type we use generic type. class className <T> { }
*
*/
public class ourVector <T> {
//private variables
privateintsize;
//Let's use .length fro capacity no need for another variable
privateintincrement;
privateT[]V;//V is a reference
//constructors
publicourVector(){
V=(T[])newObject[5];
size=0;
increment=10;
}
publicourVector(intcapacity){
V=(T[])newObject[capacity];;
size=0;
increment=2*capacity;
}
publicourVector(intcapacity,intincr){
V=(T[])newObject[capacity];
size=0;
increment=incr;
}
//methods
publicintsize(){
returnsize;
}
publicbooleanisEmpty(){
return(size==0);//if size is 0 return true else return false
}
//WH TODO: Finish the resize method and test it
privatevoidresize(){
//create a temporary array temp with capacity V.length+increment
T[]temp=(T[])newObject[V.length+increment];
//copy all elements from V to temp
for(inti=0;i<size;i++){
temp[i]=V[i];
}
//assign temp to V.
V=temp;
return;
}
publicintcapacity(){
returnV.length;
}
publicvoidaddBack(Te){
//test first if it's not full
if(size==V.length){
System.out.println("The vector is full resizing");
this.resize();
}
//insert e at index size
V[size]=e;
size++;
}
publicvoidadd(Te){
this.addBack(e);
}
//remove removes the back and returns it
Tremove(){
returnremoveBack();
}
//removes the back and returns it.
publicTremoveBack(){
//if it is empty then display a message and return 0.
if(this.isEmpty()){
System.out.println("Vector empty");
returnnull;
}
//save the last element
Tsave=V[size-1];
//decrease the size by one
size--;
//return the saved element
returnsave;
}
//T get(intind) returns the element at index ind. If the index is invalid
//display a message.
publicTget(intind)throwsIndexOutOfBoundsException{
if(ind>=0&&ind<size){
returnV[ind];
}
System.out.println("Invlid index");
thrownewIndexOutOfBoundsException();
}
publicTelementAt(intind){
returnthis.get(ind);
}
//void addFront(int e) adds e to the front of the vector
publicvoidaddFront(Te){
//if full resize
if(size==V.length){
this.resize();
}
//push all elements up by one starting from the last one
for(inti=size;i>0;i--){
V[i]=V[i-1];
}
//insert e at index 0
V[0]=e;
//increase size.
size++;
}
//removeFront removes the front and returns
publicTremoveFront(){
if(this.isEmpty()){
System.out.println("The vector is empty");
returnnull;
}
//save the first
Tsave=V[0];
//bring all elements down by one
for(inti=1;i<size;i++){
V[i-1]=V[i];
}
size--;
returnsave;
}
//toString to return the array as a string in the form [-1, -5, -7]
publicStringtoString(){
if(this.isEmpty()){
return"[]";
}
Stringst="[";
for(inti=0;i<size-1;i++){
st+=V[i].toString()+", ";
}
//deal with the last one separately
st+=V[size-1]+"]";
returnst;
}
//OR THIS WAY
/*
public String toString() {
if(this.isEmpty()) {
return "[]";
}
String st = "[" + V[0];
for(int i =1; i<size; i++ ) {
st += ", " + V[i];
}
//deal with the last one separately
st += "]";
return st;
}
*/
//TODO//////////////////////////////////////
//inserts e at index ind
publicvoidadd(intind,Te){
//if index is invalid display a message and return
//valid index are 0 to size
//push all elements up by one all the down to index ind (stop at index ind)
//insert e at index ind
//increase the size
}
//TODO ///////////////////////////////////////////////
publicTremove(intind){
//if index is invalid display a message and return null
//save element at ind
//bring down all elements by one starting at index ind
//decrease size
//return the saved element.
}
}

Below is the complete solution with explanation in detail for the given question about TODOs in the given code in Java Programming Language.
Step by step
Solved in 4 steps

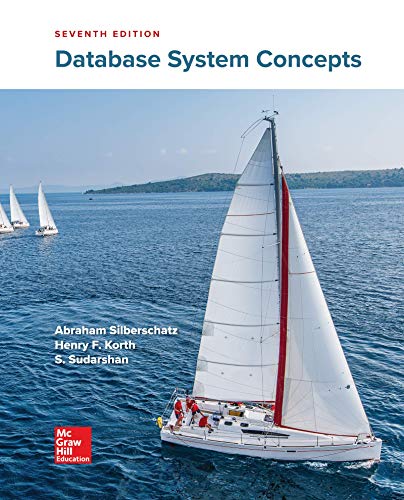
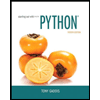
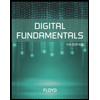
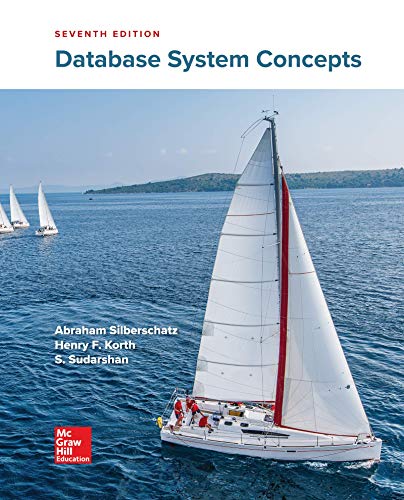
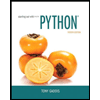
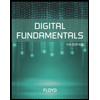
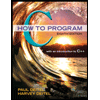
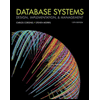
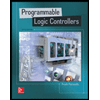