Note: Help me debug and complete code The program should do the following: Show the customer the different products sold by the candy machine Let the customer make the selection Show the customer the cost of the item selected Accept money from the customer Return change Release the item, that is, make the sale import java.util.InputMismatchException; import java.util.*; public class CandyMachine { CashRegistercashRegister; Dispensercandy; Dispenserchips; Dispensergum; Dispensercookies; publicCandyMachine(){ CashRegistercashRegister = newCashRegister(); Dispensercandy = newDispenser(); // -- Your Code for the rest of the components Dispenserchips = newDispenser(); Dispensergum = newDispenser(); Dispensercookies = newDispenser(); } publicclassCandyMachine1 { publicstaticvoidmain(String[] args) { System.out.println("*** Welcome to Anjit's Candy Shop ***"); ProductDispenserCandy = newProductDispenser(0.58, 12); ProductDispenserChips = newProductDispenser(0.69, 12); ProductDispenserGum = newProductDispenser(0.35, 12); ProductDispenserCookies = newProductDispenser(0.85, 12); CashRegistercashRegister = newCashRegister(); } publicCandyMachine() { Scannerconsole = newScanner(System.in); cashRegister = new CashRegister(console.nextInt()); candy = new candy (console.nextInt()); } publicvoidOn(){ Scannerinput = newScanner(System.in); intchoice; showSelection(); choice = input.nextInt(); } privatevoidshowSelection(){ System.out.println("*** Welcome ***"); System.out.println("To select an item, enter "); System.out.println("0 for Adding more cash"); System.out.println("1 for Candy"); System.out.println("2 for Chips"); System.out.println("3 for Gum"); System.out.println("4 for Cookies"); System.out.println("9 to exit"); intselect = 0; do { select = getIntInput("\n To select an item, enter \n" + "1 for Candy\n" + "2 for Chips\n" + "3 for Gum\n" + "4 for Cookies\n" + "9 to exit \n"); switch (select) { case1: purchase(Candy, cashRegister); break; case2: purchase(Chips, cashRegister); break; case3: purchase(Gum, cashRegister); break; case4: purchase(Cookies, cashRegister); break; case9: break; default: System.out.println("Invalid Selection"); } } while (select != 9); } } privatevoidsellProduct(Dispenserproduct, CashRegistercRegister) { Scannerinput = newScanner(System.in); if (product.getProdQty() > 0) { doublepmt = 0; do { System.out.print("Please deposit " + (product.getProdCost() * 100 - pmt) + " cents."); pmt += input.nextDouble(); } while (pmt < product.getProdCost() * 100); product.setProdQty(1); cRegister.setCashOnHand(product.getProdCost()); System.out.println("Collect your item at the bottom and enjoy."); } } publicstaticintgetIntInput(Stringprompt) { Scannerinput = newScanner(System.in); System.out.print(prompt); intintValue = 10; try { intValue = input.nextInt(); } catch (InputMismatchException e) { System.out.println("*** That is not a number. ***"); } return intValue; } } //cashRegister public class CashRegister { privateintcashOnHand; //Default constructor to set the cash in the register to 0 //Postcondition: cashOnHand = 0 publicCashRegister() { // -- Your Code Here privatedoublecashOnHand; CashRegister() { cashOnHand = 0; } //Constructor with parameters to set the cash in the register to a specific amount //Postcondition: cashOnHand = cashIn publicCashRegister(int cashIn) { // -- Your Code Here doublegetCashOnHand() { return cashOnHand; } //Method to show the current amount in the cash register //Postcondition: The value of the instance variable cashOnHand is returned. publicintcurrentBalance() { // -- Your Code Here } //Method to receives the amount deposited by the customer and updates the amount in the register //Postcondition: cashOnHand = cashOnHand + amountIn publicvoidacceptAmount(int amountIn) { // -- Your Code Here voidsetCashOnHand(double purchase) { cashOnHand += purchase; } //Method to deduct the amount deposited by the customer and updates the amount in the register //Postcondition: cashOnHand = cashOnHand - amountCost publicvoiddeductAmount(int amountCost) { // -- Your Code Here } } //Dispenser public class Dispenser { privateintnumberOfItems; privateintcost; publicDispenser() { privatedoubleprodCost; privateintprodQty; } publicDispenser(intsetNoOfItems, intsetCost) { prodQTy= setNoOfItems; prodCost= setCost; } //Method to show the number of items in the dispenser //Postcondition: The value of the instance variable numberOfItems is returned. publicintgetCount() { // -- Your Code Here } //Method to show the cost of the item //Postcondition: The value of the instance variable cost is returned. publicintgetProductCost() { returnprodCost(); } //Method to reduce the number of items by 1 //Postcondition: numberOfItems = numberOfItems - 1 publicvoidmakeSale() { numberOfItems--; } }
Note: Help me debug and complete code The program should do the following: Show the customer the different products sold by the candy machine Let the customer make the selection Show the customer the cost of the item selected Accept money from the customer Return change Release the item, that is, make the sale import java.util.InputMismatchException; import java.util.*; public class CandyMachine { CashRegistercashRegister; Dispensercandy; Dispenserchips; Dispensergum; Dispensercookies; publicCandyMachine(){ CashRegistercashRegister = newCashRegister(); Dispensercandy = newDispenser(); // -- Your Code for the rest of the components Dispenserchips = newDispenser(); Dispensergum = newDispenser(); Dispensercookies = newDispenser(); } publicclassCandyMachine1 { publicstaticvoidmain(String[] args) { System.out.println("*** Welcome to Anjit's Candy Shop ***"); ProductDispenserCandy = newProductDispenser(0.58, 12); ProductDispenserChips = newProductDispenser(0.69, 12); ProductDispenserGum = newProductDispenser(0.35, 12); ProductDispenserCookies = newProductDispenser(0.85, 12); CashRegistercashRegister = newCashRegister(); } publicCandyMachine() { Scannerconsole = newScanner(System.in); cashRegister = new CashRegister(console.nextInt()); candy = new candy (console.nextInt()); } publicvoidOn(){ Scannerinput = newScanner(System.in); intchoice; showSelection(); choice = input.nextInt(); } privatevoidshowSelection(){ System.out.println("*** Welcome ***"); System.out.println("To select an item, enter "); System.out.println("0 for Adding more cash"); System.out.println("1 for Candy"); System.out.println("2 for Chips"); System.out.println("3 for Gum"); System.out.println("4 for Cookies"); System.out.println("9 to exit"); intselect = 0; do { select = getIntInput("\n To select an item, enter \n" + "1 for Candy\n" + "2 for Chips\n" + "3 for Gum\n" + "4 for Cookies\n" + "9 to exit \n"); switch (select) { case1: purchase(Candy, cashRegister); break; case2: purchase(Chips, cashRegister); break; case3: purchase(Gum, cashRegister); break; case4: purchase(Cookies, cashRegister); break; case9: break; default: System.out.println("Invalid Selection"); } } while (select != 9); } } privatevoidsellProduct(Dispenserproduct, CashRegistercRegister) { Scannerinput = newScanner(System.in); if (product.getProdQty() > 0) { doublepmt = 0; do { System.out.print("Please deposit " + (product.getProdCost() * 100 - pmt) + " cents."); pmt += input.nextDouble(); } while (pmt < product.getProdCost() * 100); product.setProdQty(1); cRegister.setCashOnHand(product.getProdCost()); System.out.println("Collect your item at the bottom and enjoy."); } } publicstaticintgetIntInput(Stringprompt) { Scannerinput = newScanner(System.in); System.out.print(prompt); intintValue = 10; try { intValue = input.nextInt(); } catch (InputMismatchException e) { System.out.println("*** That is not a number. ***"); } return intValue; } } //cashRegister public class CashRegister { privateintcashOnHand; //Default constructor to set the cash in the register to 0 //Postcondition: cashOnHand = 0 publicCashRegister() { // -- Your Code Here privatedoublecashOnHand; CashRegister() { cashOnHand = 0; } //Constructor with parameters to set the cash in the register to a specific amount //Postcondition: cashOnHand = cashIn publicCashRegister(int cashIn) { // -- Your Code Here doublegetCashOnHand() { return cashOnHand; } //Method to show the current amount in the cash register //Postcondition: The value of the instance variable cashOnHand is returned. publicintcurrentBalance() { // -- Your Code Here } //Method to receives the amount deposited by the customer and updates the amount in the register //Postcondition: cashOnHand = cashOnHand + amountIn publicvoidacceptAmount(int amountIn) { // -- Your Code Here voidsetCashOnHand(double purchase) { cashOnHand += purchase; } //Method to deduct the amount deposited by the customer and updates the amount in the register //Postcondition: cashOnHand = cashOnHand - amountCost publicvoiddeductAmount(int amountCost) { // -- Your Code Here } } //Dispenser public class Dispenser { privateintnumberOfItems; privateintcost; publicDispenser() { privatedoubleprodCost; privateintprodQty; } publicDispenser(intsetNoOfItems, intsetCost) { prodQTy= setNoOfItems; prodCost= setCost; } //Method to show the number of items in the dispenser //Postcondition: The value of the instance variable numberOfItems is returned. publicintgetCount() { // -- Your Code Here } //Method to show the cost of the item //Postcondition: The value of the instance variable cost is returned. publicintgetProductCost() { returnprodCost(); } //Method to reduce the number of items by 1 //Postcondition: numberOfItems = numberOfItems - 1 publicvoidmakeSale() { numberOfItems--; } }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Note: Help me debug and complete code
The
- Show the customer the different products sold by the candy machine
- Let the customer make the selection
- Show the customer the cost of the item selected
- Accept money from the customer
- Return change
- Release the item, that is, make the sale
import java.util.InputMismatchException;
import java.util.*;
public class CandyMachine {
CashRegistercashRegister;
Dispensercandy;
Dispenserchips;
Dispensergum;
Dispensercookies;
publicCandyMachine(){
CashRegistercashRegister = newCashRegister();
Dispensercandy = newDispenser();
// -- Your Code for the rest of the components
Dispenserchips = newDispenser();
Dispensergum = newDispenser();
Dispensercookies = newDispenser();
}
publicclassCandyMachine1 {
publicstaticvoidmain(String[] args) {
System.out.println("*** Welcome to Anjit's Candy Shop ***");
ProductDispenserCandy = newProductDispenser(0.58, 12);
ProductDispenserChips = newProductDispenser(0.69, 12);
ProductDispenserGum = newProductDispenser(0.35, 12);
ProductDispenserCookies = newProductDispenser(0.85, 12);
CashRegistercashRegister = newCashRegister();
}
publicCandyMachine() {
Scannerconsole = newScanner(System.in);
cashRegister = new CashRegister(console.nextInt());
candy = new candy (console.nextInt());
}
publicvoidOn(){
Scannerinput = newScanner(System.in);
intchoice;
showSelection();
choice = input.nextInt();
}
privatevoidshowSelection(){
System.out.println("*** Welcome ***");
System.out.println("To select an item, enter ");
System.out.println("0 for Adding more cash");
System.out.println("1 for Candy");
System.out.println("2 for Chips");
System.out.println("3 for Gum");
System.out.println("4 for Cookies");
System.out.println("9 to exit");
intselect = 0;
do {
select = getIntInput("\n To select an item, enter \n" + "1 for Candy\n"
+ "2 for Chips\n" + "3 for Gum\n" + "4 for Cookies\n"
+ "9 to exit \n");
switch (select) {
case1:
purchase(Candy, cashRegister);
break;
case2:
purchase(Chips, cashRegister);
break;
case3:
purchase(Gum, cashRegister);
break;
case4:
purchase(Cookies, cashRegister);
break;
case9:
break;
default:
System.out.println("Invalid Selection");
}
} while (select != 9);
}
}
privatevoidsellProduct(Dispenserproduct, CashRegistercRegister)
{
Scannerinput = newScanner(System.in);
if (product.getProdQty() > 0) {
doublepmt = 0;
do {
System.out.print("Please deposit " + (product.getProdCost() * 100 - pmt) + " cents.");
pmt += input.nextDouble();
} while (pmt < product.getProdCost() * 100);
product.setProdQty(1);
cRegister.setCashOnHand(product.getProdCost());
System.out.println("Collect your item at the bottom and enjoy.");
}
}
publicstaticintgetIntInput(Stringprompt) {
Scannerinput = newScanner(System.in);
System.out.print(prompt);
intintValue = 10;
try {
intValue = input.nextInt();
} catch (InputMismatchException e) {
System.out.println("*** That is not a number. ***");
}
return intValue;
}
}
//cashRegister
public class CashRegister
{
privateintcashOnHand;
//Default constructor to set the cash in the register to 0
//Postcondition: cashOnHand = 0
publicCashRegister()
{
// -- Your Code Here
privatedoublecashOnHand;
CashRegister() {
cashOnHand = 0;
}
//Constructor with parameters to set the cash in the register to a specific amount
//Postcondition: cashOnHand = cashIn
publicCashRegister(int cashIn)
{
// -- Your Code Here
doublegetCashOnHand() {
return cashOnHand;
}
//Method to show the current amount in the cash register
//Postcondition: The value of the instance variable cashOnHand is returned.
publicintcurrentBalance()
{
// -- Your Code Here
}
//Method to receives the amount deposited by the customer and updates the amount in the register
//Postcondition: cashOnHand = cashOnHand + amountIn
publicvoidacceptAmount(int amountIn)
{
// -- Your Code Here
voidsetCashOnHand(double purchase) {
cashOnHand += purchase;
}
//Method to deduct the amount deposited by the customer and updates the amount in the register
//Postcondition: cashOnHand = cashOnHand - amountCost
publicvoiddeductAmount(int amountCost)
{
// -- Your Code Here
}
}
//Dispenser
public class Dispenser
{
privateintnumberOfItems;
privateintcost;
publicDispenser()
{
privatedoubleprodCost;
privateintprodQty;
}
publicDispenser(intsetNoOfItems, intsetCost)
{
prodQTy= setNoOfItems;
prodCost= setCost;
}
//Method to show the number of items in the dispenser
//Postcondition: The value of the instance variable numberOfItems is returned.
publicintgetCount()
{
// -- Your Code Here
}
//Method to show the cost of the item
//Postcondition: The value of the instance variable cost is returned.
publicintgetProductCost()
{
returnprodCost();
}
//Method to reduce the number of items by 1
//Postcondition: numberOfItems = numberOfItems - 1
publicvoidmakeSale()
{
numberOfItems--;
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
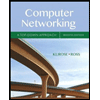
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
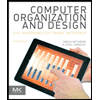
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
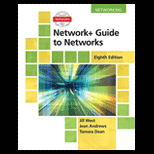
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
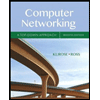
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
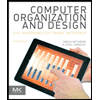
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
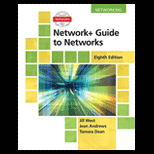
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
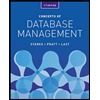
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
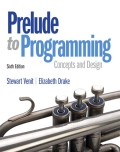
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
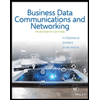
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY