Note - Give a step-by-step and brief explanation for your solution. To develop a user interface for our already-existing parking management system (class diagram included below – image 1), while ensuring that our code is loosely coupled, we need to provide a layer on top of the ParkingOffice class that will accept commands and send them on. This layer will be the ParkingService (class diagram included below- image 2) For simplicity, we’ll represent the commands and their arguments as strings. We must implement the following commands: CUSTOMER - register a customer, along with the appropriate information, with the parking office. The sequence diagram for this command is shown below (Image 2). CAR - register a car with the parking office and provide a permit for the car. The sequence diagram is very similar to that of the CUSTOMER registration. Based on the diagram below use java code to create the following classes and interfaces and implement the behavior described in the sequence diagram for both car and customer commands: ParkingService Command RegisterCarCommand RegisterCustomerCommand We have already implemented the classes in Image 1 (car, customer, parking office, parking charge, parking lot, and money). We just need the code for the above four. Use the UML diagram and generate your code. Note: All the classes in image 1 has been implemented, just covert Image 2 UML diagram to code Include a small explanation about how you propose to solve the problem and write your java code Image 1- A class diagram for our simple existing parking application Image 2 – A class diagram for our parking service and a sequence diagram for our customer command *** The second image contains two pictures side by side, the one on the left is parking service the one on the right is the customer command *** Let me know if you need additional information to solve the problem. Thank You!!!!!! Here is a java code for our parking office class. public class ParkingOffice { String name; String address; String phone; String parkingOfficeName; List customers; List cars; List lots; List charges; public ParkingOffice(){ customers = new ArrayList<>(); cars = new ArrayList<>(); lots = new ArrayList<>(); charges = new ArrayList<>(); } public Customer register() { Customer cust = new Customer(name,address,phone); customers.add(cust); return cust; } public Car register(Customer c,String licence, CarType t) { Car car = new Car(c,licence,t); cars.add(car); return car; } public Customer getCustomer(String name) { for(Customer cust : customers) if(cust.getName().equals(name)) return cust; return null; } public double addCharge(ParkingCharge p) { charges.add(p); return p.amount; } public String[] getCustomerIds(){ String[] stringArray1 = new String[2]; for(int i=0;i<2;i++){ stringArray1[i] = customers.get(i).customerId; } return stringArray1; } public String [] getPermitID () { String[] stringArray2 = new String[1]; for(int i=0;i<1;i++){ stringArray2[i] = charges.get(i).permitID; } return stringArray2; } public List getPermitIds(Customer c){ List permitid = new ArrayList<>(); for(Car car:cars){ if(car.owner == c.customerId) permitid.add(car.permit); } return permitid; } public String getParkingOfficeName(){ return this.parkingOfficeName; } /* Implement the desired park function */ public String park(Date date,String ParkingPermit,ParkingLot pl){ /* to have the amount paid during the transaction for the specified parking lot */ double amount=pl.amount; /* to have the date on which the parking lot is booked */ String onDate=date.toString(); /* to know the permit id */ String permitId=ParkingPermit; /* to print the transaction receipt */ String s="Amount paid on date: "; s=s+onDate; s=s+" is "+Double.toString(amount); s=s+"\nDear customer, your transaction for vehicle with permit ID, "; s=s+permitId; s=s+" is successful!!"; return s; } public double getParkingCharges (ParkingCharge p ){ if(p.numofHours <= 3) { p.amount= 2; } else if(p.numofHours> 3 && p.numofHours <= 19){ p.amount = 2.0 + 0.5*(p.numofHours - 3); } else if (p.numofHours > 19) { p.amount = 10; return p.amount ; } return p.amount; }
Note - Give a step-by-step and brief explanation for your solution.
To develop a user interface for our already-existing parking management system (class diagram included below – image 1), while ensuring that our code is loosely coupled, we need to provide a layer on top of the ParkingOffice class that will accept commands and send them on. This layer will be the ParkingService (class diagram included below- image 2)
For simplicity, we’ll represent the commands and their arguments as strings. We must implement the following commands:
- CUSTOMER - register a customer, along with the appropriate information, with the parking office. The sequence diagram for this command is shown below (Image 2).
- CAR - register a car with the parking office and provide a permit for the car. The sequence diagram is very similar to that of the CUSTOMER registration.
Based on the diagram below use java code to create the following classes and interfaces and implement the behavior described in the sequence diagram for both car and customer commands:
- ParkingService
- Command
- RegisterCarCommand
- RegisterCustomerCommand
We have already implemented the classes in Image 1 (car, customer, parking office, parking charge, parking lot, and money). We just need the code for the above four. Use the UML diagram and generate your code.
Note: All the classes in image 1 has been implemented, just covert Image 2 UML diagram to code
Include a small explanation about how you propose to solve the problem and write your java code
Image 1- A class diagram for our simple existing parking application
Image 2 – A class diagram for our parking service and a sequence diagram for our customer command
*** The second image contains two pictures side by side, the one on the left is parking service the one on the right is the customer command ***
Let me know if you need additional information to solve the problem. Thank You!!!!!!
Here is a java code for our parking office class.
public class ParkingOffice {
String name;
String address;
String phone;
String parkingOfficeName;
List<Customer> customers;
List<Car> cars;
List<ParkingLot> lots;
List<ParkingCharge> charges;
public ParkingOffice(){
customers = new ArrayList<>();
cars = new ArrayList<>();
lots = new ArrayList<>();
charges = new ArrayList<>();
}
public Customer register() {
Customer cust = new Customer(name,address,phone);
customers.add(cust);
return cust;
}
public Car register(Customer c,String licence, CarType t) {
Car car = new Car(c,licence,t);
cars.add(car);
return car;
}
public Customer getCustomer(String name) {
for(Customer cust : customers)
if(cust.getName().equals(name))
return cust;
return null;
}
public double addCharge(ParkingCharge p) {
charges.add(p);
return p.amount;
}
public String[] getCustomerIds(){
String[] stringArray1 = new String[2];
for(int i=0;i<2;i++){
stringArray1[i] = customers.get(i).customerId;
}
return stringArray1;
}
public String [] getPermitID () {
String[] stringArray2 = new String[1];
for(int i=0;i<1;i++){
stringArray2[i] = charges.get(i).permitID;
}
return stringArray2;
}
public List<String> getPermitIds(Customer c){
List<String> permitid = new ArrayList<>();
for(Car car:cars){
if(car.owner == c.customerId)
permitid.add(car.permit);
}
return permitid;
}
public String getParkingOfficeName(){
return this.parkingOfficeName;
}
/* Implement the desired park function */
public String park(Date date,String ParkingPermit,ParkingLot pl){
/* to have the amount paid during the transaction for
the specified parking lot */
double amount=pl.amount;
/* to have the date on which the parking lot is booked */
String onDate=date.toString();
/* to know the permit id */
String permitId=ParkingPermit;
/* to print the transaction receipt */
String s="Amount paid on date: ";
s=s+onDate;
s=s+" is "+Double.toString(amount);
s=s+"\nDear customer, your transaction for vehicle with permit ID, ";
s=s+permitId;
s=s+" is successful!!";
return s;
}
public double getParkingCharges (ParkingCharge p ){
if(p.numofHours <= 3) {
p.amount= 2;
}
else if(p.numofHours> 3 && p.numofHours <= 19){
p.amount = 2.0 + 0.5*(p.numofHours - 3);
}
else if (p.numofHours > 19) {
p.amount = 10;
return p.amount ;
}
return p.amount;
}
}
![ParkingOffice
ParkingService
service:ParkingService
Customer
office:ParkingOffice
office: ParkingOffice
commands: Map<String,Command>
+register(Customer): String - customer id
+register(Car): String - parking permit id
- register(command): void
performCommand("CUSTOMER", args)
checkParameters(args)
+performCommand(String, String[] parameters): String
•..
create(parameters)
*customer
RegisterCarCommand
RegisterCustomerCommand
register(customer)
office: ParkingOffice
office: ParkingOffice
- checkParameters(Properties): void
- checkParameters(Properties): void
+execute(Properties): String
+execute(Properties): String
List of changes:
1) Command parameters in an array, not List
Command
getCommandName(): String
getDisplayName(): String
execute(Properties params): String](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0148e73e-0d28-4d98-a412-189d898de2e8%2Fa274e024-1550-4fc6-b592-43def3dc8c19%2F5yd4q1n_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

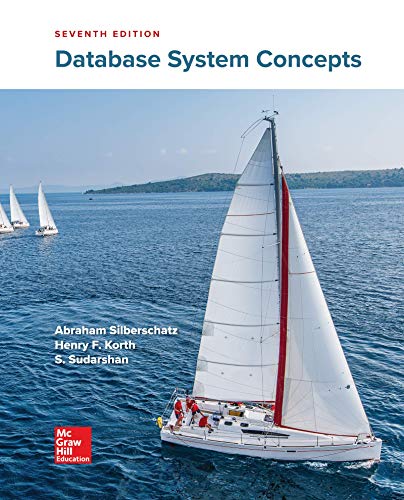
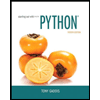
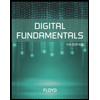
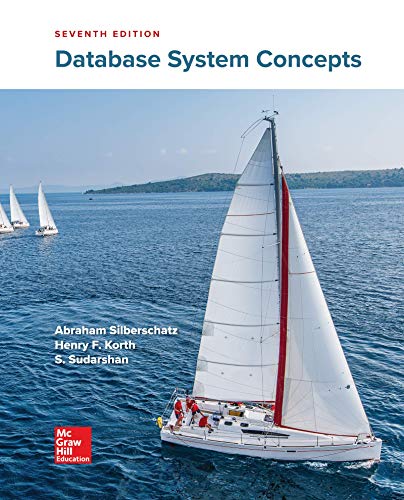
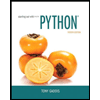
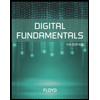
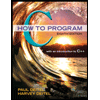
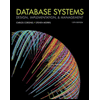
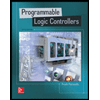