Next.js Web API Create a words-app directory then create a Next.js 13.3 application inside words-app that implements the following API to manage multiple collections of words. 1. Data is provided to you in words.json. The file contains an array of almost every word in the (American) English language. Copy that file to words-app/data/words.json. 2. Add an endpoint to search for a certain number of words using a query text. The number of matching words is selected at random and returned. Return 10 words when the number of words is not provided. You should return at most 100 words per request. 3. Add an endpoint to read all collections. 4. Add an endpoint to read all words in a collection, add a word to a collection, and delete a word from a collection. 5. You should validate all parameters provided by the user to the API and respond with an error (4xx) for invalid requests, such as an invalid parameter value, attempting to delete a non-empty collection, or attempting to delete a word from a collection that does not contain it. 6. You should handle all server errors and respond with an error status and message (500). 7. You should respond with correct status codes for successful requests (2xx). [ { name: collection_0, words: [word_0_0, word_0_1, ..., word_0_m] }, { name: collection_1, words: [word_1_0, word_1_1, ..., word_1_n] }, I did this steps, and wrote this code; // Import necessary modules and setup the Express app const express = require('express'); const fs = require('fs'); const app = express(); const wordsDataPath = './words-app/data/words.json'; const collectionsDataPath = './words-app/data/collections.json'; const PORT = process.env.PORT || 3000; // Endpoint to search for a certain number of words using a query text app.get('/api/words/:query', (req, res) => { let query = req.params.query; let count = req.query.count || 10; count = count > 100 ? 100 : count; // Limit to 100 words per request let words = fs.readFileSync(wordsDataPath); words = JSON.parse(words); let matchingWords = words.filter(word => word.includes(query)); let selectedWords = []; for (let i = 0; i < count && matchingWords.length > 0; i++) { let index = Math.floor(Math.random() * matchingWords.length); selectedWords.push(matchingWords[index]); matchingWords.splice(index, 1); } res.send(selectedWords); }); // Endpoint to read all collections app.get('/api/collections', (req, res) => { let collections = fs.readFileSync(collectionsDataPath); collections = JSON.parse(collections); res.send(collections); }); // Endpoint to create a new collection app.post('/api/collections', (req, res) => { let collections = fs.readFileSync(collectionsDataPath); collections = JSON.parse(collections); let newCollectionName = req.body.name; collections[newCollectionName] = []; fs.writeFileSync(collectionsDataPath, JSON.stringify(collections)); res.sendStatus(200); }); // Endpoint to get all words in a collection app.get('/api/collections/:name', (req, res) => { let collections = fs.readFileSync(collectionsDataPath); collections = JSON.parse(collections); let collectionName = req.params.name; let collection = collections[collectionName]; res.send(collection); }); // Endpoint to delete an empty collection app.delete('/api/collections/:name', (req, res) => { let collections = fs.readFileSync(collectionsDataPath); collections = JSON.parse(collections); let collectionName = req.params.name; if (collections[collectionName].length === 0) { delete collections[collectionName]; fs.writeFileSync(collectionsDataPath, JSON.stringify(collections)); res.sendStatus(200); } else { res.sendStatus(400); } }); // Endpoint to add a word to a collection app.post('/api/collections/:name/:word', (req, res) => { let collections = fs.readFileSync(collectionsDataPath); collections = JSON.parse(collections); let collectionName = req.params.name; let word = req.params.word; collections[collectionName].push(word); fs.writeFileSync(collectionsDataPath, JSON.stringify(collections)); res.sendStatus(200); }); // Endpoint to delete a word from a collection app.delete('/api/collections/:name/:word', (req, res) => { let collections = fs.readFileSync(collectionsDataPath); collections = JSON.parse(collections); let collectionName = req.params.name; let word = req.params.word; let index = collections[collectionName].indexOf(word); if (index > -1) { collections[collectionName].splice(index, 1); fs.writeFileSync(collectionsDataPath, JSON.stringify(collections)); res.sendStatus(200); } else { res.sendStatus(400); } }); // Start the server app.listen(PORT, () => { console.log(`server listening on port ${PORT}`); }); Need to do this; Testing using Postman Test every route and handler in the API using Postman then export the Postman collection of tests as postman.json and commit it along with your solution.
Next.js Web API
Create a words-app directory then create a Next.js 13.3 application inside words-app that implements the following API to manage multiple collections of words.
1. Data is provided to you in words.json. The file contains an array of almost every word in the (American) English language. Copy that file to words-app/data/words.json.
2. Add an endpoint to search for a certain number of words using a query text. The number of matching words is selected at random and returned. Return 10 words when the number of words is not provided. You should return at most 100 words per request.
3. Add an endpoint to read all collections.
4. Add an endpoint to read all words in a collection, add a word to a collection, and delete a word from a collection.
5. You should validate all parameters provided by the user to the API and respond with an error (4xx) for invalid requests, such as an invalid parameter value, attempting to delete a non-empty collection, or attempting to delete a word from a collection that does not contain it.
6. You should handle all server errors and respond with an error status and message (500).
7. You should respond with correct status codes for successful requests (2xx).
[
{ name: collection_0, words: [word_0_0, word_0_1, ..., word_0_m] }, { name: collection_1, words: [word_1_0, word_1_1, ..., word_1_n] },
I did this steps, and wrote this code;
// Import necessary modules and setup the Express app
const express = require('express');
const fs = require('fs');
const app = express();
const wordsDataPath = './words-app/data/words.json';
const collectionsDataPath = './words-app/data/collections.json';
const PORT = process.env.PORT || 3000;
// Endpoint to search for a certain number of words using a query text
app.get('/api/words/:query', (req, res) => {
let query = req.params.query;
let count = req.query.count || 10;
count = count > 100 ? 100 : count; // Limit to 100 words per request
let words = fs.readFileSync(wordsDataPath);
words = JSON.parse(words);
let matchingWords = words.filter(word => word.includes(query));
let selectedWords = [];
for (let i = 0; i < count && matchingWords.length > 0; i++) {
let index = Math.floor(Math.random() * matchingWords.length);
selectedWords.push(matchingWords[index]);
matchingWords.splice(index, 1);
}
res.send(selectedWords);
});
// Endpoint to read all collections
app.get('/api/collections', (req, res) => {
let collections = fs.readFileSync(collectionsDataPath);
collections = JSON.parse(collections);
res.send(collections);
});
// Endpoint to create a new collection
app.post('/api/collections', (req, res) => {
let collections = fs.readFileSync(collectionsDataPath);
collections = JSON.parse(collections);
let newCollectionName = req.body.name;
collections[newCollectionName] = [];
fs.writeFileSync(collectionsDataPath, JSON.stringify(collections));
res.sendStatus(200);
});
// Endpoint to get all words in a collection
app.get('/api/collections/:name', (req, res) => {
let collections = fs.readFileSync(collectionsDataPath);
collections = JSON.parse(collections);
let collectionName = req.params.name;
let collection = collections[collectionName];
res.send(collection);
});
// Endpoint to delete an empty collection
app.delete('/api/collections/:name', (req, res) => {
let collections = fs.readFileSync(collectionsDataPath);
collections = JSON.parse(collections);
let collectionName = req.params.name;
if (collections[collectionName].length === 0) {
delete collections[collectionName];
fs.writeFileSync(collectionsDataPath, JSON.stringify(collections));
res.sendStatus(200);
} else {
res.sendStatus(400);
}
});
// Endpoint to add a word to a collection
app.post('/api/collections/:name/:word', (req, res) => {
let collections = fs.readFileSync(collectionsDataPath);
collections = JSON.parse(collections);
let collectionName = req.params.name;
let word = req.params.word;
collections[collectionName].push(word);
fs.writeFileSync(collectionsDataPath, JSON.stringify(collections));
res.sendStatus(200);
});
// Endpoint to delete a word from a collection
app.delete('/api/collections/:name/:word', (req, res) => {
let collections = fs.readFileSync(collectionsDataPath);
collections = JSON.parse(collections);
let collectionName = req.params.name;
let word = req.params.word;
let index = collections[collectionName].indexOf(word);
if (index > -1) {
collections[collectionName].splice(index, 1);
fs.writeFileSync(collectionsDataPath,
JSON.stringify(collections)); res.sendStatus(200); } else { res.sendStatus(400); } });
// Start the server app.listen(PORT, () => { console.log(`server listening on port ${PORT}`);
});
Need to do this;
Testing using Postman
Test every route and handler in the API using Postman then export the Postman collection of tests as postman.json and commit it along with your solution.


Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

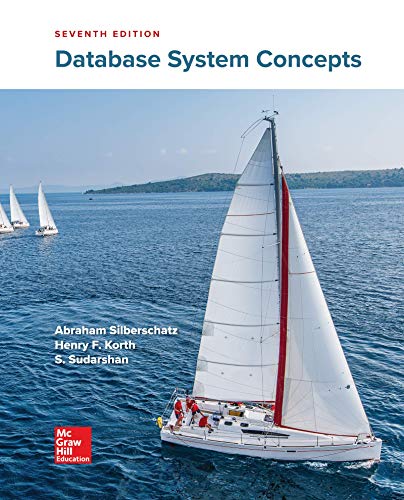
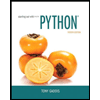
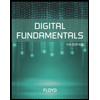
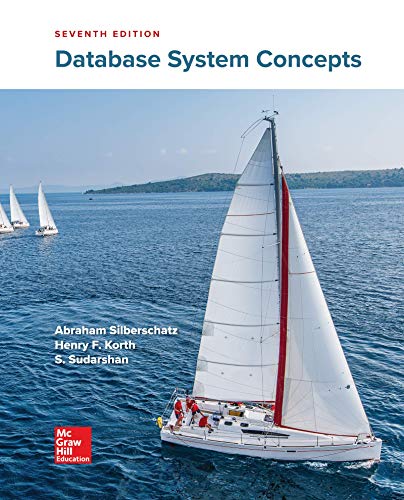
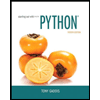
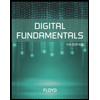
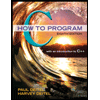
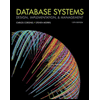
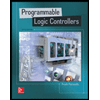