#DB File import sqlite3 import sys import os from contextlib import closing from Objects import Player, Lineup conn = None def connect(): global conn if not conn: if sys.platform =="win32": DB_FILE = "player_db.sqlite" else: HOME = os.environ["HOME"] DB_FILE = HOME + "player_db.sqlite" conn = sqlite3.connect(DB_FILE) conn.row_factory = sqlite3.Row def close(): if conn: conn.close() def get_players(): query = '''SELECT * FROM Player;''' with closing(conn.cursor()) as c: c.execute(query) results = c.fetchall() player = Player(playerID, batOrder, first, last, position, at_bats, hits) for row in results: for player in row: lineup = Lineup.add(Player(player)) return lineup # SQL statement to select all 7 fields for all players # Use a with statement to execute the query # Create a lineup object # use a loop to populate the lineup object with player objects # return the lineup object def get_player(playerID): query = '''SELECT * FROM Player WHERE playerID = ?;''' with closing(conn.cursor()) as c: c.execute(query, playerID) results = c.fetchall() for row in results: player = Player(playerID, batOrder, first, last, position, at_bats, hits) return player # SQL statement to select all 7 fields for a player # Use a with statement to execute the query & return a player object if the player exists def add_player(player): query = '''INSERT INTO Player VALUES (?, ?, ?, ?, ?, ?);''' with closing(conn.cursor()) as c: c.execute(query, batOrder, first, last, position, at_bats, hits) conn.commit() # SQL statement to insert 6 fields for a player added to the table # Use a with statement to execute the query def delete_player(player): query = '''DELETE FROM Player WHERE playerID = ?''' with closing(conn.cursor()) as c: c.execute(query, player) conn.commit() # SQL statement to delete a single player # Use a with statement to execute the query def update_bat_order(lineup): query = '''SELECT * FROM Player UPDATE Player SET batOrder = playerID;''' with closing(conn.cursor()) as c: c.execute(query) conn.commit() # Use a loop to call a SQL statement that updates # the batOrder for each player based on their playerID # Use a with statement to execute the query def update_player(player): query = '''UPDATE Player SET firstName = first, lastName = last, position = position, at_bats = at_bats, hits = hits WHERE playerID = ?;''' with closing(conn.cursor()) as c: c.execute(query) conn.commit() # SQL statement to update 6 fields of a player based on the playerID # Use a with statement to execute the query def main(): # code to test the get_players function connect() players = get_players() if players != None: for player in players: print(player.batOrder, player.firstName, player.lastName, player.position, player.atBats, player.hits, player.getBattingAvg()) else: print("Code is needed for the get_players function.") if __name__ == "__main__": main() #Objects File #player class class Player: def __init__(self, playerID, batOrder, first, last, position, at_bats, hits): self.playerID = playerID self.batOrder = batOrder self.first = first self.last = last self.position = position self.at_bats = at_bats self.hits = hits self.avg = (self.hits / self.at_bats) def name(self): return self.first + " " + self.last def average(self): return self.avg def __str__(self): return (self.name()).ljust(25) + str(self.position).rjust(2) + " " \ + str(self.at_bats).rjust(3) + " " + str(self.hits).rjust(3) + " " \ + f"{self.average():1.3f}" class Lineup: def __init__(self): self.__data = [] # add method def add(self, player): if isinstance(player, Player): self.__data.append(player) else: print("Invalid object!") # remove method def remove(self, location): if location <= len(self.__data): del self.__data[location - 1] else: print("Invalid line up location") def get(self, location): if location <= len(self.__data) + 1: return self.__data[location - 1] else: print("Invalid line up location") def __iter__(self): self.index = 0 return self def __next__(self): if self.index == len(self.__data): raise StopIteration else: self.index += 1 return self.__data[self.index - 1] # help with finish please i need for tonight
#DB File
import sqlite3
import sys
import os
from contextlib import closing
from Objects import Player, Lineup
conn = None
def connect():
global conn
if not conn:
if sys.platform =="win32":
DB_FILE = "player_db.sqlite"
else:
HOME = os.environ["HOME"]
DB_FILE = HOME + "player_db.sqlite"
conn = sqlite3.connect(DB_FILE)
conn.row_factory = sqlite3.Row
def close():
if conn:
conn.close()
def get_players():
query = '''SELECT * FROM Player;'''
with closing(conn.cursor()) as c:
c.execute(query)
results = c.fetchall()
player = Player(playerID, batOrder, first, last, position, at_bats, hits)
for row in results:
for player in row:
lineup = Lineup.add(Player(player))
return lineup
# SQL statement to select all 7 fields for all players
# Use a with statement to execute the query
# Create a lineup object
# use a loop to populate the lineup object with player objects
# return the lineup object
def get_player(playerID):
query = '''SELECT * FROM Player
WHERE playerID = ?;'''
with closing(conn.cursor()) as c:
c.execute(query, playerID)
results = c.fetchall()
for row in results:
player = Player(playerID, batOrder, first, last, position, at_bats, hits)
return player
# SQL statement to select all 7 fields for a player
# Use a with statement to execute the query & return a player object if the player exists
def add_player(player):
query = '''INSERT INTO Player
VALUES (?, ?, ?, ?, ?, ?);'''
with closing(conn.cursor()) as c:
c.execute(query, batOrder, first, last, position, at_bats, hits)
conn.commit()
# SQL statement to insert 6 fields for a player added to the table
# Use a with statement to execute the query
def delete_player(player):
query = '''DELETE FROM Player
WHERE playerID = ?'''
with closing(conn.cursor()) as c:
c.execute(query, player)
conn.commit()
# SQL statement to delete a single player
# Use a with statement to execute the query
def update_bat_order(lineup):
query = '''SELECT * FROM Player
UPDATE Player
SET batOrder = playerID;'''
with closing(conn.cursor()) as c:
c.execute(query)
conn.commit()
# Use a loop to call a SQL statement that updates
# the batOrder for each player based on their playerID
# Use a with statement to execute the query
def update_player(player):
query = '''UPDATE Player
SET firstName = first,
lastName = last,
position = position,
at_bats = at_bats,
hits = hits
WHERE playerID = ?;'''
with closing(conn.cursor()) as c:
c.execute(query)
conn.commit()
# SQL statement to update 6 fields of a player based on the playerID
# Use a with statement to execute the query
def main():
# code to test the get_players function
connect()
players = get_players()
if players != None:
for player in players:
print(player.batOrder, player.firstName, player.lastName,
player.position, player.atBats, player.hits, player.getBattingAvg())
else:
print("Code is needed for the get_players function.")
if __name__ == "__main__":
main()
#Objects File
#player class
class Player:
def __init__(self, playerID, batOrder, first, last, position, at_bats, hits):
self.playerID = playerID
self.batOrder = batOrder
self.first = first
self.last = last
self.position = position
self.at_bats = at_bats
self.hits = hits
self.avg = (self.hits / self.at_bats)
def name(self):
return self.first + " " + self.last
def average(self):
return self.avg
def __str__(self):
return (self.name()).ljust(25) + str(self.position).rjust(2) + " " \
+ str(self.at_bats).rjust(3) + " " + str(self.hits).rjust(3) + " " \
+ f"{self.average():1.3f}"
class Lineup:
def __init__(self):
self.__data = []
# add method
def add(self, player):
if isinstance(player, Player):
self.__data.append(player)
else:
print("Invalid object!")
# remove method
def remove(self, location):
if location <= len(self.__data):
del self.__data[location - 1]
else:
print("Invalid line up location")
def get(self, location):
if location <= len(self.__data) + 1:
return self.__data[location - 1]
else:
print("Invalid line up location")
def __iter__(self):
self.index = 0
return self
def __next__(self):
if self.index == len(self.__data):
raise StopIteration
else:
self.index += 1
return self.__data[self.index - 1]
# help with finish please i need for tonight


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

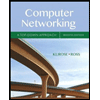
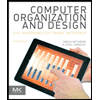
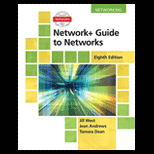
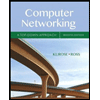
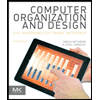
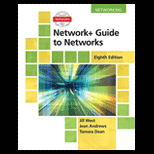
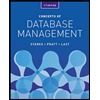
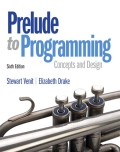
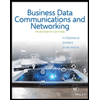