Need help writing the following method: get(int id) – returns a Student, assuming that the student is either registered for the course or on the waitlist. If there is no student in the table or waitlist that has this id, get() should return null. You should go directly to slot id % m rather than iterating through all the slots. For context public class Course { public String code; public int capacity; public SLinkedList[] studentTable; public int size; public SLinkedList waitlist; public Course(String code) { this.code = code; this.studentTable = new SLinkedList[10]; this.size = 0; this.waitlist = new SLinkedList(); this.capacity = 10; } public Course(String code, int capacity) { this.code = code; this.studentTable = new SLinkedList[capacity]; this.size = 0; this.waitlist = new SLinkedList<>(); this.capacity = capacity; }
Need help writing the following method: get(int id)
– returns a Student, assuming that the student is either registered for the course or on the waitlist. If there is no student in the table or waitlist that has this id, get() should return null. You should go directly to slot id % m rather than iterating through all the slots.
For context
public class Course {
public String code;
public int capacity;
public SLinkedList<Student>[] studentTable;
public int size;
public SLinkedList<Student> waitlist;
public Course(String code) {
this.code = code;
this.studentTable = new SLinkedList[10];
this.size = 0;
this.waitlist = new SLinkedList<Student>();
this.capacity = 10;
}
public Course(String code, int capacity) {
this.code = code;
this.studentTable = new SLinkedList[capacity];
this.size = 0;
this.waitlist = new SLinkedList<>();
this.capacity = capacity;
}


Step by step
Solved in 2 steps

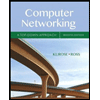
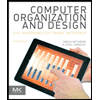
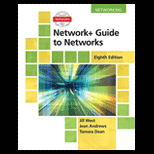
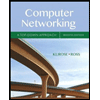
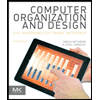
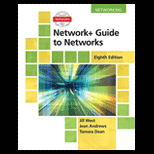
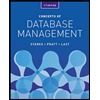
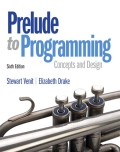
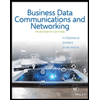