Need help understanding each step of the following code. This is the prompt: In c programming capitalize the first occurrence of each vowel (a,e,i,o,u) . For example apple = ApplE; I also want to understand how to start an assignment like this in the future. #include #include #include // Return a newly allocated copy of original // with the first occurrence of each vowel capitalized char* CapVowels(char* original) { int a = 1, e = 1, i = 1, o = 1, u = 1; int count = 0; char c; while (original[count]) ++count; char* modified = (char *) malloc(sizeof(char) * (1+count)); for (int j=0; j
Need help understanding each step of the following code. This is the prompt: In c programming capitalize the first occurrence of each vowel (a,e,i,o,u) . For example apple = ApplE; I also want to understand how to start an assignment like this in the future.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// Return a newly allocated copy of original
// with the first occurrence of each vowel capitalized
char* CapVowels(char* original) {
int a = 1, e = 1, i = 1, o = 1, u = 1;
int count = 0;
char c;
while (original[count]) ++count;
char* modified = (char *) malloc(sizeof(char) * (1+count));
for (int j=0; j<count; j++) {
c =original[j];
if (c =='a' || c == 'A') {
if (a ==1){
a =0;
c='A';
}
}else if (c =='e' || c == 'E') {
if (e ==1){
e =0;
c='E';
}
}else if (c =='i' || c == 'I') {
if (i ==1){
i =0;
c='I';
}
}else if (c =='o' || c == 'O') {
if (o ==1){
o =0;
c ='O';
}
}else if (c =='u' || c == 'U') {
if (u ==1){
u =0;
c ='U';
}
}
modified[j] = c;
}
modified[count] ='\0';
return modified;
}
int main(void) {
char userCaption[50];
char* resultStr;
scanf("%s", userCaption);
resultStr = CapVowels(userCaption);
printf("Original: %s\n", userCaption);
printf("Modified: %s\n", resultStr);
// Always free dynamically allocated memory when no longer needed
free(resultStr);
return 0;
}

Step by step
Solved in 3 steps with 4 images

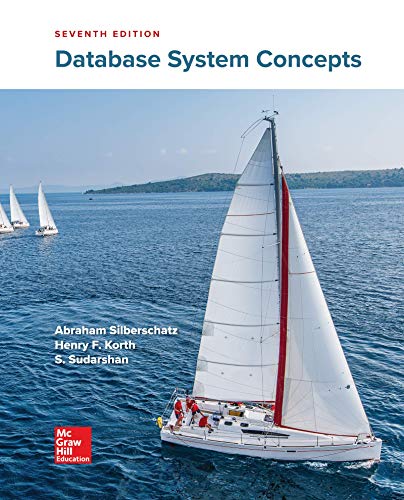
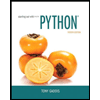
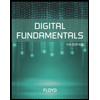
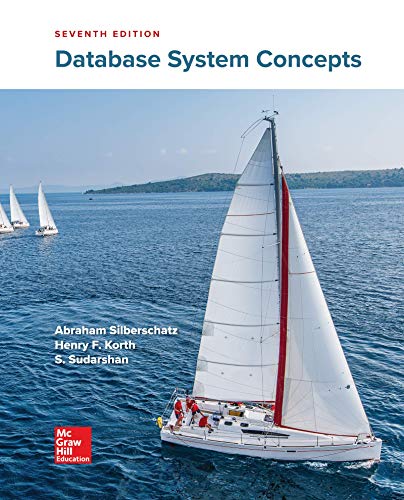
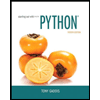
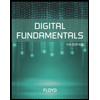
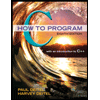
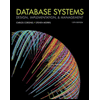
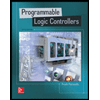