Need Help fixing my code!! only under TODO's. // Util function to convert a FoodWastageReport class object into a serialized // JSON object. crow::json::wvalue FoodWastageReportToCrowJSON( const FoodWastageReport &report) { crow::json::wvalue report_json({}); std::vector most_common_disposal_mechanisms{}; // TODO 1: Call the member function of FoodWastageReport class that returns // all the most common disposal mechanisms as a vector of strings. Store the // result in the vector declared above. FoodWastageReport report; std::vector most_common_disposal_mechanisms = report.MostCommonDisposalMechanisms(); report_json["most_common_disposal_mechanism_"] = most_common_disposal_mechanisms; std::vector most_commonly_wasted_foods{}; // TODO 2: Call the member function of FoodWastageReport class that returns // all the most commonly wasted foods as a vector of strings. Store the result // in the vector declared above. std::vector most_commonly_wasted_foods = report.MostCommonlyWastedFoods(); report_json["most_commonly_wasted_food_"] = most_commonly_wasted_foods; std::vector most_common_wastage_reasons{}; // TODO 3: Call the member function of FoodWastageReport class that returns // all the most commonwastage reasons as a vector of strings. Store the result // in the vector declared above. std::vector most_common_wastage_reasons = report.MostCommonWastageReasons(); report_json["most_common_wastage_reason_"] = most_common_wastage_reasons; std::vector most_costly_waste_producing_meals{}; // TODO 4: Call the member function of FoodWastageReport class that returns // all the most costly waste producing meals as a vector of strings. Store the // result in the vector declared above. std::vector most_costly_waste_producing_meals = report.MostCostlyWasteProducingMeals(); report_json["most_waste_producing_meal_"] = most_costly_waste_producing_meals; std::vector suggested_strategies_to_reduce_waste{}; // TODO 5: Call the member function of FoodWastageReport class that returns // all the suggested strategies as a vector of strings. Store the result in // the vector declared above. std::vector suggested_strategies_to_reduce_waste = report.SuggestWasteReductionStrategies(); report_json["suggested_strategies_to_reduce_waste_"] = suggested_strategies_to_reduce_waste; double total_cost_of_wastage = -9999.0;
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
Need Help fixing my code!! only under TODO's.
// Util function to convert a FoodWastageReport class object into a serialized
// JSON object.
crow::json::wvalue FoodWastageReportToCrowJSON(
const FoodWastageReport &report) {
crow::json::wvalue report_json({});
std::
// TODO 1: Call the member function of FoodWastageReport class that returns
// all the most common disposal mechanisms as a vector of strings. Store the
// result in the vector declared above.
FoodWastageReport report;
std::vector<std::string> most_common_disposal_mechanisms = report.MostCommonDisposalMechanisms();
report_json["most_common_disposal_mechanism_"] =
most_common_disposal_mechanisms;
std::vector<std::string> most_commonly_wasted_foods{};
// TODO 2: Call the member function of FoodWastageReport class that returns
// all the most commonly wasted foods as a vector of strings. Store the result
// in the vector declared above.
std::vector<std::string> most_commonly_wasted_foods = report.MostCommonlyWastedFoods();
report_json["most_commonly_wasted_food_"] = most_commonly_wasted_foods;
std::vector<std::string> most_common_wastage_reasons{};
// TODO 3: Call the member function of FoodWastageReport class that returns
// all the most commonwastage reasons as a vector of strings. Store the result
// in the vector declared above.
std::vector<std::string> most_common_wastage_reasons = report.MostCommonWastageReasons();
report_json["most_common_wastage_reason_"] = most_common_wastage_reasons;
std::vector<std::string> most_costly_waste_producing_meals{};
// TODO 4: Call the member function of FoodWastageReport class that returns
// all the most costly waste producing meals as a vector of strings. Store the
// result in the vector declared above.
std::vector<std::string> most_costly_waste_producing_meals = report.MostCostlyWasteProducingMeals();
report_json["most_waste_producing_meal_"] = most_costly_waste_producing_meals;
std::vector<std::string> suggested_strategies_to_reduce_waste{};
// TODO 5: Call the member function of FoodWastageReport class that returns
// all the suggested strategies as a vector of strings. Store the result in
// the vector declared above.
std::vector<std::string> suggested_strategies_to_reduce_waste = report.SuggestWasteReductionStrategies();
report_json["suggested_strategies_to_reduce_waste_"] =
suggested_strategies_to_reduce_waste;
double total_cost_of_wastage = -9999.0;
// TODO 6: Call the member function of FoodWastageReport class that returns
// the total cost of wastage as a double. Store the result in the double
// declared.
double total_cost_of_wastage = report.TotalCostOfFoodWasted();
report_json["total_cost_of_food_wasted_"] = total_cost_of_wastage;
return report_json;
}
// Util function to convert a crow query string into a FoodWastageRecord class
// object.
FoodWastageRecord QueryStringToFoodWastageRecord(
const crow::query_string &query_string) {
FoodWastageRecord record;
// TODO 1. Get the date from the query_string using query_string.get("date"),
// and set it in the `record` object using the setter in FoodWastageRecord
// class.
std::string date = query_string.get("date");
record.SetDate(date);
// TODO 2. Get the meal from the query_string using query_string.get("meal"),
// and set it in the `record` object using the setter in FoodWastageRecord
// class.
std::string meal = query_string.get("meal");
record.SetMeal(meal);
// TODO 3. Get the food name from the query_string using
// query_string.get("food_name"), and set it in the `record` object using the
// setter in FoodWastageRecord class.
std::string food_name = query_string.get("food_name");
record.SetMeal(food_name);
// TODO 4. Get the quantity from the query_string using
// std::stod(query_string.get("qty_in_oz")), and set it in the `record` object
// using the setter in FoodWastageRecord class.
double quantity = std::stod(query_string.get("qty_in_oz"));
record.SetQuantityInOz(quantity);
// TODO 5. Get the wastage reason from the query_string using
// query_string.get("wastage_reason"), and set it in the `record` object using
// the setter in FoodWastageRecord class.
std::string wastage_reason = query_string.get("wastage_reason");
record.SetWastageReason(wastage_reason);
// TODO 6. Get the disposal
// query_string.get("disposal_mechanism"), and set it in the `record` object
// using the setter in FoodWastageRecord class.
std::string disposal_mechanism = query_string.get("disposal_mechanism");
record.SetDisposalMechanism(disposal_mechanism);
// TODO 7. Get the cost from the query_string using
// std::stod(query_string.get("cost")), and set it in the `record` object
// using the setter in FoodWastageRecord class.
double cost = std::stod(query_string.get("cost"));
record.SetCost(cost);
return record;
}


Trending now
This is a popular solution!
Step by step
Solved in 4 steps

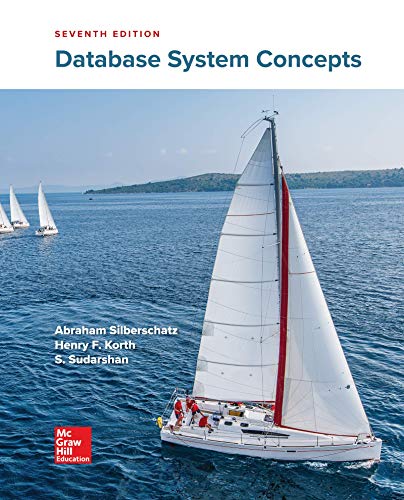
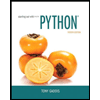
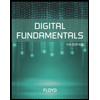
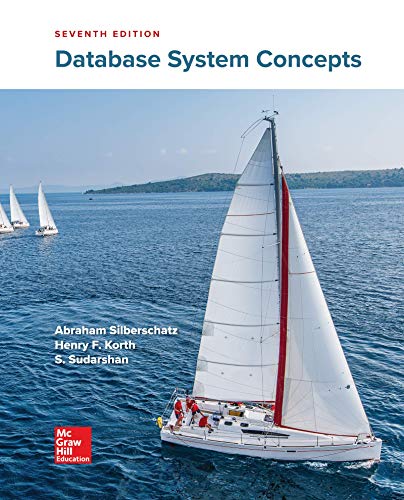
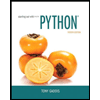
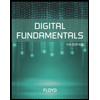
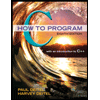
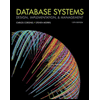
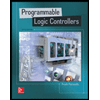