Need help creating this pseudocode into a functioning PYTHON code. Sample Pseudocode: // main module Module main() // Declare input variables Declare String inputName, String inputType, Integer inputAge // class variable to hold a pet Declare Pet Animal // create a Pet object Set Animal = new Pet() // Get values for a pet Display “Enter a pet name:” Input inputName Animal.setName(inputName) Display “Enter a pet type:” Input inputType Animal.setType(inputType) Display “Enter a pet age:” Input inputAge Animal.setAge(inputAge) // Show values for this pet Display “The pet name is “,Animal.getName() Display “The pet type is “,Animal.getType() Display “The pet age is “,Animal.getAge() End Module Class Pet // Fields Private String name Private String type Private Integer age // Constructor Public Module Pet(String n, String t, Integer a) Set name = n Set type = t Set age = a End Module // Mutators Public Module setName(String n) Set name = n End Module Public Module setType(String t) Set type = t End Module Public Module setAge(Integer a) Set age = a End Module // Accessors Public Function String getName() Return name End Function Public Function String getType() Return type End Function Public Function getAge() Return age End Function
# Need help creating this pseudocode into a functioning PYTHON code.
Sample Pseudocode:
// main module
Module main()
// Declare input variables
Declare String inputName, String inputType, Integer inputAge
// class variable to hold a pet
Declare Pet Animal
// create a Pet object
Set Animal = new Pet()
// Get values for a pet
Display “Enter a pet name:”
Input inputName
Animal.setName(inputName)
Display “Enter a pet type:”
Input inputType
Animal.setType(inputType)
Display “Enter a pet age:”
Input inputAge
Animal.setAge(inputAge)
// Show values for this pet
Display “The pet name is “,Animal.getName()
Display “The pet type is “,Animal.getType()
Display “The pet age is “,Animal.getAge()
End Module
Class Pet
// Fields
Private String name
Private String type
Private Integer age
// Constructor
Public Module Pet(String n, String t, Integer a)
Set name = n
Set type = t
Set age = a
End Module
// Mutators
Public Module setName(String n)
Set name = n
End Module
Public Module setType(String t)
Set type = t
End Module
Public Module setAge(Integer a)
Set age = a
End Module
// Accessors
Public Function String getName()
Return name
End Function
Public Function String getType()
Return type
End Function
Public Function getAge()
Return age
End Function

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

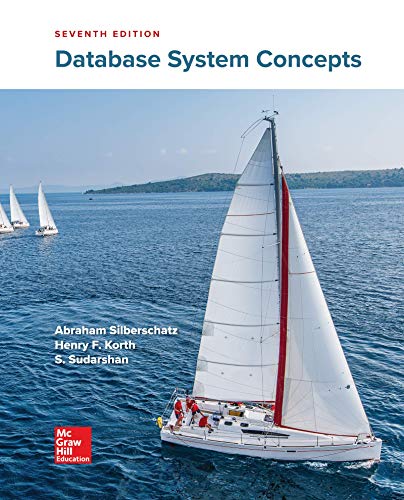
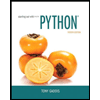
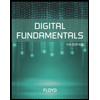
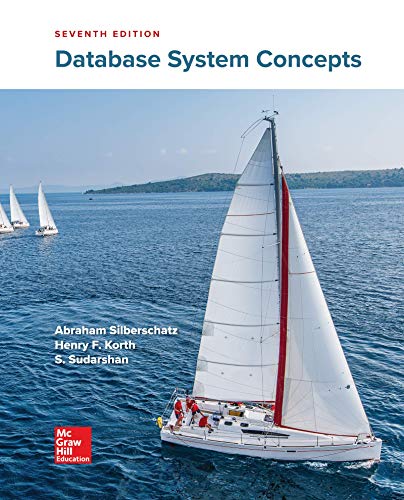
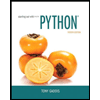
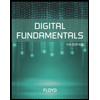
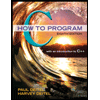
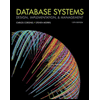
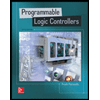