n python3!!! starter-code compare_sort_algorithms.py: import random import time maxvalue = 1000 def merge(left, right): result = [] left_idx, right_idx = 0, 0 while left_idx < len(left) and right_idx < len(right): # to change direction of sort, change direction of comparison if left[left_idx] <= right[right_idx]: result.append(left[left_idx]) left_idx += 1 else: result.append(right[right_idx]) right_idx += 1 if left: result.extend(left[left_idx:]) if right: result.extend(right[right_idx:]) return result def merge_sort(m): if len(m) <= 1: return m middle = len(m) // 2 left = m[:middle] right = m[middle:] left = merge_sort(left) right = merge_sort(right) return list(merge(left, right)) def insertion_sort(array): for slot in range(1, len(array)): value = array[slot] test_slot = slot - 1 while test_slot > -1 and array[test_slot] > value: array[test_slot + 1] = array[test_slot] test_slot = test_slot - 1 array[test_slot + 1] = value return array def bubble_sort(array): index = len(array) - 1 while index >= 0: for j in range(index): if array[j] > array[j + 1]: array[j], array[j + 1] = array[j + 1], array[j] index -= 1 return array def main(): #ADD CODE to compare the three list-sorting functions main()
In python3!!!
starter-code compare_sort_algorithms.py:
import random
import time
maxvalue = 1000
def merge(left, right):
result = []
left_idx, right_idx = 0, 0
while left_idx < len(left) and right_idx < len(right):
# to change direction of sort, change direction of comparison
if left[left_idx] <= right[right_idx]:
result.append(left[left_idx])
left_idx += 1
else:
result.append(right[right_idx])
right_idx += 1
if left:
result.extend(left[left_idx:])
if right:
result.extend(right[right_idx:])
return result
def merge_sort(m):
if len(m) <= 1:
return m
middle = len(m) // 2
left = m[:middle]
right = m[middle:]
left = merge_sort(left)
right = merge_sort(right)
return list(merge(left, right))
def insertion_sort(array):
for slot in range(1, len(array)):
value = array[slot]
test_slot = slot - 1
while test_slot > -1 and array[test_slot] > value:
array[test_slot + 1] = array[test_slot]
test_slot = test_slot - 1
array[test_slot + 1] = value
return array
def bubble_sort(array):
index = len(array) - 1
while index >= 0:
for j in range(index):
if array[j] > array[j + 1]:
array[j], array[j + 1] = array[j + 1], array[j]
index -= 1
return array
def main():
#ADD CODE to compare the three list-sorting functions
main()
![import random
import time
3
1
2
4
maxvalue
1000
6 - def merge(left, right):
7
result
left_idx, right_idx
while left_idx < len(left) and right_idx < len(right):
8
0, 0
9.
# to change direction of sort, change direction of comparison
if left[left_idx] <=
result.append(left[left_idx])
left_idx += 1
else:
result.append(right[right_idx])
right_idx += 1
10
11
right[right_idx]:
12
13
14 -
15
16
if left:
result.extend(left[left_idx:])
if right:
result.extend(right[right_idx:])
return result
17
18
19
20
21
22
def merge_sort(m):
if len(m)
23
24
<= 1:
25
return m
26
len(m) // 2
m[:middle]
m[middle:]
27
middle
28
left
29
right
30
merge_sort(left)
merge_sort(right)
return list(merge(left, right))
31
left
32
right
33
34
def insertion_sort(array):
for slot in range(1, len(array)):
value
35
36 -
37
array[slot]
38
test_slot
slot
1
while test_slot
array[test_slot + 1]
test_slot
-1 and array[test_slot] > value:
array[test_slot]
39
40
41
test_slot
- 1
42
array[test_slot + 1]
value
43
return array
44
45 - def bubble_sort(array):
46
index =
len(array)
1
47 -
while index >= 0:
48 -
49 -
for j in range(index):
if array[j] > array[j + 1]:
50
array[j], array[j + 1]
array[j + 1], array[j]
51
index
1
52
return array
53
54 - def main():
55
#ADD CODE to compare the three list-sorting functions
56 main()](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F909d4e96-ec45-4f89-986e-8cb112f8d100%2Fbf556e75-3a06-45e4-af78-cae842228580%2Fl5x5q8e_processed.png&w=3840&q=75)


Step by step
Solved in 4 steps

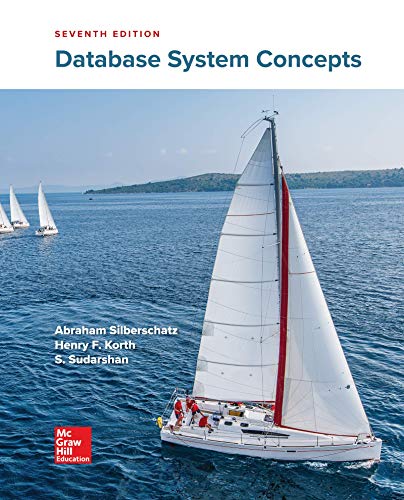
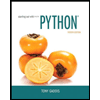
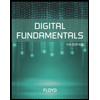
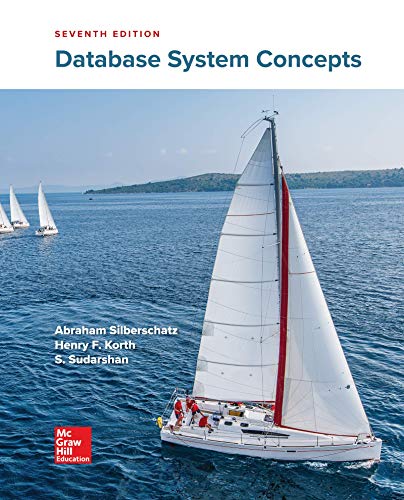
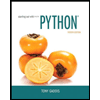
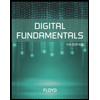
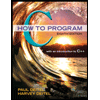
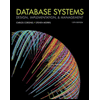
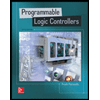