I just need the table filled out
#Searching Exercise By Antonio Rotondo
def ordered_sequential_search(a_list, item):
pos = 0
found = False
stop = False
count = 0
while pos < len(a_list) and not found and not stop:
count += 1
if a_list[pos] == item:
found = True
else:
if a_list[pos] > item:
stop = True
else:
pos = pos+1
return found, count
test_list = [0, 1, 2, 8, 13, 17, 19, 32, 42,]
print(ordered_sequential_search(test_list, 3))
print(ordered_sequential_search(test_list, 13))
def sequential_search(a_list, item):
pos = 0
found = False
count = 0
while pos < len(a_list) and not found:
count += 1
if a_list[pos] == item:
found = True
else:
pos = pos+1
return found, count
test_list = [1, 2, 32, 8, 17, 19, 42, 13, 0]
def binary_search(a_list, item, count):
if len(a_list) == 0:
return False, count
else:
midpoint = len(a_list) // 2
count+=1
if a_list[midpoint] == item:
return True, count
else:
if item < a_list[midpoint]:
return binary_search(a_list[:midpoint], item, count)
else:
return binary_search(a_list[midpoint + 1:], item,count)
test_list = [0, 1, 2, 8, 13, 17, 19, 32, 42,]
print(binary_search(test_list, 3, 0))
print(binary_search(test_list, 13, 0))
print(sequential_search(test_list, 3))
print(sequential_search(test_list, 13))
I just need the table filled out
![In_myList= [1, 2, 32, 8, 17, 19, 42, 13, 0]
Make sure to use the sorted list when calling both the Ordered Seguantial Search and the
Binary Search
Number of elements checked using
Sequential
Ordered
Binary
Sequential Search
Search
Search
Search for 3
Search for 13
Please Submit your code (.py file) together with this table (in a document file).](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa3f90e95-6ace-4455-9a40-a789a93ab131%2F74cc6676-d095-4603-8c3a-c3adfcaf3033%2Fmkp6wjr_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps

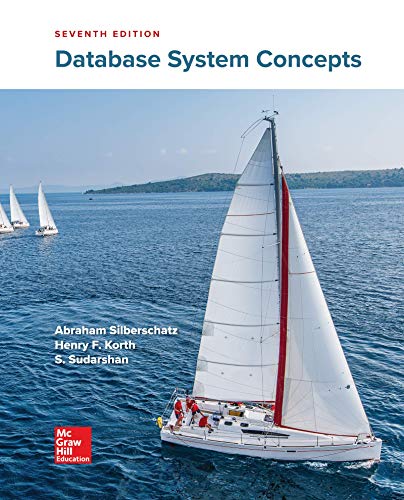
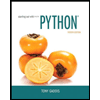
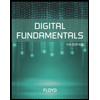
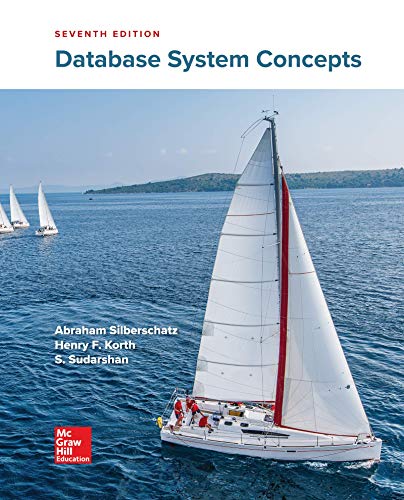
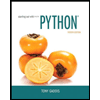
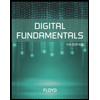
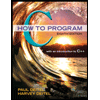
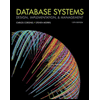
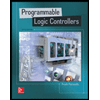