Modify the following code code so the code can -normalize the list (divide all by the maximum) - insert a bubble and merge sort and compare the counter to see which one it is the fastest sorting.
Modify the following code code so the code can
-normalize the list (divide all by the maximum)
- insert a bubble and merge sort and compare the counter to see which one it is the fastest sorting.
#include <bits/stdc++.h>
using namespace std;
int largest(int arr[], int n)
{
int i;
// Initialize maximum element
int max = arr[0];
for (i = 1; i < n; i++)
if (arr[i] > max)
max = arr[i];
return max;
}
int smallest(int arr[], int n)
{
int i;
// Initialize minimum element
int min = arr[0];
for (i = 1; i < n; i++)
if (arr[i] < min)
min = arr[i];
return min;
}
double average(int a[], int n)
{
int sum = 0;
for (int i=0; i<n; i++)
sum += a[i];
return (double)sum/n;
}
// Driver Code
int main()
{
int arr[] = {8, 9, 10, 12, 13};
int n = sizeof(arr) / sizeof(arr[0]);
cout << "Largest in given array is " << largest(arr, n);
cout << "\nSmallest in given array is " << smallest(arr, n);
cout << "\nAverage of given array is " << average(arr, n);
return 0;
}

Step by step
Solved in 2 steps with 1 images

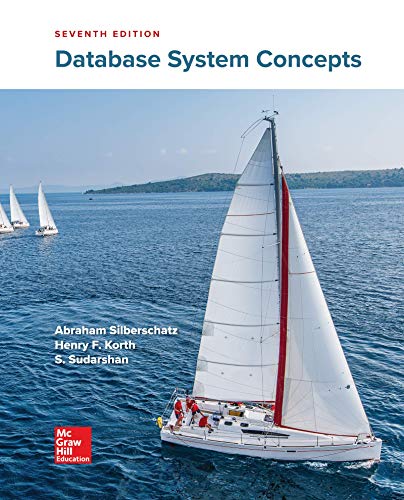
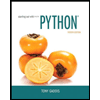
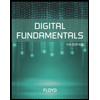
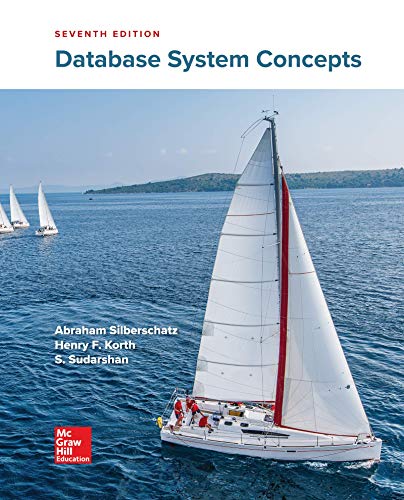
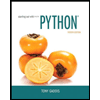
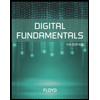
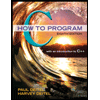
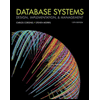
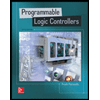