n C++ I'm trying to modify the code I've written below to check the type of the array being called from main. If the array is int or double, user should enter a number instead of a string, and then the values get added together. Otherwise, if the array is a char it will default to the concatenate code I've already written. Here is the code I have: template T MyArray::increaseElementsBy() { cout << endl << "Welcome to increaseElementsBy()" << endl; string result; stringstream ss; string toConcat; cout << "Enter something to add to the element: "; cin >> toConcat; for (int i = 0; i < 10; i++) { ss << theArray[i] << toConcat << endl; } result = ss.str(); cout << result; return 0; } If possible, it would be even better if what the user enters is also checked. If the user enters a string while the int array is being used, it should also concatenate the items together, but if they enter a string that is an int - convert the string to int and add it to the int array elements.
In C++ I'm trying to modify the code I've written below to check the type of the array being called from main. If the array is int or double, user should enter a number instead of a string, and then the values get added together. Otherwise, if the array is a char it will default to the concatenate code I've already written.
Here is the code I have:
template<typename T>
T MyArray<T>::increaseElementsBy()
{
cout << endl << "Welcome to increaseElementsBy()" << endl;
string result;
stringstream ss;
string toConcat;
cout << "Enter something to add to the element: ";
cin >> toConcat;
for (int i = 0; i < 10; i++)
{
ss << theArray[i] << toConcat << endl;
}
result = ss.str();
cout << result;
return 0;
}
If possible, it would be even better if what the user enters is also checked. If the user enters a string while the int array is being used, it should also concatenate the items together, but if they enter a string that is an int - convert the string to int and add it to the int array elements.

Step by step
Solved in 4 steps

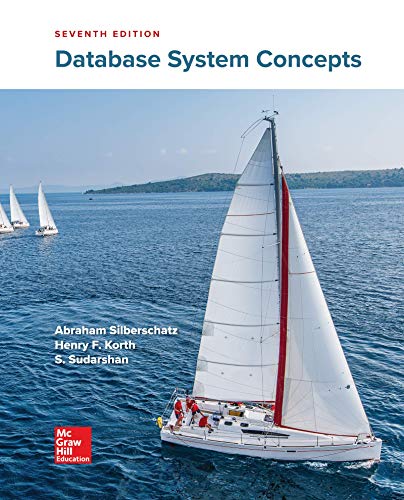
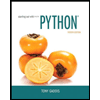
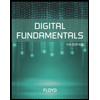
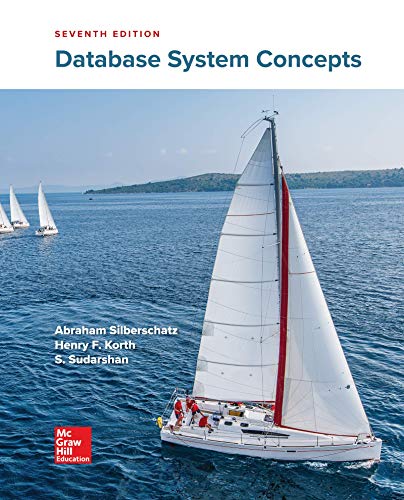
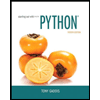
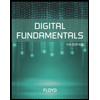
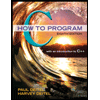
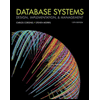
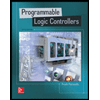