My code here is in Python and the goal is to create a BankApp where the user is suppose to enter their username and password and its suppose to show the balance but I forgot to add the information that is suppose to be implemented in my code as shown below. userName passWord Balance ======================== Mike sorat1237# 350 Jane para432@4 400 Steve asora8731% 500 Its also suppose to do down the following. Type D to deposit money Type W to withdraw money Type B to display Balance Type C to change user, display user name Type A to add new client Type E to exit My Code def read_user_information(): usernames = [] passwords = [] balances = [] try: with open("UserInformation.txt", "r") as file: lines = file.readlines() for line in lines[2:]: data = line.strip().split() usernames.append(data[0]) passwords.append(data[1]) balances.append(float(data[2])) except FileNotFoundError: with open("UserInformation.txt", "w") as file: file.write("userName passWord Balance\n") file.write("========================\n") return usernames, passwords, balances def write_user_information(usernames, passwords, balances): with open("UserInformation.txt", "w") as file: file.write("userName passWord Balance\n") file.write("========================\n") for i in range(len(usernames)): file.write(f"{usernames[i]:<12} {passwords[i]:<12} {balances[i]:<}\n") def login(usernames, passwords): username_input = input("Enter username: ") password_input = input("Enter password: ") if username_input in usernames: index = usernames.index(username_input) if password_input == passwords[index]: return index print("Invalid username or password. Please try again.") return None def deposit(balances, user_index): amount = float(input("Enter the amount to deposit: ")) balances[user_index] += amount print(f"Deposit successful. New balance: ${balances[user_index]:.2f}") def withdraw(balances, user_index): amount = float(input("Enter the amount to withdraw: ")) if amount > balances[user_index]: print("Insufficient funds. Withdrawal failed.") else: balances[user_index] -= amount print(f"Withdrawal successful. New balance: ${balances[user_index]:.2f}") def show_balance(balances, user_index): print(f"Current balance for {usernames[user_index]}: ${balances[user_index]:.2f}") def change_user(): new_user = input("Enter new username: ") return new_user def add_new_user(usernames, passwords, balances): new_username = input("Enter new username: ") new_password = input("Enter new password: ") new_balance = float(input("Enter initial balance: ")) usernames.append(new_username) passwords.append(new_password) balances.append(new_balance) print(f"New user {new_username} added with balance: ${new_balance:.2f}") def main(): global usernames # Declare usernames as a global variable usernames, passwords, balances = read_user_information() while True: user_index = login(usernames, passwords) if user_index is not None: while True: print("\nMenu:") print("Type D to deposit money") print("Type W to withdraw money") print("Type B to display balance") print("Type C to change user, display username") print("Type A to add new client") print("Type E to exit") option = input("Enter your choice: ") if option == 'D': deposit(balances, user_index) show_balance(balances, user_index) elif option == 'W': show_balance(balances, user_index) withdraw(balances, user_index) show_balance(balances, user_index) elif option == 'B': show_balance(balances, user_index) elif option == 'C': user_index = login(usernames, passwords) if user_index is not None: print(f"Switched to user: {usernames[user_index]}") elif option == 'A': add_new_user(usernames, passwords, balances) elif option == 'E': write_user_information(usernames, passwords, balances) print("Exiting program. User information updated.") return else: print("Invalid option. Please try again.") if __name__ == "__main__": main()
My code here is in Python and the goal is to create a BankApp where the user is suppose to enter their username and password and its suppose to show the balance but I forgot to add the information that is suppose to be implemented in my code as shown below. userName passWord Balance ======================== Mike sorat1237# 350 Jane para432@4 400 Steve asora8731% 500 Its also suppose to do down the following. Type D to deposit money Type W to withdraw money Type B to display Balance Type C to change user, display user name Type A to add new client Type E to exit My Code def read_user_information(): usernames = [] passwords = [] balances = [] try: with open("UserInformation.txt", "r") as file: lines = file.readlines() for line in lines[2:]: data = line.strip().split() usernames.append(data[0]) passwords.append(data[1]) balances.append(float(data[2])) except FileNotFoundError: with open("UserInformation.txt", "w") as file: file.write("userName passWord Balance\n") file.write("========================\n") return usernames, passwords, balances def write_user_information(usernames, passwords, balances): with open("UserInformation.txt", "w") as file: file.write("userName passWord Balance\n") file.write("========================\n") for i in range(len(usernames)): file.write(f"{usernames[i]:<12} {passwords[i]:<12} {balances[i]:<}\n") def login(usernames, passwords): username_input = input("Enter username: ") password_input = input("Enter password: ") if username_input in usernames: index = usernames.index(username_input) if password_input == passwords[index]: return index print("Invalid username or password. Please try again.") return None def deposit(balances, user_index): amount = float(input("Enter the amount to deposit: ")) balances[user_index] += amount print(f"Deposit successful. New balance: ${balances[user_index]:.2f}") def withdraw(balances, user_index): amount = float(input("Enter the amount to withdraw: ")) if amount > balances[user_index]: print("Insufficient funds. Withdrawal failed.") else: balances[user_index] -= amount print(f"Withdrawal successful. New balance: ${balances[user_index]:.2f}") def show_balance(balances, user_index): print(f"Current balance for {usernames[user_index]}: ${balances[user_index]:.2f}") def change_user(): new_user = input("Enter new username: ") return new_user def add_new_user(usernames, passwords, balances): new_username = input("Enter new username: ") new_password = input("Enter new password: ") new_balance = float(input("Enter initial balance: ")) usernames.append(new_username) passwords.append(new_password) balances.append(new_balance) print(f"New user {new_username} added with balance: ${new_balance:.2f}") def main(): global usernames # Declare usernames as a global variable usernames, passwords, balances = read_user_information() while True: user_index = login(usernames, passwords) if user_index is not None: while True: print("\nMenu:") print("Type D to deposit money") print("Type W to withdraw money") print("Type B to display balance") print("Type C to change user, display username") print("Type A to add new client") print("Type E to exit") option = input("Enter your choice: ") if option == 'D': deposit(balances, user_index) show_balance(balances, user_index) elif option == 'W': show_balance(balances, user_index) withdraw(balances, user_index) show_balance(balances, user_index) elif option == 'B': show_balance(balances, user_index) elif option == 'C': user_index = login(usernames, passwords) if user_index is not None: print(f"Switched to user: {usernames[user_index]}") elif option == 'A': add_new_user(usernames, passwords, balances) elif option == 'E': write_user_information(usernames, passwords, balances) print("Exiting program. User information updated.") return else: print("Invalid option. Please try again.") if __name__ == "__main__": main()
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Topic Video
Question
My code here is in Python and the goal is to create a BankApp where the user is suppose to enter their username and password and its suppose to show the balance but I forgot to add the information that is suppose to be implemented in my code as shown below.
userName passWord Balance
========================
Mike sorat1237# 350
Jane para432@4 400
Steve asora8731% 500
========================
Mike sorat1237# 350
Jane para432@4 400
Steve asora8731% 500
Its also suppose to do down the following.
Type D to deposit money
Type W to withdraw money
Type B to display Balance
Type C to change user, display user name
Type A to add new client
Type E to exit
Type W to withdraw money
Type B to display Balance
Type C to change user, display user name
Type A to add new client
Type E to exit
My Code
def read_user_information():
usernames = []
passwords = []
balances = []
try:
with open("UserInformation.txt", "r") as file:
lines = file.readlines()
for line in lines[2:]:
data = line.strip().split()
usernames.append(data[0])
passwords.append(data[1])
balances.append(float(data[2]))
except FileNotFoundError:
with open("UserInformation.txt", "w") as file:
file.write("userName passWord Balance\n")
file.write("========================\n")
return usernames, passwords, balances
def write_user_information(usernames, passwords, balances):
with open("UserInformation.txt", "w") as file:
file.write("userName passWord Balance\n")
file.write("========================\n")
for i in range(len(usernames)):
file.write(f"{usernames[i]:<12} {passwords[i]:<12} {balances[i]:<}\n")
def login(usernames, passwords):
username_input = input("Enter username: ")
password_input = input("Enter password: ")
if username_input in usernames:
index = usernames.index(username_input)
if password_input == passwords[index]:
return index
print("Invalid username or password. Please try again.")
return None
def deposit(balances, user_index):
amount = float(input("Enter the amount to deposit: "))
balances[user_index] += amount
print(f"Deposit successful. New balance: ${balances[user_index]:.2f}")
def withdraw(balances, user_index):
amount = float(input("Enter the amount to withdraw: "))
if amount > balances[user_index]:
print("Insufficient funds. Withdrawal failed.")
else:
balances[user_index] -= amount
print(f"Withdrawal successful. New balance: ${balances[user_index]:.2f}")
def show_balance(balances, user_index):
print(f"Current balance for {usernames[user_index]}: ${balances[user_index]:.2f}")
def change_user():
new_user = input("Enter new username: ")
return new_user
def add_new_user(usernames, passwords, balances):
new_username = input("Enter new username: ")
new_password = input("Enter new password: ")
new_balance = float(input("Enter initial balance: "))
usernames.append(new_username)
passwords.append(new_password)
balances.append(new_balance)
print(f"New user {new_username} added with balance: ${new_balance:.2f}")
def main():
global usernames # Declare usernames as a global variable
usernames, passwords, balances = read_user_information()
while True:
user_index = login(usernames, passwords)
if user_index is not None:
while True:
print("\nMenu:")
print("Type D to deposit money")
print("Type W to withdraw money")
print("Type B to display balance")
print("Type C to change user, display username")
print("Type A to add new client")
print("Type E to exit")
option = input("Enter your choice: ")
if option == 'D':
deposit(balances, user_index)
show_balance(balances, user_index)
elif option == 'W':
show_balance(balances, user_index)
withdraw(balances, user_index)
show_balance(balances, user_index)
elif option == 'B':
show_balance(balances, user_index)
elif option == 'C':
user_index = login(usernames, passwords)
if user_index is not None:
print(f"Switched to user: {usernames[user_index]}")
elif option == 'A':
add_new_user(usernames, passwords, balances)
elif option == 'E':
write_user_information(usernames, passwords, balances)
print("Exiting program. User information updated.")
return
else:
print("Invalid option. Please try again.")
if __name__ == "__main__":
main()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
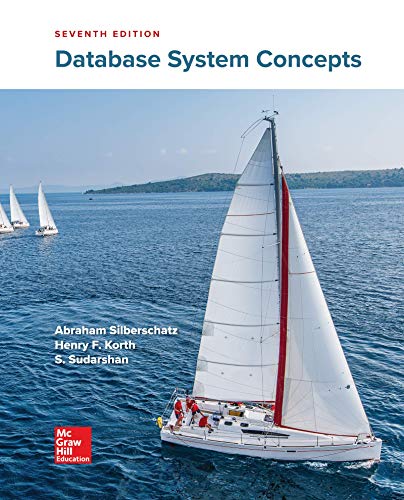
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
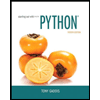
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
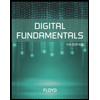
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
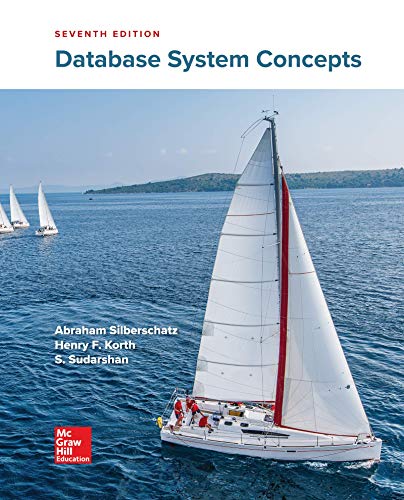
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
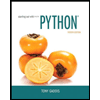
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
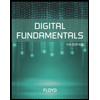
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
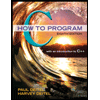
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
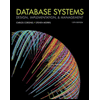
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
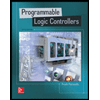
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education