mport java.util.Random; public class TestLength { public static void main(String[] args) { Random rand = new Random(10); Length length[] = new Length[10]; // fill length array and print for (int i = 0; i < length.length; i++) { length[i] = new Length(rand.nextInt(11) + 10, rand.nextInt(12)); System.out.print(length[i].toString() + " "); } System.out.println(); System.out.println(); // Print sum of the Length objects Length sum = sum(length); System.out.println("Sum is: "+sum); System.out.println(); // use sort method and print System.out.println("Sorted List"); selectionSort(length); for (int i = 0; i < length.length; i++) { System.out.print(length[i].toString() + " "); } System.out.println(); } public static void selectionSort(Length[] arr){ // Your code goes here } public static Length sum(Length[] list) { // Your code goes here } } _____________________________________________________ public class Length { private int feet; private int inches; // Your code goes here }
import java.util.Random;
public class TestLength {
public static void main(String[] args) {
Random rand = new Random(10);
Length length[] = new Length[10];
// fill length array and print
for (int i = 0; i < length.length; i++) {
length[i] = new Length(rand.nextInt(11) + 10, rand.nextInt(12));
System.out.print(length[i].toString() + " ");
}
System.out.println();
System.out.println();
// Print sum of the Length objects
Length sum = sum(length);
System.out.println("Sum is: "+sum);
System.out.println();
// use sort method and print
System.out.println("Sorted List");
selectionSort(length);
for (int i = 0; i < length.length; i++) {
System.out.print(length[i].toString() + " ");
}
System.out.println();
}
public static void selectionSort(Length[] arr){
// Your code goes here
}
public static Length sum(Length[] list) {
// Your code goes here
}
}
_____________________________________________________
public class Length
{
private int feet;
private int inches;
// Your code goes here
}
![STAGE 1 | The length of an object can be described by two integers: feet and inches (where 12
inches equal one foot). Class UML is as follows:
- feet: int
- inches : int
+ Length()
+ Length(newFeet: int, newinches: int)
+ getFeet(): int
+ setFeet(newFeet: int) : void
+ getinches(): int
+ setinches(newInches : int) : void
+ add(otherLength: Length): Length
+ subtract(otherLength: Length): Length
+ equals(otherLength: Length): boolean
+ compare To(otherLength: Length): int
+ toString(): String
Length
• Default constructor sets the length of an object to 0 feet & 0 inches
• Overloaded constructor sets the length of an object to given feet & inches (inches must
be in rage)
For each data field, define the accessor and mutator methods
•
add method, adds two length objects and return the result as a length object (feet &
inches must be in range)
• Subtract method, subtracts two length objects and return the result as a length object
(feet & inches must be in range. this object must be larger than otherLength)
• toString() method returns a String in format of [ ## ##"]
For example:
Length meLength = new Length(10, 6);
System.out.println(myLength.toString());
Will display: 10'6"
STAGE 2 | Implement the Length class using the above UML.
STAGE 3 | Compile "Length.java" and fix syntax errors
STAGE 4 | Driver program | Download "TestLength.java' from ZyBooks
1) implement the sum method to calculate sum of all the Length objects.
order
2) Implement the selectionSort to sort all Length objects in ascending or](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe4e826cb-c133-47e8-aeaa-858e7b3a09da%2Fc5054a1a-b7b9-4fa5-aa03-d58e23c9b32c%2Fqdupqh_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

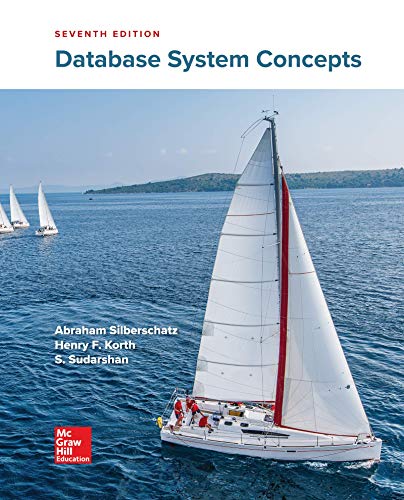
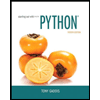
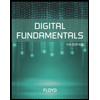
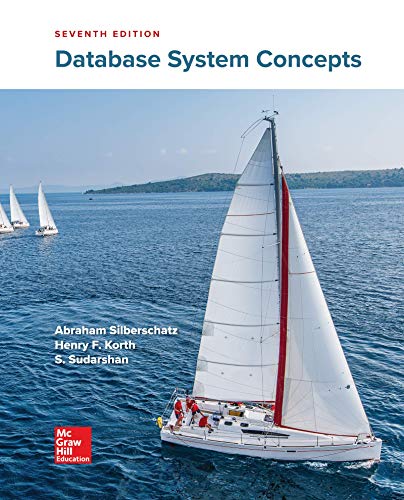
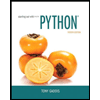
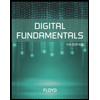
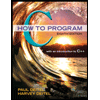
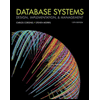
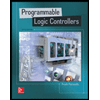