Modify the following program to read dictionary items from a file and write the inverted dictionary to a file: characters = {'Bugs':['Rabbit', 1940, 'boy'], 'Garfield': ['Cat', 1978, 'boy'], 'Tweety': ['Bird', 1942, 'girl'], 'Sandy': ['Squirrel', 1999, 'female']} print("Printing the original dictionary") print(characters) print("") def invert_dict(characters): inverse = dict() for key in characters: val = characters[key] for list_item in val: if list_item not in inverse: inverse[list_item] = [key] else: inverse[list_item].append(key) return inverse my_invert = invert_dict(characters) print("Printing the inverted dictionary") print(my_invert) Include the following: 1) The input file for your original dictionary (with at least six items). 2) The Python program to read from a file, invert the dictionary, and write to a different file. 3) The output file for your inverted dictionary.
Modify the following program to read dictionary items from a file and write the inverted dictionary to a file:
characters = {'Bugs':['Rabbit', 1940, 'boy'], 'Garfield': ['Cat', 1978, 'boy'], 'Tweety': ['Bird', 1942, 'girl'], 'Sandy': ['Squirrel', 1999, 'female']}
print("Printing the original dictionary")
print(characters)
print("")
def invert_dict(characters):
inverse = dict()
for key in characters:
val = characters[key]
for list_item in val:
if list_item not in inverse:
inverse[list_item] = [key]
else:
inverse[list_item].append(key)
return inverse
my_invert = invert_dict(characters)
print("Printing the inverted dictionary")
print(my_invert)
Include the following:
1) The input file for your original dictionary (with at least six items).
2) The Python program to read from a file, invert the dictionary, and write to a different file.
3) The output file for your inverted dictionary.
The answer must be explained technically.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

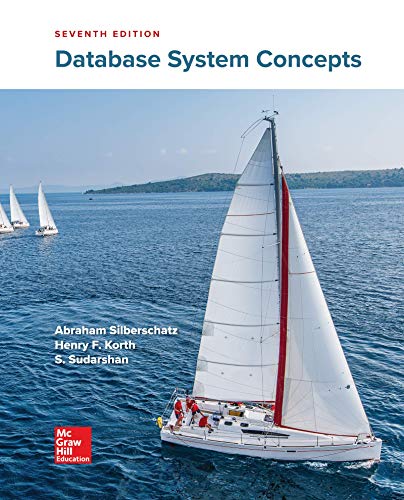
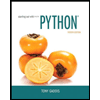
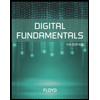
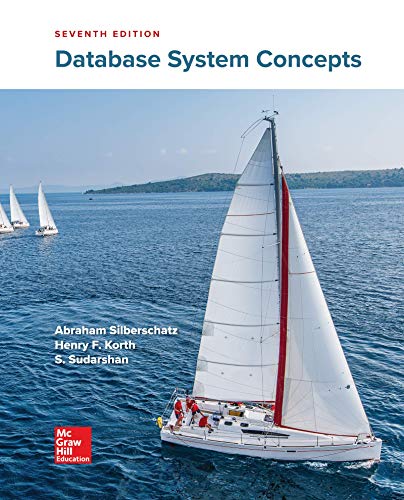
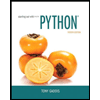
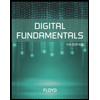
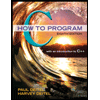
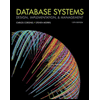
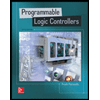